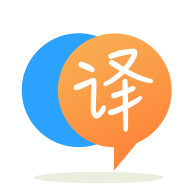
[英]Create triangle with numbers in this pattern using for loops in java?
[英]How to make a numbers triangle in Java using loops, numbers starting from right
我的项目是在 Java 中创建一个三角形,数字从右边开始
import java.util.Scanner;
public class Pyramid {
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
// loop
for (int i=1; i <= 6; i++) {
// space
for(int j=1; j <= 6-i; j++)
System.out.print(" ");
// numbers
for(int k= 1; k <= i; k++)
System.out.print(k);
// new line
System.out.println();
}
}
}
那么你唯一需要做的就是改变顺序,就像你首先添加空格然后数字而不是添加数字然后空格一样。 代码 -
import java.util.Scanner;
public class Pyramid {
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
// loop
for (int i=1; i <= 6; i++) {
// numbers
for(int k= 1; k <= i; k++)
System.out.print(k);
// space
for(int j=1; j <= 6-i; j++)
System.out.print(" ");
// new line
System.out.println();
}
}
}
现在我们得到结果——
在此处输入图像描述import java.util.Scanner;
公共 class 金字塔 {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int lines = scanner.nextInt();
for (int i = 0; i <= lines; i++) {
//space
int spaceNum = lines - i;
for (int j = 0; j < spaceNum; j++) {
System.out.print(" ");
}
//numbers
int numbers = lines - spaceNum;
for (int j = numbers; j > 0; j--) {
System.out.print(" " + j);
}
System.out.println();
}
}
}
开始k=i
然后递减它直到k>=1
。
for(int k= i; k >=1 ; k--)
System.out.print(k+" ");
output
1
2 1
3 2 1
4 3 2 1
5 4 3 2 1
6 5 4 3 2 1
这是一种方法。 它使用String.repeat()
填充前导空格。
int rows = 6;
for (int i = 1; i <= rows; i++) {
System.out.print(" ".repeat(rows-i));
for (int k = i; k > 0; k--) {
System.out.print(k+ " ");
}
System.out.println();
}
印刷
1
2 1
3 2 1
4 3 2 1
5 4 3 2 1
6 5 4 3 2 1
如果您不想使用String.repeat()
则使用循环。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.