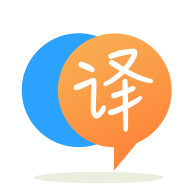
[英]Espresso: Getting a text value from a textview and storing in a String?
[英]Getting the value from textview
大家好,我为我的项目编写了一个代码,该代码是使用蓝牙将心跳传感器的值从 Arduino 获取到我的 android 手机。 到目前为止一切顺利,它可以毫无问题地将值发送到我的应用程序。 但现在的问题是我想获得它的价值,以便我可以使用我的算法,但现在似乎我陷入了困境。
这是代码:
package com.test.aplikasirevisi;
import java.io.IOException;
import java.io.InputStream;
import java.util.UUID;
import android.app.Activity;
import android.app.ProgressDialog;
import android.bluetooth.BluetoothAdapter;
import android.bluetooth.BluetoothDevice;
import android.bluetooth.BluetoothSocket;
import android.content.Intent;
import android.os.AsyncTask;
import android.os.Bundle;
import android.text.method.ScrollingMovementMethod;
import android.util.Log;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.CheckBox;
import android.widget.EditText;
import android.widget.ScrollView;
import android.widget.TextView;
import android.widget.Toast;
public class MonitoringScreen extends Activity {
private static final String TAG = "BlueTest5-MainActivity";
private int mMaxChars = 50000;//Default
private UUID mDeviceUUID;
private BluetoothSocket mBTSocket;
private ReadInput mReadThread = null;
private boolean mIsUserInitiatedDisconnect = false;
private TextView mTxtReceive;
private Button mBtnClearInput;
private ScrollView scrollView;
private CheckBox chkScroll;
private CheckBox chkReceiveText;
private boolean mIsBluetoothConnected = false;
private BluetoothDevice mDevice;
private ProgressDialog progressDialog;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_monitoring_screen);
ActivityHelper.initialize(this);
Intent intent = getIntent();
Bundle b = intent.getExtras();
mDevice = b.getParcelable(MainActivity.DEVICE_EXTRA);
mDeviceUUID = UUID.fromString(b.getString(MainActivity.DEVICE_UUID));
mMaxChars = b.getInt(MainActivity.BUFFER_SIZE);
Log.d(TAG, "Ready");
mTxtReceive = (TextView) findViewById(R.id.txtReceive);
chkScroll = (CheckBox) findViewById(R.id.chkScroll);
chkReceiveText = (CheckBox) findViewById(R.id.chkReceiveText);
scrollView = (ScrollView) findViewById(R.id.viewScroll);
mBtnClearInput = (Button) findViewById(R.id.btnClearInput);
mTxtReceive.setMovementMethod(new ScrollingMovementMethod());
mBtnClearInput.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View arg0) {
mTxtReceive.setText("");
}
});
}
private class ReadInput implements Runnable{
private boolean bStop = false;
private Thread t;
public ReadInput() {
t = new Thread(this, "Input Thread");
t.start();
}
public boolean isRunning() {
return t.isAlive();
}
@Override
public void run() {
InputStream inputStream;
try {
inputStream = mBTSocket.getInputStream();
while (!bStop) {
byte[] buffer = new byte[256];
if (inputStream.available() > 0) {
inputStream.read(buffer);
int i;
/*
* This is needed because new String(buffer) is taking the entire buffer i.e. 256 chars on Android 2.3.4 http://stackoverflow.com/a/8843462/1287554
*/
for (i = 0; i < buffer.length && buffer[i] != 0; i++) {
}
final String strInput = new String(buffer, 0, i);
/*
* If checked then receive text, better design would probably be to stop thread if unchecked and free resources, but this is a quick fix
*/
if (chkReceiveText.isChecked()) {
mTxtReceive.post(new Runnable() {
@Override
public void run() {
mTxtReceive.append(strInput);
int txtLength = mTxtReceive.getEditableText().length();
if(txtLength > mMaxChars){
mTxtReceive.getEditableText().delete(0, txtLength - mMaxChars);
System.out.println(mTxtReceive.getText().toString());
}
if (chkScroll.isChecked()) { // Scroll only if this is checked
scrollView.post(new Runnable() { // Snippet from http://stackoverflow.com/a/4612082/1287554
@Override
public void run() {
scrollView.fullScroll(View.FOCUS_DOWN);
}
});
}
}
});
}
}
Thread.sleep(500);
}
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
public void stop() {
bStop = true;
}
}
private class DisConnectBT extends AsyncTask<Void, Void, Void> {
@Override
protected void onPreExecute() {
}
@Override
protected Void doInBackground(Void... params) {
if (mReadThread != null) {
mReadThread.stop();
while (mReadThread.isRunning())
; // Wait until it stops
mReadThread = null;
}
try {
mBTSocket.close();
} catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
return null;
}
@Override
protected void onPostExecute(Void result) {
super.onPostExecute(result);
mIsBluetoothConnected = false;
if (mIsUserInitiatedDisconnect) {
finish();
}
}
}
private void msg(String s) {
Toast.makeText(getApplicationContext(), s, Toast.LENGTH_SHORT).show();
}
@Override
protected void onPause() {
if (mBTSocket != null && mIsBluetoothConnected) {
new DisConnectBT().execute();
}
Log.d(TAG, "Paused");
super.onPause();
}
@Override
protected void onResume() {
if (mBTSocket == null || !mIsBluetoothConnected) {
new ConnectBT().execute();
}
Log.d(TAG, "Resumed");
super.onResume();
}
@Override
protected void onStop() {
Log.d(TAG, "Stopped");
super.onStop();
}
@Override
protected void onSaveInstanceState(Bundle outState) {
// TODO Auto-generated method stub
super.onSaveInstanceState(outState);
}
private class ConnectBT extends AsyncTask<Void, Void, Void> {
private boolean mConnectSuccessful = true;
@Override
protected void onPreExecute() {
progressDialog = ProgressDialog.show(MonitoringScreen.this, "Hold on", "Connecting");// http://stackoverflow.com/a/11130220/1287554
}
@Override
protected Void doInBackground(Void... devices) {
try {
if (mBTSocket == null || !mIsBluetoothConnected) {
mBTSocket = mDevice.createInsecureRfcommSocketToServiceRecord(mDeviceUUID);
BluetoothAdapter.getDefaultAdapter().cancelDiscovery();
mBTSocket.connect();
}
} catch (IOException e) {
// Unable to connect to device
e.printStackTrace();
mConnectSuccessful = false;
}
return null;
}
@Override
protected void onPostExecute(Void result) {
super.onPostExecute(result);
if (!mConnectSuccessful) {
Toast.makeText(getApplicationContext(), "Could not connect to device. Is it a Serial device? Also check if the UUID is correct in the settings", Toast.LENGTH_LONG).show();
finish();
} else {
msg("Connected to device");
mIsBluetoothConnected = true;
mReadThread = new ReadInput(); // Kick off input reader
}
progressDialog.dismiss();
}
}
}
我想要的是获得 mTxtReceive 的价值:
int txtLength = mTxtReceive.getEditableText().length();
if(txtLength > mMaxChars){
mTxtReceive.getEditableText().delete(0, txtLength - mMaxChars);
System.out.println(mTxtReceive.getText().toString());
}
我使用 System.out.println 来查看我是否得到了值,但在日志中它没有显示任何内容。 所以我需要你们的智慧对此有什么帮助吗?
您的视图mTextReceive
似乎是一个TextView
,因此不可编辑:
private TextView mTxtReceive;
如果它不是EditText
(可编辑),则mTxtReceive.getEditableText
将返回null
请参阅 docs并且应该调用getText
而不是see docs 。
因此,您的条件可能总是从if(txtLength > mMaxChars)
解析为if(null > 50000)
始终为false
(或者实际上它甚至可能之前崩溃),因此您在 if 块中的代码永远不会执行
尝试:
// recommended for debugging. Check if this is even called and if text length is really longer than max chars
Log.d(TAG, "text length:" + mTxtReceive.getText().length());
int txtLength = mTxtReceive.getText().length();
if(txtLength > mMaxChars){
// not sure what operation you want to do here but leave out for debugging
Log.d(TAG, "text longer than allowed:" + mTxtReceive.getText().toString());
}
还要使用Log.d
而不是System.out.println
因为否则您的日志可能不会转发到 Logcat。 另外你确定这个块被执行了吗? 我会放一个Log.d(TAG, "text length:" + mTxtReceive.getText().length());
出于调试目的而超出条件。 最后一个明显的问题是txtLength
是否比 max chars 更长(这将是一个包含 50000 个字符的相当长的文本)。 但是您也可以简单地使用块外的推荐日志进行验证。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.