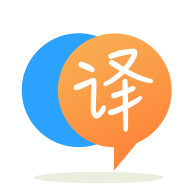
[英]C Program - Why is my card shuffling function not working correctly?
[英]Why is my C program not counting correctly? Card game
我创建了一个纸牌游戏,它比较两个玩家的两张牌,并根据特定条件确定获胜者。 我有一个 function 比较卡片并根据回合的获胜者返回真或假。 我在运行游戏的 function 的循环中调用它,迭代次数基于发给每个玩家的牌数。 我的比较卡 function 有一些东西允许某些回合无法通过比较测试,我不知道为什么。 结果是我的球员获胜计数在比赛结束时被取消。 我试过在不同的地方玩这些条件,但我找不到问题。
比较卡片 function - 在看似随机的回合中,最后的打印条件将执行,告诉我我已经通过其他条件
bool compareCards(char *card1, char *card2)
{
char card1Face = card1[0];
char card2Face = card2[0];
//if card1 is A/K/Q/J and card2 is num
if ((card1Face >= 'A' && card1Face <= 'Z') && !(card2Face >= 'A' && card2Face <= 'Z'))
{
return true;
}
//if card1 is num and card2 is A/K/Q/J
else if (!(card1Face >= 'A' && card1Face <= 'Z') && (card2Face >= 'A' && card2Face <= 'Z'))
{
return false;
}
//if card1 and card2 both A/K/Q/J
else if ((card1Face >= 'A' && card1Face <= 'Z') && (card2Face >= 'A' && card2Face <= 'Z'))
{
//test for A
if (card1Face == 'A' && card2Face != 'A')
{
return true;
}
else if (card1Face != 'A' && card2Face == 'A')
{
return false;
}
//test for K
if (card1Face == 'K' && card2Face != 'K')
{
return true;
}
else if (card1Face != 'K' && card2Face == 'K')
{
return false;
}
//test for Q
if (card1Face == 'Q' && card2Face != 'Q')
{
return true;
}
else if (card1Face != 'Q' && card2Face == 'Q')
{
return false;
}
//test for J
if (card1Face == 'J' && card2Face != 'J')
{
return true;
}
else if (card1Face != 'J' && card2Face == 'J')
{
return false;
}
} //end cards are face cards
//If card1 and card2 are nums
else if ((card1Face >= '1' && card1Face <= '9') && (card2Face >= '1' && card2Face <= '9'))
{
//if card1 is 10 and card2 is not
if (card1Face == '1' && card2Face != '1')
{
return true;
}
//if card2 is 10 and card1 is not
else if (card1Face != '1' && card2Face == '1')
{
return false;
}
//if card1 higher than card2
else if (card1Face > card2Face)
{
return true;
}
//if card1 lower than card2
else if (card1Face < card2Face)
{
return false;
}
} //end cards are nums
//if card1 and card2 same face value
else if (card1Face == card2Face)
{
char card1Suit = card1[strlen(card1) - 1];
char card2Suit = card2[strlen(card2) - 1];
//test for spade
if (card1Suit == 'S')
{
return true;
}
else if (card2Suit == 'S')
{
return false;
}
//test for Hearts
if (card1Suit == 'H')
{
return true;
}
else if (card2Suit == 'H')
{
return false;
}
//test for Diamonds
if (card1Suit == 'D')
{
return true;
}
else if (card2Suit == 'D')
{
return false;
}
//test for Clubs
if (card1Suit == 'C')
{
return true;
}
else if (card2Suit == 'C')
{
return false;
}
}
printf("IF THIS PRINTS UH OH");
return EXIT_SUCCESS;
}
主要玩法游戏function
void playGame(void)
{
srand(time(NULL));
//initialize deck
char *(*deck)[13] = initializeDeck();
deck = shuffleDeck(deck);
bool quit = false;
while (1)
{
printHeaderDeal();
//user input loop
while (1)
{
fflush(stdin);
char choice = getchar();
//if enter to deal
if (choice == '\n')
{
break;
}
//if q to quit
else if (choice == 'q' || choice == 'Q')
{
printf("You chose to quit\n");
quit = true;
break;
}
//handling wrong input
else
{
printf("Incorrect choice! Please choose ENTER to deal or q to quit. \n");
scanf("%*[^\n]%*c");
}
} //end user input
//if quit selected break from loop
if (quit == true)
{
break;
}
int numCardsToDeal;
//user selects number of cards to deal
while (1)
{
printf("How many cards would you like to deal? 2-20\n");
scanf(" %d", &numCardsToDeal);
scanf("%*[^\n]");
//set range = even number between 2-20
if (numCardsToDeal >= 2 && numCardsToDeal <= 20)
{
if (numCardsToDeal % 2 == 1)
{
printf("Players must have same amount of cards in hand. Pick an even amount to deal.\n");
}
else
{
break;
}
}
else
{
printf("Incorrect selection.\n");
}
}//end num to deal selection and validation
//call deal cards and subtract the return value from the remaining card total
cardsRemaining -= dealCards(deck, numCardsToDeal);
bool result;
这是我打印玩家手牌的地方,调用比较 function 并根据每一轮计算获胜次数
int length1 = sizeof(player1Hand)/sizeof(player1Hand[0]);
printf("\n\n");
printf("Player 1 hand: ");
for(int i = 0; i < length1; i++)
{
printf("[%s]", player1Hand[i]);
}
printf("\n\n");
int length2 = sizeof(player2Hand)/sizeof(player2Hand[0]);
printf("Player 2 hand: ");
for(int i = 0; i < length2; i++)
{
printf("[%s]", player2Hand[i]);
}
printf("\n\n");
//loop through player hands based on num of dealt cards, call compareCards on each iteration and store results of each comparison
for (int i = 0; i < (numCardsToDeal / 2); i++)
{
result = compareCards(player1Hand[i], player2Hand[i]);
if (result == true)
{
player1Wins++;
}
else if (result == false)
{
player2Wins++;
}
}
//if run out of cards end game
if (cardsRemaining == 0)
{
printf("No more cards remaining in deck. Game over.\n\n");
break;
}
} //end game
printf("Total wins for player 1: %d\n", player1Wins);
printf("Total wins for player 2: %d\n", player2Wins);
free(deck);
}
我不确定这是否真的能解决问题,但这对于评论来说太长了:
可能还有其他一些逻辑问题,但我注意到的第一件事是在您对带有数字的卡片的测试中:
//If card1 and card2 are nums
else if ((card1Face >= '1' && card1Face <= '9') && (card2Face >= '1' && card2Face <= '9'))
{
// snipped
} //end cards are nums
如果card1Face
和card2Face
都是同一个数字,则没有条件匹配,所以它会失败。
请注意,即使您有另一个else if
测试卡是否相等,这在这种情况下并且不会运行,因为您已经匹配了一个else if
条件。
因此,这可能无法完全解决问题,但我认为如果您将card1Face == card2Face
的检查移至较早的检查之一,那么您至少可以删除一些无法正常工作的情况期待。 实际上,我认为您应该将等卡检查作为第一个检查; 使if
条件card1Face == card2Face
,然后所有其他条件为else if
。
最后,为了调试,如果修改后仍然不能正常工作,添加一个else
块,只打印两个卡值; 您可能会开始看到一种模式,该模式将有助于找到可能发生其他问题的位置。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.