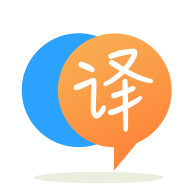
[英]How can I convert from a class with a 2D vector of chars to a 2D vector of another obj with a char variable?
[英]how do I handle an Array for a 2D Vector in an Object of another class?
我需要一点帮助来安排我的约会。 我的教授给了我们这个类(以及一个里面有 RGB 颜色作为浮点变量的 Color 类)现在我必须实现标题中显示的函数。
#include "color.h"
#include <assert.h>
Color::Color()
{
R = 255;
G = 255;
B = 255;
}
Color::Color( float r, float g, float b)
{
R = r;
G = g;
B = b;
}
Color Color::operator*(const Color& c) const
{
return Color(R * c.R, G * c.G, B * c.B );
}
Color Color::operator*(const float Factor) const
{
return Color(R * Factor, G * Factor, B * Factor);
}
Color Color::operator+(const Color& c) const
{
return Color(R + c.R, G + c.G, B + c.B);
}
Color& Color::operator+=(const Color& c)
{
R += c.R;
G += c.G;
B += c.B;
return *this;
}
标题 RGBImage
Konstruktor 应该创建一个 2DImage 内存来保存宽*高像素。 (不知道这里最好的解决方案是什么?颜色或向量类型的数组?)我的第一个猜测是:
RGBImage 类(我刚刚得到了空方法)
#include "rgbimage.h"
#include "color.h"
#include "assert.h"
using namespace std;
RGBImage::RGBImage( unsigned int Width, unsigned int Height)
{
m_Image = new Color[Width * Height]; // probably wrong?
m_Width = Width;
m_Height = Height;
}
RGBImage::~RGBImage()
{
}
void RGBImage::setPixelColor( unsigned int x, unsigned int y, const Color& c)
{
if (x < width() && y < height())
{
// get offset of pixel in 2D array.
const unsigned offset = (y * width()) + x;
m_Image[offset] = c;
}
}
const Color& RGBImage::getPixelColor( unsigned int x, unsigned int y) const
{
if (x < width() && y < height())
{
// get offset of pixel in 2D array.
const unsigned offset = (y * width()) + x;
return m_Image[offset];
}
}
unsigned int RGBImage::width() const
{
return this->m_Width;
}
unsigned int RGBImage::height() const
{
return this->m_Height;
}
unsigned char RGBImage::convertColorChannel( float v)
{
if (v < 0) {
v = 0;
}
else if (v > 1) {
v = 1;
}
int convertedColorChannel = v * 255;
return convertedColorChannel;
}
bool RGBImage::saveToDisk( const char* Filename)
{
// TODO: add your code
return false; // dummy (remove)
}
之后,我意识到颜色数组不是每个 Header 定义的 RGBImage 类变量,所以我如何将像素保存在 RGBImage 中,或者继续这种方法是一个可行的选择。 如果是这样,我如何在 setter 中设置颜色? 用这个试过了。bildspeicher[x] 没用...
我对编程还很陌生,这是我在这个平台上的第一个问题,如果我说得不好,我很抱歉。
RGB 数据通常存储在一维数组中,就像 RGBImage 的情况一样。 像素逐行打包,从图像的左下角或左上角开始。 方向不应影响访问单个像素行的函数,但会影响调用应用程序处理像素数据的方式。
要访问单个像素,请使用以下公式:
// ...
inline void setPixel(unsigned x, unsigned y, const Color& clr)
{
if (x < width() && y < height()) // ALWAYS crop!
{
// get offset of pixel in 2D array.
const unsigned offset = (y * width()) + x;
m_image[offset] = clr;
}
}
我已经将公式放在了它自己的代码行中,但这通常是作为一行代码完成的。
相同的公式可用于读取像素。 请注意,此公式假定像素线没有对齐。 某些位图 fprmat 确实需要 2d 数组中每行的 4 字节对齐方式中的 2 个,在这种情况下,您需要将 y 乘以对齐宽度()而不是宽度())。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.