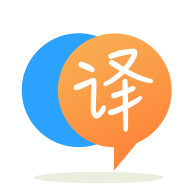
[英]How do i display & style JSON data in my HTML (via node JS/Express) page
[英]How do i fetch json from a url and display json content on my js page
我正在制作一个自定义的 MS Teams 应用程序,并在该应用程序中尝试从 url 获取 json 以便稍后显示内容。 但是,获取失败。 我的目标是从提供的 url 中获取数据列表,然后将其显示在我的团队应用程序中的选项卡中,这将在此处:我希望我的 json 内容显示在哪里
正如您可能知道的那样,我根本没有任何使用 javascript 的经验,但是自定义 MS 团队应用程序想要 javascript...
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License.
import React from 'react';
import './App.css';
import * as microsoftTeams from "@microsoft/teams-js";
/**
* The 'GroupTab' component renders the main tab content
* of your app.
*/
class Tab extends React.Component {
constructor(props){
super(props)
this.state = {
context: {}
}
}
//React lifecycle method that gets called once a component has finished mounting
//Learn more: https://reactjs.org/docs/react-component.html#componentdidmount
componentDidMount(){
// Get the user context from Teams and set it in the state
microsoftTeams.getContext((context, error) => {
this.setState({
context: context
});
});
// Next steps: Error handling using the error object
}
componentDidMount() {
fetch('http://example.com/movies.json')
.then(response => response.json())
.then(data => console.log(data));
}
render() {
var jsondata = data;
let userName = Object.keys(this.state.context).length > 0 ? this.state.context['upn'] : "";
return (
<div>
</div>
);
}
}
export default Tab;
总而言之,我如何从 url 获取我的 json 并在团队内的 tab.js 页面上显示 json 的内容?
提前感谢您的帮助。
虽然我无法说明 Teams API 的工作原理,但我可以帮助您了解如何在您的 react 组件中从 json API 渲染事物。
在您的componentDidMount
function 中,您的示例正在发送和接收来自 API 的响应。 要呈现此响应,我们需要将data
分配给组件的“状态”,然后使用它在 HTML 中呈现它。
这将非常简单。 首先,您需要扩展组件的 state,方式与您对context
所做的类似。 首先在构造函数中执行此操作,我们将在其中声明一个空 object 的初始 state(我将其命名为content
,但您可以使用最有意义的任何名称):
// inside the constructor() function
this.state = {
context: {},
content: {}
}
在 React 中,我们使用setState
来更新此 state object state ,例如在诸如componentDidMount
之类的生命周期方法上。 当您想将 state object 从其初始值更改为其他值时,您只需再次调用此setState
。 在我们的例子中,当我们收到来自 API 的数据时。
setState
获取您提供的任何内容并将其合并到 state object 中,因此您只需声明您想要更改的任何内容。 其他未声明的内容将保持不变。
因此,在componentDidMount
中,我们可以做一个小改动,以便在data
到达时对其进行处理:
componentDidMount() {
fetch('http://example.com/movies.json')
.then(response => response.json())
.then(data => {
this.setState({
content: data
})
});
}
这基本上是在说:
content
。 然后,您可以通过调用this.state.content
来处理这些数据。 I'm not sure what format the data will come in, but whatever json object arrives back from the API will be stored under this.state.content
.
例如,假设我们从 API 返回一个简单的 object,它看起来像这样{ title: "Tab title" }
。 这意味着,在成功调用 API 后,我们的 state object 将如下所示:
{
context: "whatever you have here", // whatever you have here, I don't know this
content: { title: "Tab title" }
}
当此 state object 更新时,react 将触发组件的新渲染。
所以,为了让它出现在我们的组件中,我们需要在我们的render
function 中使用这个 state(如果需要动态渲染而不是硬编码,我们将它们包裹在花括号中):
render() {
return (
//... the rest of your function
<div>{this.state.content.title}</div>
);
}
正如您可能已经猜到的那样,如果标题存在,这将在 div 中显示标题。
最后,您应该考虑在 API 调用自行解决之前处理组件的 state。 生命周期方法componentDidMount
将在组件安装后被调用,并且因为您遇到的是 API,所以在 API 调用自行解决之前,将有一些东西呈现给 DOM。 在我的示例中,它只是一个空 div,然后它会在 state 更新并且再次调用渲染 function 时出现。
您可以通过扩展您的 state object 来更有效地做到这一点,以查看 API 响应是否完成(您可以在设置内容的同一位置更新它),并且您可以有条件地在渲染此内容时进行更新。
生命周期方法的官方文档将帮助您更多地理解这种模式。
祝你好运!
好吧,你可以依靠@Josh Vince 来解释,因为他做得很完美,我会为你编写更简洁的代码。
让我们创建 2 个方法,用相应的数据设置 state,然后简单地调用 state 值并根据您的意愿呈现以下内容。
import React, {Component} from 'react';
import './App.css';
import * as microsoftTeams from "@microsoft/teams-js";
class Tab extends Component {
this.state = {
context: {},
movies: null
}
getContext = () => {
try {
microsoftTeams.getContext((context) => this.setState({context}));
}
catch(error){
console.log('ERROR ->', error);
throw error;
}
}
fetchMoviesData = () => {
try {
fetch('http://example.com/movies.json')
.then(response => response.json())
.then(data => this.setState({movies: data}));
} catch(error){
console.log('ERROR ->', error);
throw error;
}
}
componentDidMount() {
this.getContext();
this.fetchMoviesData();
}
render() {
let {movies, context} = this.state;
const jsondata = movies;
let userName = Object.keys(this.state.context).length ? context['upn'] : "";
return <>JSONDATA: {jsondata}, USERNAME: {userName}</>
}
}
export default Tab;
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.