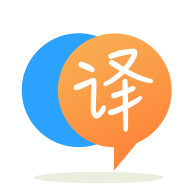
[英]How to use Switch and Enum Parameters (Not ENUM VALUE) in Java?
[英]How to use Enum if your value is not fixed?
我知道我不清楚我的问题,但我在这里尝试做的是使用枚举来转换温度单位,而且我所知道的枚举具有固定值,因此例如从华氏温度转换为摄氏温度需要输入计算才能工作但我不能使用主活动中的输入,所以我试图用它的 setter 声明一个输入,并使用类 Convert2 在主活动中设置输入(类似于 c.setInput(input))并获取它的值能够在课堂上使用它,认为如果你得到值,你可以将它添加到常量中,然后使最后的返回值等于 1 乘以乘数,乘数是 = 到常量,但这不起作用,我被困在这里,因为我是枚举的新手。 希望你能理解我在代码中的意思。
public class Convert2 {
private final double multiplier;
private double input;
public void setInput(double input) {
this.input = input;
}
public double getInput() {
return input;
}
public enum Unit2 {
Fahrenheit,
Celsius,
Kelvin,
;
public static Unit2 from(String text) {
if (text != null) {
for (Convert2.Unit2 unit2 : Convert2.Unit2.values()) {
if (text.equalsIgnoreCase(unit2.toString())) {
return unit2;
}
}
}
throw new IllegalArgumentException("Cannot find a value for " + text);
}
}
public Convert2(Convert2.Unit2 from, Convert2.Unit2 to) {
double constant = 1;
switch (from) {
case Fahrenheit:
if (to == Convert2.Unit2.Celsius) {
constant = (input - 32)
* 1.8;///here you can see how the calculation work as it needs the input value///
} else if (to == Convert2.Unit2.Kelvin) {
constant = (5 / 9) + 273.15 - 32;
}
break;
}
multiplier = constant;
}
public double convert2(double input) {
return (input - input + 1)
* multiplier;///(input-input+1) gives 1 multiplied by the multiplier gives the constant value///
}
}
您可以查看使用 switch 语句。 例如
如果你定义一个 Unit 枚举如下
enum Unit {
FAHRENHEIT,
CELSIUS,
KELVIN
}
一个完整的“转换”方法将评估所有情况:
f -> f
f -> c
f -> k
c -> f
c -> c
c -> k
k -> f
k -> c
k -> k
所以总的来说,你有类似的东西
public double convert(double input, Unit from, Unit to) {
if (from == null || to == null) {
throw new IllegalArgumentException("units cannot be null");
}
switch (from) {
case FAHRENHEIT:
switch (to) {
case FAHRENHEIT:
return input;
case CELSIUS:
return (input - 32) * (5D / 9D);
case KELVIN:
return (input + 459.67) * (5D / 9D);
default:
throw new IllegalStateException("Unhandled from / to values: " + from + " " + to);
}
case CELSIUS:
switch (to) {
case FAHRENHEIT:
return input * (9D / 5D) + 32;
case CELSIUS:
return input;
case KELVIN:
return input + 273.5;
default:
throw new IllegalStateException("Unhandled from / to values: " + from + " " + to);
}
case KELVIN:
switch (to) {
case FAHRENHEIT:
return (input - 273.5) * (9D / 5D) + 32;
case CELSIUS:
return input - 273.5;
case KELVIN:
return input;
default:
throw new IllegalStateException("Unhandled from / to values: " + from + " " + to);
}
default:
throw new IllegalStateException("Unhandled from value: " + from);
}
}
如果需要,您可以根据输入重构为更小的方法,以减少 switch 语句中的分支,从而稍微整理一下。
如果您使用的是 java 13 或更高版本,另一种方法是使用增强型开关。 此时, convert 方法将如下所示:
public double convert(double input, Unit from, Unit to) {
if (from == null || to == null) {
throw new IllegalArgumentException("units cannot be null");
}
return switch (from) {
case FAHRENHEIT -> switch (to) {
case FAHRENHEIT -> input;
case CELSIUS -> (input - 32) * (5D / 9D);
case KELVIN -> (input + 459.67) * (5D / 9D);
};
case CELSIUS -> switch (to) {
case FAHRENHEIT -> input * (9D / 5D) + 32;
case CELSIUS -> input;
case KELVIN -> input + 273.5;
};
case KELVIN -> switch (to) {
case FAHRENHEIT -> (input - 273.5) * (9D / 5D) + 32;
case CELSIUS -> input - 273.5;
case KELVIN -> input;
};
};
看到这里,我认为它更具可读性。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.