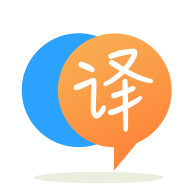
[英]How to compare two array of objects and return the not matching object?
[英]Compare two array of objects and return matching values in a new array
我有两个 arrays 对象: inputData
和jobList
。 我必须比较两个对象数组的 primarySkills 数组,并仅返回两个数组中匹配的值。我的对象数组如下:
let inputData = [
{
"candidateID": "911772331",
"skillSet": ["Information Technology"],
"addressCity": "Bengaluru",
"addressState": "KA",
"country": "India",
"primarySkills": ['asp.net', 'react', 'javascript'],
"secondarySkills": ['powerbi', 'redux'],
"preferredPositionType": [],
}
]
let jobList = [
{
jobId: '600039355',
jobType: 'fulltime',
primarySkills: [ 'asp.net','node' ],
secondarySkills: [ 'javascript' ],
skillSet: [ 'javascript' ],
Address: 'Indonesia, Bekasi Kabupaten, 53, Jalan Londan 5',
City: 'Bekasi Kabupaten',
State: 'JABODETABEK',
Zipcode: '17522',
Country: 'Indonesia'
},
{
jobId: '562190375',
jobType: 'fulltime',
primarySkills: [ 'javascript','mainframe' ],
secondarySkills: [ 'javascript' ],
skillSet: [ 'javascript' ],
Address: 'India, Pune, 411001, Pune, Pune Station',
City: 'Pune',
State: 'MH',
Zipcode: '411001',
Country: 'India'
},
{
jobId: '883826845',
jobType: 'fulltime',
primarySkills: [ 'sqlserver', 'react', 'powershell' ],
secondarySkills: [ 'powerbi' ],
skillSet: [ 'powerbi' ],
Address: 'ประเทศไทย, หมู่ที่ 3, 1234',
City: 'หมู่ที่ 3',
State: null,
Zipcode: '57110',
Country: 'ประเทศไทย'
}
]
我已经完成了下面提到的代码来实现这一点:
jobList.forEach((item) => {
inputData.forEach((data) => {
for (let i = 0; i <= item.primarySkills.length; i++) {
for (let j = 0; j <= data.primarySkills.length; j++) {
if (item.primarySkills[i] === data.primarySkills[j]) {
PMSkill.push(item.primarySkills[i]);
} else {
PMSkill.push(0)
}
}
}
})
})
Expected output to be like in the PMSkill array:
let PMSkill= [
{
jobId: '600039355',
jobType: 'fulltime',
primarySkills: [ 'asp.net'],----here asp.net is the only skill matching with inputData primarySkill
secondarySkills: [ 'javascript' ],
skillSet: [ 'javascript' ],
Address: 'Indonesia, Bekasi Kabupaten, 53, Jalan Londan 5',
City: 'Bekasi Kabupaten',
State: 'JABODETABEK',
Zipcode: '17522',
Country: 'Indonesia'
},
{
jobId: '562190375',
jobType: 'fulltime',
primarySkills: [ 'javascript'],
secondarySkills: [ 'javascript' ],
skillSet: [ 'javascript' ],
Address: 'India, Pune, 411001, Pune, Pune Station',
City: 'Pune',
State: 'MH',
Zipcode: '411001',
Country: 'India'
},
{
jobId: '883826845',
jobType: 'fulltime',
primarySkills: ['react'],
secondarySkills: [ 'powerbi' ],
skillSet: [ 'powerbi' ],
Address: 'ประเทศไทย, หมู่ที่ 3, 1234',
City: 'หมู่ที่ 3',
State: null,
Zipcode: '57110',
Country: 'ประเทศไทย'
}
]
嗨,有一个简单的方法,我经常使用
function arr_intersection(a1: number[], a2: number[]) {
return a1.filter((x) => a2.includes(x));
}
你也可以在 lodash 中使用交集方法
const _ = require("lodash");
// Original array
let array1 = [1, 2, 4, 3, 4, 4]
let array2 = [2, 4, 5, 6]
let array3 = [2, 3, 5, 6]
// Using _.intersection() method
let newArray = _.intersection(
array1, array2, array3);
// Printing original Array
console.log("original Array1: ", array1)
console.log("original Array2: ", array2)
console.log("original Array3: ", array3)
// Printing the newArray
console.log("new Array: ", newArray)
在这里你可以发送多个数组,不像我的方法
function getIntersection(x, y) { // ensure two arrays... const [ comparisonBase, //... the shorter one as comparison base comparisonList, //... the longer one to filter from. ] = [[...x], [...y]].sort((a, b) => a.length - b.length); // create a `Map` based lookup table from the shorter array. const itemLookup = comparisonBase.reduce((map, item) => map.set(item, true), new Map) // the intersection is the result of following filter task. return comparisonList.filter(item => itemLookup.has(item)); } const inputData = [{ "candidateID": "911772331", "skillSet": ["Information Technology"], "addressCity": "Bengaluru", "addressState": "KA", "country": "India", "primarySkills": ['asp.net', 'react', 'javascript'], "secondarySkills": ['powerbi', 'redux'], "preferredPositionType": [], }]; const jobList = [{ jobId: '600039355', jobType: 'fulltime', primarySkills: [ 'javascript' ], secondarySkills: [ 'javascript' ], skillSet: [ 'javascript' ], Address: 'Indonesia, Bekasi Kabupaten, 53, Jalan Londan 5', City: 'Bekasi Kabupaten', State: 'JABODETABEK', Zipcode: '17522', Country: 'Indonesia' }, { jobId: '562190375', jobType: 'fulltime', primarySkills: [ 'javascript' ], secondarySkills: [ 'javascript' ], skillSet: [ 'javascript' ], Address: 'India, Pune, 411001, Pune, Pune Station', City: 'Pune', State: 'MH', Zipcode: '411001', Country: 'India' }, { jobId: '883826845', jobType: 'fulltime', primarySkills: [ 'sqlserver', 'azure', 'powershell' ], secondarySkills: [ 'powerbi' ], skillSet: [ 'powerbi' ], Address: 'ประเทศไทย, หมู่ที่ 3, 1234', City: 'หมู่ที่ 3', State: null, Zipcode: '57110', Country: 'ประเทศไทย' }]; const candidateSkills = inputData[0].primarySkills; const openJobsSkills = [...new Set( jobList.reduce((arr, { primarySkills }) => arr.concat(primarySkills), []) )]; const skillIntersection = getIntersection(openJobsSkills, candidateSkills); console.log({ candidateSkills, openJobsSkills, skillIntersection });
.as-console-wrapper { min-height: 100%;important: top; 0; }
像这样更改您的验证
matchingPrimarySkills =[]
jobList.forEach((job) => {
inputData.forEach((candidate) => {
job.primarySkills.forEach( (jobPrimarySkill) => {
candidate.primarySkills.forEach( (candidatePrimarySkill) => {
if (jobPrimarySkill === candidatePrimarySkill && matchingPrimarySkills.indexOf(jobPrimarySkill) === -1) {
matchingPrimarySkills.push(jobPrimarySkill);
}
})
})
})
})
// With your examples, it will og [ "javascript"] without duplicates
console.log(matchingPrimarySkills)
这是实现所需 output 的一种方法。 仅显示相关属性仅用于演示。 您可以将inputData
中的候选人传递给getJobs(input)
function 以从输入中获取与某些primarySkills
匹配的工作列表:
let inputData = [ { candidateID: '111111', primarySkills: ['asp.net', 'react', 'javascript'] }, { candidateID: '222222', primarySkills: ['python'] }, { candidateID: '333333', primarySkills: ['powershell', 'node'] }, ] let jobList = [ { jobId: '77777', primarySkills: ['asp.net', 'node'] }, { jobId: '88888', primarySkills: ['javascript', 'python'] }, { jobId: '99999', primarySkills: ['sqlserver', 'azure', 'powershell'] }, ] function getJobs(input) { // Get a copy of jopList const temp = jobList.map(obj => { return {...obj } }) return temp.filter(job => { // Filter the job's primarySkills to only show // skills matching the candidates's primarySkills. job.primarySkills = job.primarySkills.filter(jobSkill => input.primarySkills.includes(jobSkill) ) return job.primarySkills.length }) } // Get jobs for all candidates in inputData for (let candidate of inputData) { console.log(`\nJobs for candidateID ${candidate.candidateID}:\n`, getJobs(candidate)) }
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.