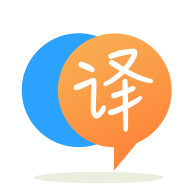
[英]Spring Boot - Load application.properties/yml from dependent jar
[英]Load dynamic objects from application.properties in Spring Boot
我目前在 Spring Boot 中有一个微服务,其中我有一些属性存储在 application.properties 中。 目前,它仅配置为一个商店。 但我想更改逻辑,以便可以动态添加商店而无需更改任何代码。
我为商店创建了一个 model java class ,它看起来像这样:
public class Store {
private String username;
private String password;
private String hostname;
private int port;
}
我的 application.properties 今天看起来像这样:
system.connection.username=TestUser
system.connection.password=123456
system.connection.hostname=12.34.56.78
system.connection.port=1234
但我希望能够指定它是哪个商店,并且能够通过将它们添加到 application.properties 中来添加更多商店,如下所示:
system.connection.Store1.username=TestUser1
system.connection.Store1.password=123456
system.connection.Store1.hostname=12.34.56.78
system.connection.Store1.port=1234
system.connection.Store2.username=TestUser2
system.connection.Store2.password=859382
system.connection.Store2.hostname=34.34.34.34
system.connection.Store2.port=4444
然后将其添加到一些 hashmap 中,并能够从我的任何其他类中检查和检索特定商店的变量。
为此,我尝试了以下方法:
@Configuration
@ConfigurationProperties(prefix = "system.connection")
class StoresConfiguration(
val stores: HashMap<String, Store> = HashMap()
) {
fun getStore(storeName: String): Store? {
return stores.get(storeName)
}
}
但是getStore只返回null,即使我检查stores.size它也是0。那么我在哪里做错了,我怎样才能有效地做到这一点? 我曾想过跳过废话,只创建一个名为“stores”的文件夹,然后将 json 文件放入其中,然后从那里读取。 因此,每个 Store 只是一个 json 文件,因此在启动时应用程序会扫描文件夹,并为每个 json 文件创建一个 Store object。 但我不知道这是否是一个好的解决方案。 这是我第一次使用 Spring Boot。 谢谢你的帮助!
这会有帮助吗? 这是在ConfigurationProperties
class 中使用索引列表。
@ConfigurationProperties("system.connection")
// use a fitting name here
public class Stores {
// reference your Store type
private final List<Store> store = new ArrayList<>();
public List<Store> getStore() {
return this.store;
}
}
system.connection.store[0].username=foo
system.connection.store[0].password=pass1
...
system.connection.store[1].username=bar
system.connection.store[1].password=pass2
稍后,上面的链接中还给出了 map 的示例,但带有索引的列表方法可能更适合您的需求。
使用 store 作为数组来解决问题。
通过玩一点,我设法解决了这个问题。 我将 class 恢复为 Kotlin,并对检索值的方式进行了一些调整。 这是您可以从 Spring 中的 application.properties 加载动态对象的方法 使用 Kotlin 启动:
假设您有一个名为 Store.kt 的 object class
data class Store(
var name: String ?= null,
var username: String ?= null,
var password: String ?= null,
var hostname: String ?= null,
var port: Int ?= null,
)
在你的 application.properties
system.stores.Store1.name=Test1
system.stores.Store1.username=test1
system.stores.Store1.password=123456
system.stores.Store1.hostname=12.34.56.78
system.stores.Store1.port=1234
system.stores.Store2.name=Test2
system.stores.Store2.username=test2
system.stores.Store2.password=123456
system.stores.Store2.hostname=12.34.56.78
system.stores.Store2.port=1234
这是在您的 StoresConfiguration.kt
@Configuration
@ConfigurationProperties(prefix = "system")
class StoreConfiguration(
var stores: HashMap<String, Store> = HashMap()
) {
fun getStore(storeName: String) : Store? {
return stores.get(storeName)
}
}
然后假设您想要获取对象并在您的服务类之一中使用它们:
@Service
class StoreService(
private val storeConfiguration: StoreConfiguration,
) {
fun test() {
val MyStore = storeConfiguration.getStore("Store1")
if (MyStore != null) {
// Do whatever you want to do with your object
} else {
// If your object does not exist
}
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.