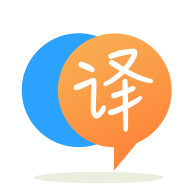
[英]If we cannot create an instance of an interface in Java and arrays are nothing but Objects. Then, How is it possible to create Array of interface?
[英]How can we create an instance for a nested class in array of objects in java?
import java.util.Arrays;
import java.util.Scanner;
public class employee{
public String name;
public class employee_address{
String street_name;
String city;
String zipcode;
String state;
String country;
}
public static void main(String []args){
Scanner user_input = new Scanner(System.in);
int no_of_employees = user_input.nextInt();
employee[] employees_list = new employee[no_of_employees];
for(int i = 0;i < no_of_employees;i++){
employees_list[i].name = user_input.nextLine();
employees_list[I].employee_address = // this is it ?
}
}
}
在上面的代码中,我确实理解employee_address 是一个类,如果没有像代码中那样创建实例,就不能直接访问它,这是没有意义的。 但是如何创建与每个员工关联的 employee_address 类的实例。
就像上面的代码中的“employee_address”与每个员工相关联,但是如何初始化“employee_address”类以及如何设置地址类中的街道名称、城市和其他成员。 任何想法,将不胜感激。
下面的代码使用Java 命名约定(您的代码没有)。
代码后的注释。
import java.util.Scanner;
public class Employee {
private String name;
private EmployeeAddress address;
public class EmployeeAddress {
String streetName;
String city;
String zipcode;
String state;
String country;
}
public static void main(String[] args) {
Scanner userInput = new Scanner(System.in);
int noOfEmployees = userInput.nextInt();
Employee[] employeesList = new Employee[noOfEmployees];
for (int i = 0; i < noOfEmployees; i++) {
employeesList[i] = new Employee();
employeesList[i].name = userInput.nextLine();
EmployeeAddress employeeAddress = employeesList[i].new EmployeeAddress();
employeesList[i].address = employeeAddress;
employeesList[i].address.streetName = userInput.nextLine();
}
}
}
内部类是一个普通类。 它不是其封闭类的成员。 如果您希望Employee
类具有 [employee] 地址以及 [employee] 名称,则需要向Employee
类添加另一个成员变量,其类型为EmployeeAdress
。
Employee[] employeesList = new Employee[noOfEmployees];
上面的行创建了一个数组,但数组中的每个元素都为空。 因此,您需要首先创建一个Employee
对象并将其分配给数组的一个元素。 因此,我上面的代码中有以下行:
employeesList[i] = new Employee();
由于EmployeeAddress
不是静态类,为了创建一个新实例,您首先需要一个封闭类的实例,即Employee
。 因此,上面代码中的以下行。
EmployeeAddress employeeAddress = employeesList[i].new EmployeeAddress();
由于您的所有代码都在Employee
类中,因此在main
方法中您可以直接访问Employee
类和EmployeeAddress
类的成员。 尽管如此,您需要了解 java 中的不同访问修饰符。
不能直接创建内部类的实例,因为它是另一个实例的属性,我们总是需要通过父变量的实例来使用它。
假设你有一个类,它有两个属性:
public class Employee {
public String name;
public EmployeeAddress emAddress;
}
要访问您需要通过Employee
类的实例使用的emAddress
,例如 -
Employee object = new Employee();
EmployeeAddress empAdd = object.new EmployeeAddress();
完整代码:
public class Employee {
public String name;
public EmployeeAddress emAddress;
public class EmployeeAddress {
String street_name;
String city;
String zipcode;
String state;
String country;
public String getStreet_name() {
return street_name;
}
public void setStreet_name(String street_name) {
this.street_name = street_name;
}
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
public String getZipcode() {
return zipcode;
}
public void setZipcode(String zipcode) {
this.zipcode = zipcode;
}
public String getState() {
return state;
}
public void setState(String state) {
this.state = state;
}
public String getCountry() {
return country;
}
public void setCountry(String country) {
this.country = country;
}
@Override
public String toString() {
return "EmployeeAddress [street_name=" + street_name + ", city=" + city + ", zipcode=" + zipcode
+ ", state=" + state + ", country=" + country + "]";
}
}
public static void main(String[] args) {
Scanner user_input = new Scanner(System.in);
int no_of_employees = user_input.nextInt(); // let's say no_of_employees = 1
Employee[] employees = new Employee[no_of_employees];
for (int i = 0; i < no_of_employees; i++) {
Employee object = new Employee();
object.setName("Virat Kohli");
EmployeeAddress empAdd = object.new EmployeeAddress();
empAdd.setCity("New Delhi");
empAdd.setCountry("India");
empAdd.setState("Delhi");
empAdd.setStreet_name("Chandni Chalk");
empAdd.setZipcode("741124");
object.setEmAddress(emAddress);
employees[i] = object;
}
System.out.println(employees[0]);
user_input.close();
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public EmployeeAddress getEmAddress() {
return emAddress;
}
@Override
public String toString() {
return "Employee [name=" + name + ", emAddress=" + emAddress + "]";
}
public void setEmAddress(EmployeeAddress emAddress) {
this.emAddress = emAddress;
}
}
我已将您的代码修改为sonar
标准。
一些提示:
ArrayList
等EmployeeAddress
,它应该在方便时初始化它。 所以一个对象总是对它自己的东西负责scanner.nextInt()
可能会出现换行符/换行符的问题。 对于用户输入更好的readLine()
和解析输入代码:
import java.io.PrintStream;
import java.util.ArrayList;
import java.util.Scanner;
public class Employee {
static public class EmployeeAddress {
public final String street_name;
public final String city;
public final String zipcode;
public final String state;
public final String country;
public EmployeeAddress(final Scanner pScanner, final PrintStream pOutPS) {
street_name = readLine(pScanner, pOutPS, "Please enter Street Name:");
city = readLine(pScanner, pOutPS, "Please enter City Name:");
zipcode = readLine(pScanner, pOutPS, "Please enter Zip Code:");
state = readLine(pScanner, pOutPS, "Please enter State:");
country = readLine(pScanner, pOutPS, "Please enter Country:");
}
}
static public String readLine(final Scanner pScanner, final PrintStream pOutPS, final String pPrompt) {
pOutPS.print(pPrompt);
final String value = pScanner.nextLine();
pOutPS.println();
return value;
}
static public int readInt(final Scanner pScanner, final PrintStream pOutPS, final String pPrompt) {
return Integer.parseInt(readLine(pScanner, pOutPS, pPrompt));
}
public final String name;
public final EmployeeAddress address;
public Employee(final Scanner pScanner, final PrintStream pOutPS) {
name = readLine(pScanner, pOutPS, "Please enter Employee Name: ");
System.out.println("Name: " + name);
address = new EmployeeAddress(pScanner, pOutPS);
}
public static void main(final String[] args) {
try (final Scanner user_input = new Scanner(System.in);
final PrintStream output = System.out;) {
final int no_of_employees = readInt(user_input, output, "Please enter number of users: ");
final Employee[] employees_list = new Employee[no_of_employees]; // either this line
final ArrayList<Employee> employees = new ArrayList<>(); // or this line
for (int i = 0; i < no_of_employees; i++) {
output.println("Creating user #" + (i + 1) + "...");
final Employee newEmployeeWithAddress = new Employee(user_input, output);
employees_list[i] = newEmployeeWithAddress; // either this line
employees.add(newEmployeeWithAddress); // or this line
}
}
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.