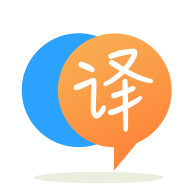
[英]Wrapping a C++ class method in a try catch block with added functionality
[英]try catch block in c++ class ussage
这是导致错误的 v1
#include <iostream>
#include "exceptionHandlingV2.h"
using namespace std;
int main() {
exceptionHandlingV2 ob(4,0);
cout<<ob.divide();
cout<<"\nbye";
return 0;
}
#ifndef EXCEPTION_HANDLING_V2_EXCEPTIONHANDLINGV2_H
#define EXCEPTION_HANDLING_V2_EXCEPTIONHANDLINGV2_H
#include <iostream>
using namespace std;
class exceptionHandlingV2 {
private:
int numerator;
int denominator;
string error;
public:
exceptionHandlingV2();
exceptionHandlingV2(int ,int);
void setDenominator(int );
void setNumerator(int );
void readAgain ();
void print_errors();
double divide() const;
};
#endif //EXCEPTION_HANDLING_V2_EXCEPTIONHANDLINGV2_H
#include "exceptionHandlingV2.h"
exceptionHandlingV2::exceptionHandlingV2(){
numerator=1;
denominator=1;
}
exceptionHandlingV2::exceptionHandlingV2(int numerator,int denominator){
setNumerator(numerator);
try {
setDenominator(denominator);
error = "";
}catch (const char * e){
error = e;
print_errors();
readAgain();
}
}
void exceptionHandlingV2::setDenominator(int denominator){
if ( denominator == 0 ){
throw "divided by zero\n";
}
(*this).denominator = denominator;
}
void exceptionHandlingV2::readAgain (){
int temp;
cout<<"enter denominator again other than 0\n";
cin >> temp;
exceptionHandlingV2 ( numerator,temp );
}
void exceptionHandlingV2::print_errors(){
cout<<error;
}
void exceptionHandlingV2::setNumerator(int numerator){
(*this).numerator = numerator;
}
double exceptionHandlingV2::divide() const{
return (double)numerator/denominator;
}
这是 v2 设计,我停止在构造函数中与用户交互
exceptionHandlingV2::exceptionHandlingV2(int numerator,int denominator){
setNumerator(numerator);
setDenominator( denominator );
}
void exceptionHandlingV2::setDenominator(int denominator){
try
{
if ( denominator == 0 )
throw "divided by zero\n";
(*this).denominator = denominator;
error = "";
}
catch (const char * e)
{
error = e;
print_errors();
readAgain();
}
}
在 v3 设计中,我添加了一个新函数来处理类本身内部的异常
exceptionHandlingV4::exceptionHandlingV4(int numerator,int denominator){
setNumerator(numerator);
setDenominator( denominator );
}
void exceptionHandlingV4::setDenominator(int denominator){
try {
runtime_set_denominator_error ( denominator );
}
catch ( const char *e )
{
error = e;
print_errors();
readAgain();
}
}
void exceptionHandlingV4 :: runtime_set_denominator_error ( int denominator ){
if ( !denominator )
throw "divided by zero \n";
(*this).denominator = denominator;
error = "";
}
我做了一个 v4 设计来停止在 class 中处理异常,而不是在 main() 中处理。 哪一个是正确的? 在类中处理异常或只是从类中抛出异常并在 main 中处理?,问题是我不能再继续从用户读取 readAgain() 直到用户输入写入输入,因为异常将进入 catch 块并且无法处理catch 块中的运行时错误,所以我从设计中删除了 readagain() 函数,只在 main() 部分打印了错误
exceptionHandlingV5::exceptionHandlingV5(int numerator,int denominator){
setNumerator(numerator);
setDenominator( denominator );
}
void exceptionHandlingV5::setDenominator(int denominator){
if ( !denominator ){
error = "divided by zero \n";
throw "divided by zero\n";
}
(*this).denominator = denominator;
error = "";
}
//and also I had to move obj creation
//out of try block, and therefore
//because of set_denominator(); handles exception
// I had to forget initializing when creating obj
//and instead used set methods
int main() {
exceptionHandlingV5 ob;
ob.setNumerator(4);
try {
ob.setDenominator(0);
// logic
cout << ob.divide();
}
catch(char const *e)
{
// I can only print errors now
// can't keep reading
ob.print_errors();
}
}
感谢 @sonulohani、@RichardCritten 和 @molbdnilo 指导我并修改我的愚蠢问题,我的意思是现在我看了我的第一个设计,我发现我犯了很多错误和错误,我通过你的帮助和评论解决了. 无论如何,我最终修改了我的整个设计,并为 Division 创建了一个新类和 main() 函数。 我首先想做的就是从类中练习运行时错误处理,现在我想我终于明白了。 这是最后的设计。 它可能会在未来帮助某人。
#ifndef EXCEPTION_HANDLING_FINAL_V1_EXCEPTION_HANDLING_FINAL_V1_H
#define EXCEPTION_HANDLING_FINAL_V1_EXCEPTION_HANDLING_FINAL_V1_H
class exception_handling_final_v1 {
private:
double numerator;
double denominator;
char * error;
public:
exception_handling_final_v1();
exception_handling_final_v1( double , double );
void set_numerator ( double );
void set_denominator ( double );
double divide ( );
char * errors();
};
#endif //EXCEPTION_HANDLING_FINAL_V1_EXCEPTION_HANDLING_FINAL_V1_H
#include "exception_handling_final_v1.h"
exception_handling_final_v1::exception_handling_final_v1() {
numerator = 1;
denominator = 1;
error = "";
}
exception_handling_final_v1::exception_handling_final_v1( double numerator,
double denominator) {
set_numerator( numerator );
set_denominator ( denominator );
}
void exception_handling_final_v1::set_numerator ( double numerator ){
(*this).numerator = numerator;
}
void exception_handling_final_v1::set_denominator( double denominator ) {
if ( !denominator ){
error = "denominator can not be zero\n";
throw "denominator can not be zero\n";
}
(*this).denominator = denominator;
error = "";
}
double exception_handling_final_v1 :: divide () {
return denominator / numerator;
}
char * exception_handling_final_v1::errors() {
return error;
}
#include <iostream>
#include "exception_handling_final_v1.h"
using namespace std;
int main() {
exception_handling_final_v1 ob;
double numerator , denominator , result;
cout << "enter nummerator\n";
cin >> numerator;
ob.set_numerator( numerator );
cout << "enter denominator\n";
cin >> denominator ;
try{
ob.set_denominator( denominator );
result = ob.divide();
}
catch( char const * error){
cout << ob.errors();
}
cout<<"bye";
return 0;
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.