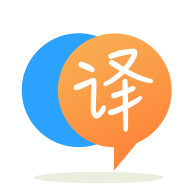
[英]How can i open different form pages in C# by login page while collecting data from database
[英]C# How to get data from the same form open several times and in each form there are different data?
我在 MDI 容器中有 Form1,当我提交名称和年龄时,Form2 在同一个容器内打开,名称和年龄写在 2 个标签上,我可以根据需要多次执行此操作。
我的问题是当我单击 Form1 中的保存按钮时,如何从 3 Form2 中获取数据并将其写入 a.txt 文件。
我看到了结合 Form2 字段名称和年龄并存储打开的 Form2 的愚蠢解决方案。 也许是这样:
// Example of Form1
public class Form1 : Form
{
// In this collection we will store all Form2 which would be opened
List<Form2> openedForms2 = new List<Form2>();
private void ButtonOpenForm2_Click(object sender, EventArgs e)
{
string name = nicknameBox.Text;
string age = ageBox.Text;
// Open Form2 with some values and store it in a List
Form2 form2 = new Form2(name, age);
// Subscribe to FormClosed event to be able to remove it from List of opened forms after closing
form2.FormClosed += (sender, args) => { openedForms2.Remove(form2); };
form2.Show();
openedForms2.Add(form2);
}
private void ButtonSave_Click(object sender, EventArgs e)
{
StringBuilder sb = new StringBuilder(); // Reference to System.Text should be provided
// Here you getting opened forms, which are stored in List
foreach (Form2 form2 in openedForms2)
{
// And you able to get values of fields Name and Age of each opened form
string name = form2.Name;
string age = form2.Age;
// Combine them in a way you wish
sb.AppendLine("Name: " + name + " | Age: " + age);
}
// Finally save all combined lines in StringBuilder to some file
// Don't forget about System.IO reference
File.WriteAllText("PathToYourFile", sb.ToString());
}
}
// Example of Form2
public class Form2 : Form
{
// This fields will be available for access from Form1 after Form2 opened
private string Name = string.Empty;
private string Age = string.Empty;
public Form2(string name, string age)
{
// Set fields values
Name = name;
Age = age;
// Set labels text
form2Name.Text = name;
form2Age.Text = age;
}
}
我认为您要做的是Winform
将 Winform 的文本框值作为 a.txt 文件?
如果是这样,您可以使用System.IO
创建TextWriter
的实例。 这将采用给定的字符串输入并将其写入您选择的destination.txt 文件,如果该文件尚不存在则创建该文件。
确保在您的 class 顶部有:
using System.IO;
您必须将您的方法放在try/catch
块中,因为它在写入时可能会遇到很多错误。 这篇文章告诉你关于获取文本框值
static void WriteOutput()
{
//A using statement uses the object once, then disposes of it's resources after the block
using (StreamWriter writer = File.CreateText("YOURPATH/YOURFILE.txt"))
{
writer.WriteLine(this.textbox1.Text + "\n");
writer.WriteLine(this.textbox2.Text);
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.