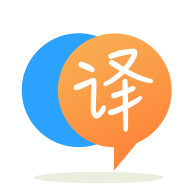
[英]How to write a find prefix and find word method for strings stored in a binary search tree?
[英]How do I use a binary search tree to find prefix matches?
我有以下二进制搜索树代码,如果有人输入书名的前缀,后跟一个星号,递归方法将打印文本文件中与前缀匹配的所有书名。 例如,如果有人输入 Cor*,程序可能会在不同的行中返回“Coraline”、“Coral Reefs”和“Corpse Bride”。
我在下面编写的方法不会返回任何内容,如果有人能帮助我找出我出错的地方,我将不胜感激,因为我是递归和 BST 的新手。 我的插入和搜索方法工作正常,所以我知道错误出在我的 booksPrefix 方法中。
private void booksPrefix(Node root, String prefix) {
while (root != null) {
String rootLow = root.key.toLowerCase();
String prefixLow = prefix.toLowerCase();
//if current node starts with given prefix, print data
if (rootLow.startsWith(prefixLow)) {
System.out.println(root.data);
}
if (root.right != null) {
booksPrefix(root.right, prefix);
} if (root.left != null) {
booksPrefix(root.left, prefix);
}
}
}
这是 Tester 类中调用 booksPrefix() 的部分:
else if(search.indexOf('*') > 0)
{
//call prefix search
System.out.println("[Finding all movies that start with " + search.split("\\*")[0] + "..]");
bst.booksPrefix(search);
}
你的算法坏了。 这里有更好的东西:
if (rootLow.startsWith(prefixLow)) {
System.out.println(root.data);
}
if (root.hasChildren()) {
root.children.forEach(child -> booksPrefix(child, prefix));
}
尝试这个。
public class Node {
String key;
Node left, right;
Node(String key, Node left, Node right) {
this.key = key;
this.left = left;
this.right = right;
}
private void booksPrefix(Node node, String prefix, List<String> results) {
if (node == null) return;
String nodeLow = node.key.toLowerCase();
String prefixLow = prefix.toLowerCase();
if (nodeLow.startsWith(prefixLow)) results.add(node.key);
booksPrefix(node.right, prefixLow, results);
booksPrefix(node.left, prefixLow, results);
}
public List<String> booksPrefix(Node root, String prefix) {
List<String> results = new ArrayList<>();
booksPrefix(root, prefix, results);
return results;
}
}
和
Node root = new Node("Coraline",
new Node("Coral Reefs",
new Node("Apocalypse", null, null),
new Node("CorpseBride", null, null)),
new Node("Oddessy", null, null));
System.out.println(root.booksPrefix(root, "Cor"));
输出:
[Coraline, Coral Reefs, CorpseBride]
保持良好和简单:
private void booksPrefix(Node node, String prefix) {
if (node != null) {
booksPrefix(node.left, prefix);
if (node.key.toLowerCase().startsWith(prefix.toLowerCase()))
System.out.println(node.data);
booksPrefix(node.right, prefix);
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.