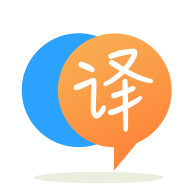
[英]React Native: How to get data from JSON with different keys and display it?
[英]How to display data from json in React
我尝试创建生成随机报价的简单应用程序。 我创建了一个函数,(我认为)从 json 文件中获取我想要的数据。 但是当我尝试将该函数传递给我的 App 函数时,我得到错误:对象作为 React 子对象无效(发现:[object Promise])
功能报价:
function Quote (data) {
var x = (Math.floor(Math.random() * (103 - 1) + 1) );
return fetch('https://gist.githubusercontent.com/camperbot/5a022b72e96c4c9585c32bf6a75f62d9/raw/e3c6895ce42069f0ee7e991229064f167fe8ccdc/quotes.json')
.then((response) => response.json())
.then((responseJson) => {
console.log(responseJson['quotes'][0]['author']);
return responseJson['quotes'][x]['author'];
})
.catch((error) => {
console.error(error);
});
}
应用功能:
function App() {
var text = '';
return (
<div id="quote-box">
<div id="author"><Quote /></div>
<button id="new-quote">New Quote</button>
<a href="twitter.com" id="tweet-quote">Tweet</a>
</div>
);
}
我会使用 useEffect 在开始时触发调用。 并使用 useState 来保存值。 然后还将相同的逻辑添加到 onClick。
import { useEffect, useState } from "react";
function getQuote() {
var x = Math.floor(Math.random() * (103 - 1) + 1);
return fetch(
"https://gist.githubusercontent.com/camperbot/5a022b72e96c4c9585c32bf6a75f62d9/raw/e3c6895ce42069f0ee7e991229064f167fe8ccdc/quotes.json"
)
.then((response) => response.json())
.then((responseJson) => {
console.log(responseJson["quotes"][0]["author"]);
return responseJson["quotes"][x]["author"];
})
.catch((error) => {
console.error(error);
});
}
export default function App() {
const [author, setAuthor] = useState("");
useEffect(() => {
getQuote().then((newAuthor) => setAuthor(newAuthor));
}, []);
return (
<div id="quote-box">
<div id="author">{author}</div>
<button
id="new-quote"
onClick={() => getQuote().then((newAuthor) => setAuthor(newAuthor))}
>
New Quote
</button>
<a href="twitter.com" id="tweet-quote">
Tweet
</a>
</div>
)
}
您也可以通过这种方式存档。 创建自定义挂钩并使用它。
import React from "react";
function useFetchQuote(newQuote) {
const [author, setAuthor] = React.useState();
React.useEffect(() => {
var x = Math.floor(Math.random() * (20 - 1) + 1);
return fetch(
"https://gist.githubusercontent.com/camperbot/5a022b72e96c4c9585c32bf6a75f62d9/raw/e3c6895ce42069f0ee7e991229064f167fe8ccdc/quotes.json"
)
.then((response) => response.json())
.then((responseJson) => {
console.log(responseJson["quotes"][x]["author"]);
setAuthor(responseJson["quotes"][x]["author"]);
})
.catch((error) => {
console.error(error);
});
}, [newQuote]);
return { author };
}
function App() {
const [newQuote, setQuote] = React.useState(0);
const { author } = useFetchQuote(newQuote);
return (
<div id="quote-box">
<div id="author">{author}</div>
<button id="new-quote" onClick={() => setQuote(newQuote + 1)}>
New Quote
</button>
<a href="twitter.com" id="tweet-quote">
Tweet
</a>
</div>
);
}
export default App;
在React
组件中返回 promise 不会像您在代码中所做的那样工作。 React
组件必须返回一个jsx
。 例如在名为Quote.js
的文件中
import * as React from 'react';
const url =
'https://gist.githubusercontent.com/camperbot/5a022b72e96c4c9585c32bf6a75f62d9/raw/e3c6895ce42069f0ee7e991229064f167fe8ccdc/quotes.json';
const Qoute = () => {
const [quote, setQuote] = React.useState(null);
React.useEffect(() => {
fetch(url)
.then((response) => response.json())
.then((data) => {
const randomNumber = 1; // Generate random number which is lesser or equal to data's length
setQuote(data['quotes'][randomNumber]);
});
}, []);
if (!quote) return <React.Fragment>Loading...</React.Fragment>;
return <div>{JSON.stringify(quote)}</div>;
};
export default Qoute;
然后你只需要将它导入到你想使用它的地方并像这样调用它
<Quote />
PS:如果某些东西不起作用,我会从typescript
转换它,我很乐意提供帮助。 并请记得更新这里我把注释行。 祝你好运,兄弟。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.