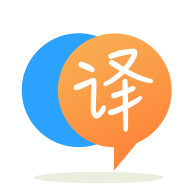
[英]How to check if the Firebase Stripe extension has finished creating the customer/user document?
[英]ios stripe not creating stripe customer
这是我的 index.js -
const functions = require('firebase-functions');
const admin = require('firebase-admin')
const stripe = require('stripe') ('sk_test_51HmAsMFzRM1fSBZ2CkBBjW5a3sEpxEQLGT7OkGxa8BZMMfgzT1Jeqs7dq63AlPRLIJmzHFC0HHAmKHDazcpriuh9001IWIVmw1');
admin.initializeApp()
const db = admin.firestore()
const storage = admin.storage().bucket()
const auth = admin.auth()
exports.createStripeUser = functions.https.onCall( async (data, context) => {
const email = data.email
const uid = context.auth.uid
if (uid === null) {
throw new functions.https.HttpsError('internal', 'Illegal access attempt')
}
return stripe.customers.create({
email: email,
})
.then(customer => {
return customer["id"]
})
.then( customerId => {
admin.firestore().collection('users').doc(uid).set(
{
stripeId: customerId,
email: email,
id: uid
}
)
})
.catch( err => {
throw new functions.https.HttpsError('internal', 'Unable to create Stripe customer')
})
})
exports.createEphemeralKey = functions.https.onCall(async (data, context) => {
const customerId = data.customer_id;
const stripeVersion = data.stripe_version;
const uid = context.auth.uid;
if (uid === null) {
console.log('Illegal access attempt due to unauthenticated attempt.')
throw new functions.https.HttpsError('internal', 'Illegal access attempt');
}
return stripe.ephemeralKeys.create(
{ customer: customerId },
{ stripe_version: stripeVersion }
).then((key) => {
return key
}).catch( (err) => {
functions.logger.log('Error creating ephemeral key', err)
throw new functions.https.HttpsError('internal', 'Unable to create ephemeral key: ' + err)
})
})
exports.createPaymentIntent = functions.https.onCall( async (data, context) => {
const total = data.total
const idempotency = data.idempotency
const customer = context.auth.uid
const uid = context.auth.uid;
if (uid === null) {
console.log('Illegal access attempt due to unauthenticated attempt.')
throw new functions.https.HttpsError('internal', 'Illegal access attempt');
}
return stripe.paymentIntents.create(
{
amount: total,
currency: 'usd',
customer: customer,
payment_method_types: ['card', 'ach_debit']
},
{
idempotencyKey: idempotency
}
).then( intent => {
return intent.client_secret
}).catch( err => {
functions.logger.log('Unable to create Stripe Payment Intent.')
return null
})
})
exports.fetchBankSources = functions.https.onCall(async (data, context) => {
const customerId = data.customer_id
const bankAccounts = await stripe.customers.listSources(
customerId,
{ object: 'bank_account'}
)
return { banks: bankAccounts.data }
})
exports.deleteSource = functions.https.onCall(async (data, context) => {
const customerId = data.customer_id
const source = data.source
return stripe.customers.deleteSource(customerId, source)
.then(res => {
return {
status: 200
}
})
.catch(err => {
functions.logger.log(err)
throw new functions.https.HttpsError('internal', 'Unable to delete bank source: ' + err)
})
})
// PLAID
const plaid = require('plaid');
exports.createPlaidLinkToken = functions.https.onCall(async (data, context) => {
const customerId = context.auth.uid
const env = functions.config().app.env
let plaidEnv = plaid.environments.sandbox
if (env === 'production') {
plaidEnv = plaid.environments.development
} else {
plaidEnv = plaid.environments.sandbox
}
const plaidClient = new plaid.Client({
clientID: functions.config().plaid.client_id,
secret: functions.config().plaid.secret,
env: plaidEnv,
options: {
version: '2019-05-29'
}
})
return plaidClient.createLinkToken({
user: {
client_user_id: customerId
},
client_name: 'Bon Voyage',
products: ['auth'],
country_codes: ['US'],
language: 'en',
redirect_uri: 'https://bon-voyage.jonnybcodes.com'
})
.then((apiResponse) => {
const linkToken = apiResponse.link_token
return linkToken
})
.catch((err) => {
functions.logger.log(err)
throw new functions.https.HttpsError('internal', 'Unable to create plaid link token: ' + err)
})
})
exports.createPlaidBankAccount = functions.https.onCall(async (data, context) => {
const token = data.publicToken
const custId = data.stripeId
const bankAccountId = data.accountId
const env = functions.config().app.env
let plaidEnv = plaid.environments.sandbox
if (env === 'production') {
plaidEnv = plaid.environments.development
} else {
plaidEnv = plaid.environments.sandbox
}
const plaidClient = new plaid.Client({
clientID: functions.config().plaid.client_id,
secret: functions.config().plaid.secret,
env: plaidEnv,
options: {
version: '2019-05-29'
}
})
return plaidClient.exchangePublicToken(token)
.then(res => {
const accessToken = res.access_token
return plaidClient.createStripeToken(accessToken, bankAccountId)
})
.then(resp => {
const bankAccountToken = resp.stripe_bank_account_token
return stripe.customers.createSource(custId, {
source: bankAccountToken
}).then(bank => {
return bank
})
})
.catch((err) => {
functions.logger.log(err)
throw new functions.https.HttpsError('internal', 'Unable to create plaid link token: ' + err)
})
})
功能显示部署在控制台中,如下所示:-
这是我的视图控制器-
import UIKit
import FirebaseFirestore
import FirebaseAuth
import Stripe
import FirebaseFunctions
class SignUpViewController: UIViewController {
var paymentContext = STPPaymentContext()
@IBOutlet weak var email: UITextField!
@IBOutlet weak var password: UITextField!
@IBOutlet weak var passwordConfirm: UITextField!
@IBAction func signUpAction(_ sender: Any) {
if password.text != passwordConfirm.text {let alertController = UIAlertController(title: "Password Incorrect", message: "Please re-type password", preferredStyle: .alert)
let defaultAction = UIAlertAction(title: "OK", style: .cancel, handler: nil)
alertController.addAction(defaultAction)
self.present(alertController, animated: true, completion: nil)
}else{
Auth.auth().createUser(withEmail: email.text!, password: password.text!){ (user, error) in if error == nil {
self.performSegue(withIdentifier: "signupToHome", sender: self)
}
else{
let alertController = UIAlertController(title: "Error", message: error?.localizedDescription, preferredStyle: .alert)
let defaultAction = UIAlertAction(title: "OK", style: .cancel, handler: nil)
alertController.addAction(defaultAction)
self.present(alertController, animated: true, completion: nil)
}
}
}
Functions.functions().httpsCallable("createStripeUser").call(["email": email.text ?? ""]) { (result, error) in
if let error = error {
debugPrint(error.localizedDescription)
return
}
self.dismiss(animated: true)
}
}
}
我究竟做错了什么? 据我所知,一切都是正确的。 功能部署好了,在firebase控制台创建了用户,为什么Stripe Dashboard没有创建客户?
嘿,代码没有问题我遇到了同样的问题,我通过注销或退出模拟器并在新模拟器上运行应用程序来修复它。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.