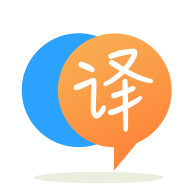
[英]How to call parent widget function from child widget in Flutter
[英]Flutter - how to call child widget's method from parent
假设我们有两个有状态的小部件。
ParentWidget(){
}
ChildWidget() {
someMethod(){
// some code, for example setState code
}
}
现在,当我在ParentWidget
使用ChildWidget
时,如何调用someMethod()
?
如果您需要在小部件上调用函数,您可以使用:
context.findAncestorWidgetOfExactType<T>()
如果您需要在该小部件的状态上调用函数,您可以使用:
context.findRootAncestorStateOfType<T>();
阅读更多信息:
https://api.flutter.dev/flutter/widgets/BuildContext/findAncestorWidgetOfExactType.html
https://api.flutter.dev/flutter/widgets/BuildContext/findRootAncestorStateOfType.html
这是我使用过的方式。
GlobalKey<ChildWidgetState> globalKey = GlobalKey();
ParentWidget(){
ChildWidget(key: globalKey);
...
globalKey.currentState.someMethod();
}
ChildWidget() {
ChildWidget({Key key}) : super(key: key);
someMethod(){
// some code, for example setState code
}
}
测试代码
import 'package:flutter/material.dart';
const Color darkBlue = Color.fromARGB(255, 18, 32, 47);
void main() {
runApp(MyApp());
}
GlobalKey<ChildWidgetState> globalKey = GlobalKey();
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
theme: ThemeData.dark().copyWith(
scaffoldBackgroundColor: darkBlue,
),
debugShowCheckedModeBanner: false,
home: Scaffold(
body: Center(
child: MyWidget(),
),
),
);
}
}
class MyWidget extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Column(
children: [
InkWell(
onTap: () {
globalKey.currentState.someMethod();
},
child: Text('ParentWidget'),
),
ChildWidget(key: globalKey),
],
);
}
}
class ChildWidget extends StatefulWidget {
ChildWidget({Key key}) : super(key: key);
@override
ChildWidgetState createState() => ChildWidgetState();
}
class ChildWidgetState extends State<ChildWidget> {
void someMethod() {
print('someMethod is called');
}
@override
Widget build(BuildContext context) {
return Text('childWidget');
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.