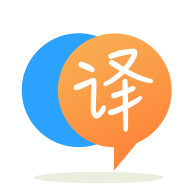
[英]How to merge lists of Map with Lists values using Java Streams API?
[英]How to merge two lists after comparing using java streams API
我有两个相同类型的列表:Boxes1 和 Boxes2:
"boxes1": [{
"boxId": "ABC",
"ele": [{
"eleId": "8040",
"chars": [{
"no": "123",
"qty": 2
}
]
}
]
}, {
"boxId": "XYZ",
"ele": [{
"eleId": "1212",
"chars": [{
"no": "456",
"qty": 3
}
]
}
]
}
]
和
"boxes2": [{
"boxId": "ABC",
"ele": [{
"eleId": "8040",
"chars": [{
"no": "123",
"qty": 6
}
]
}, {
"eleId": "4560",
"chars": [{
"no": "012",
"qty": 3
}
]
}
]
}, {
"boxId": "PQR",
"ele": [{
"eleId": "1111",
"chars": [{
"no": "456",
"qty": 8
}
]
}
]
}
]
我想检查 boxes1 中的任何boxId是否与boxes2中的boxId匹配,然后我想将box1的公共元素eleId的字符添加到box2中,以便合并后的结果如下所示:
"box2": [{
"boxId": "ABC",
"ele": [{
"eleId": "8040",
"chars": [{
"no": "123",
"qty": 6
},
{
"no": "123",
"qty": 2
}
]
},.....................
而且,如果Boxes1中有任何boxId不存在于Boxes2中,我想将该框添加到Boxes2中。 所以最后它应该是这样的:
"box2": [{
"boxId": "ABC",
"ele": [{
"eleId": "8040",
"chars": [{
"no": "123",
"qty": 6
}, {
"no": "123",
"qty": 2
}
]
}, {
"eleId": "4560",
"chars": [{
"no": "012",
"qty": 3
}
]
}
]
}, {
"boxId": "PQR",
"ele": [{
"eleId": "1111",
"chars": [{
"no": "456",
"qty": 8
}
]
}
]
}, {
"boxId": "XYZ",
"ele": [{
"eleId": "1212",
"chars": [{
"no": "456",
"qty": 3
}
]
}
]
}
]
我是 Stream 的新手,所以尝试使用旧方法但它有问题:
boxes2.forEach(box2 -> {
boxes1.forEach(box1 -> {
if (box1.getBoxId().equals(box2.getBoxId())) {
box2.getEle().forEach(ele1 -> {
box1.getEle().forEach(ele2 -> {
if (ele1.getEleId().equals(ele2.getEleId())) {
ele1.chars().addAll(ele2.chars());
}
});
});
} else {
boxes2.add(box1);
}
});
});
使用Stream
对您的情况没有多大帮助。 我建议 go 用于 POIC(普通的旧命令式代码)。
伪代码算法:
for ( box1 of boxes1 ) {
var box1Id = box.boxId;
if ( boxes2.containsBoxId(box1Id) ) {
var box2 = boxes2.getBoxById(boxId);
for ( ele1 of box1.ele ) {
var ele1Id = ele1.eleId;
if ( box2.containsEleId(ele1Id) ) {
var ele2 = box2.getEleById(ele1Id);
ele2.addToChars(ele1.chars);
}
}
} else {
// if boxes2 doesn't contain box1Id
boxes2.addBox(box1);
}
}
对于Stream
s,我唯一要考虑的是“查找匹配的元素并添加字符”部分。 但是对于巨大的 collections 来说,这会变慢。
var box1Id = ...
var box1ele = ...
boxes2.stream()
.filter(b -> b.boxId.equals(box1Id))
.flatMap(b -> b.ele.stream())
.filter(e -> e.eleId.equals(box1ele))
.forEach(e -> e.addChars(box1ele.chars)
您当然也可以使用Stream
进行 box1 迭代。 但这将导致一些非常丑陋和不可读的代码。 (编辑:就像你的示例代码一样:不好。)
您可以改为:将相关数据放入Map
中,以便在没有所有这些循环的情况下更快、更轻松地访问。
另一个解决方案是这样的:
List<Box> boxResult = Stream.concat(boxes1.stream(), boxes2.stream())
.collect(toMap(Box::getBoxId,
v-> new ArrayList<>(v.getEle()),
YOUR_CLASS::mergeEle))
.entrySet().stream()
.map(entry -> new Box(entry.getKey(), entry.getValue()))
.collect(Collectors.toList());
和mergeEle
方法:
private static List<Ele> mergeEle(List<Ele> l1, List<Ele> l2) {
return Stream.concat(l1.stream(), l2.stream())
.collect(toMap(Ele::getEleId,
v -> new ArrayList<>(v.getChars()),
YOUR_CLASS::mergeChar))
.entrySet().stream()
.map(e -> new Ele(e.getKey(), e.getValue()))
.collect(Collectors.toList());
}
和mergeChar
:
private List<Char> mergeChar(List<Char> c1, List<Char> c2) {
c1.addAll(c2);
return c1;
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.