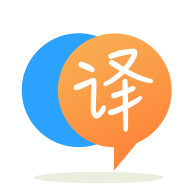
[英]Why I can't take a screenshot using selenium webdriver in java?
[英]How can I store a screenshot from an object to a string and save the image into an excel worksheet in selenium WebDriver?
我有一个简单的 selenium WebDriver 程序,它读取 excel 文件数据并执行登录功能。
每当测试用例失败时,我都需要截屏并将其保存到 excel 工作表单元格中。
为了完成excel的读写,我已经创建了代码。 我还编写了一个程序,它可以捕获屏幕截图并以唯一的名称保存它们。 该程序位于实用程序 package 中的单独 class 中,我使用其实例/对象调用 MAIN 方法。
I want to store the object of the FailedTestScreenCapture class into a string variable and extract the image captured from inside this object, then save it in the excel worksheet.
下面是我创建的用于捕获屏幕截图并使用唯一名称保存它们的代码。
package utilities;
import java.io.File;
import java.util.UUID;
import org.openqa.selenium.io.FileHandler;
import org.junit.rules.MethodRule;
import org.junit.runners.model.FrameworkMethod;
import org.junit.runners.model.Statement;
import org.openqa.selenium.OutputType;
import org.openqa.selenium.TakesScreenshot;
import org.openqa.selenium.WebDriver;
public class FailedTestScreenCapture implements MethodRule {
WebDriver driver;
public FailedTestScreenCapture(WebDriver driver) {
this.driver = driver;
}
public Statement apply(final Statement statement, final FrameworkMethod frameworkMethod, final Object obj) {
// Auto-generated method stub
return new Statement() {
@Override
public void evaluate() throws Throwable {
try {
statement.evaluate();
} catch (Throwable t) {
// handle exception
captureScreen(frameworkMethod.getName());
throw t;
}
}
public void captureScreen(String screenShot) {
// creating a screenshot method
try {
TakesScreenshot ts = (TakesScreenshot) driver;
File source = ts.getScreenshotAs(OutputType.FILE);
screenShot += UUID.randomUUID().toString();
FileHandler.copy(source, new File("./Screenshots/" + screenShot + ".jpg"));
} catch (Exception e) {
System.out.println("An exception occured while taking the screenshot" + e.getMessage());
}
}
};
}
}
下面是读写 excel 工作表的代码。
package view;
import java.io.IOException;
import java.time.Duration;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import utilities.ExcelUtils;
import utilities.FailedTestScreenCapture;
import utilities.Constants;
public class OTMGeneral {
static WebDriver driver;
// creating object of ExcelUtils class
static ExcelUtils excelUtils = new ExcelUtils();
static FailedTestScreenCapture failedTstCase = new FailedTestScreenCapture(driver);
// using the constants class values for excel file path
static String excelFilePath = Constants.Path_TestData + Constants.File_TestData;
public static void main(String args[]) throws IOException {
System.setProperty("webdriver.chrome.driver", Constants.chromeDriverPath);
driver = new ChromeDriver();
// maximize the browser screen
driver.manage().window().maximize();
driver.navigate().to(Constants.BaseUrl);
// wait for the browser to load resources
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(20));
// Identify the WebElements for OTM Login
WebElement continueBtn = driver.findElement(By.xpath("/html/body/div[1]/div/div/div[2]/button"));
WebElement loginBtn = driver.findElement(By.xpath("/html/body/header/div/div/nav/div/ul/li[2]/a"));
// landing page pop-up message
WebElement popupMsg = driver.findElement(By.xpath("/html/body/div[1]/div/div"));
// click the sing-in button
if (popupMsg.isDisplayed()) {
// if the pop-up is displayed, click the continue button
continueBtn.click();
// wait for page to come back to landing page after continue button is clicked
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(20));
// click the login button
loginBtn.click();
} else {
loginBtn.click();
}
WebElement email = driver.findElement(By.id("Email"));
WebElement pwd = driver.findElement(By.id("Password"));
WebElement signInBtn = driver.findElement(By.id("btnlogin"));
// calling ExcelUtils class method to initialize the workbook and sheet
excelUtils.setExcelFile(excelFilePath, "Login_Data");
for (int i = 1; i <= excelUtils.getRowCountInSheet(); i++) {
if (i > 1) {
// Handling staled elements by refreshing the screen
driver.navigate().refresh();
driver.findElement(By.xpath("/html/body/header/div/div/nav/div/ul/li[2]/a")).click();
driver.findElement(By.id("Email")).sendKeys(excelUtils.getCellData(i, 0));
driver.findElement(By.id("Password")).sendKeys(excelUtils.getCellData(i, 1));
driver.findElement(By.id("btnlogin")).click();
} else {
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(20));
// Enter the value read from excel in email and password fields
email.sendKeys(excelUtils.getCellData(i, 0));
pwd.sendKeys(excelUtils.getCellData(i, 1));
// click on login button
signInBtn.click();
}
// wait after login button clicked
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(20));
// check for login pass or fail
boolean helloCustomer = driver.findElement(By.xpath("/html/body/header/div/div/nav/div/ul/li[1]/a"))
.getText().equalsIgnoreCase("Regístrate");
System.out.println(helloCustomer);
if (!helloCustomer) {
// capture the result in the excel sheet
excelUtils.setCellValue(i, 2, "Pass", excelFilePath);
System.out.println("login pass");
// Identify logout button
WebElement logout = driver.findElement(By.id("logout"));
logout.click();
} else {
excelUtils.setCellValue(i, 2, "Fail", excelFilePath);
System.out.println("login fail");
// capture screenshot when a test case fails
excelUtils.setCellValue(i, 3, failedTstCase.toString(), excelFilePath);
}
// wait for page to come back to registration page after logout button is
// clicked
driver.manage().timeouts().implicitlyWait(Duration.ofSeconds(20));
}
// closing the driver
driver.quit();
}
}
您可以通过将图像转换为byte[]
然后在所需位置绘制图像来做到这一点。 你可以这样做,
在您的OTMGeneral
class 中:
if (!helloCustomer) {
//Your code
} else {
excelUtils.setCellValue(i, 2, "Fail", excelFilePath);
System.out.println("login fail");
// capture screenshot when a test case fails
excelUtils.attachImageToExcel("NameOfScreenshot", i, 3, 0.3);
}
将attachImageToExcel
方法保留在您的ExcelUtils
中。 您需要通过以下@Params
。
@Param screenShot - The name what you assigned to the image while capturing.
@Param column - Column number
@Param row - Row number
@Param image size - Pass the float value. Example 0.3 = 30% image
代码:
public static void attachImageToExcel(String screenShot, int column, int row, double imageSize)
throws Exception {
Workbook wb = new XSSFWorkbook(new FileInputStream(new File("FileLocation")));
Sheet sheet = wb.getSheet("SheetName");
InputStream inputStream = new FileInputStream("./Screenshots/" + screenShot + ".jpg");
byte[] bytes = IOUtils.toByteArray(inputStream);
int pictureIdx = wb.addPicture(bytes, Workbook.PICTURE_TYPE_PNG);
inputStream.close();
CreationHelper helper = wb.getCreationHelper();
Drawing drawing = sheet.createDrawingPatriarch();
ClientAnchor anchor = helper.createClientAnchor();
anchor.setCol1(column);
anchor.setRow1(row);
Picture pict = drawing.createPicture(anchor, pictureIdx);
pict.resize(imageSize);
FileOutputStream fileOut = null;
fileOut = new FileOutputStream((new File("FileLocation")));
wb.write(fileOut);
fileOut.close();
}
Output :
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.