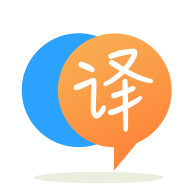
[英]Pass data from view to controller using AJAX in C# ASP.NET Core MVC
[英]ASP.Net Mvc How to pass data from View into Controller with a Button using Ajax
我有一个按钮,它从 Model 获取数据并将其传递给 Ajax function。 然后,这个 function 应该调用 controller,但它不会调用 controller 上的断点。
参数取自 Model 的按钮(均为字符串):
<button class="btn btn-primary btn-lg active" onclick="PassHwData(@obj.Name,@obj.HomeWorldBonus)" >Choose @obj.Name</button>
Ajax function:
<script>
function PassHwData(name, hwBonus)
{
$.ajax({
url: '@Url.Action("Create", "HomeWorld")',
type: "POST",
data: {'name' : name, 'hwBonus' : hwBonus}
datatype: "text",
success: function(name, hwBonus)
{
document.getElementById('success').innerHTML += success {name}{hwBonus};
}
})
}
</script>
<div id=success>
success:
</div>
Controller(还有其他方法,但我在这里省略了):
using DarkHeresy.Application.Services.Interfaces;
using Microsoft.AspNetCore.Authorization;
using Microsoft.AspNetCore.Mvc;
namespace DarkHeresy.Web.Controllers
{
[Authorize]
public class HomeWorldController : Controller
{
private readonly IHomeWorldService _homeWorldService;
public HomeWorldController(IHomeWorldService homeWorldService)
{
_homeWorldService = homeWorldService;
}
[HttpPost]
public async Task<IActionResult> Create(string name, string hwBonus)
{
return View(await _homeWorldService.UpdateCharacterList()); //this will have implementation later
}
}
}
我还应该补充一点,我正在使用 Asp.Net Core MVC 并使用 Clean Architecture,并且在这两方面都非常新。
您可以使用这种替代方式通过AJAX
将数据发送到您的Controller
方法:
HTML:
<button class="btn btn-primary btn-lg active" onclick="PassHwData('@obj.Name','@obj.HomeWorldBonus')" >Choose @obj.Name</button>
AJAX:
<script>
function PassHwData(name, hwBonus)
{
console.log(name);
console.log(hwBonus);
var json = {
name : name,
hwBonus : hwBonus
};
$.ajax({
type: "POST",
url: "@Url.Action("Create", "HomeWorld")",
dataType: "json",
data: {"json": JSON.stringify(json)},
success: function(name, hwBonus)
{
document.getElementById('success').innerHTML += success {name}{hwBonus};
}
})
}
</script>
<div id="success">
success:
</div>
Controller 方法:
using DarkHeresy.Application.Services.Interfaces;
using Microsoft.AspNetCore.Authorization;
using Microsoft.AspNetCore.Mvc;
using System.Text.Json; //For .NET CORE
namespace DarkHeresy.Web.Controllers
{
[Authorize]
public class HomeWorldController : Controller
{
private readonly IHomeWorldService _homeWorldService;
public HomeWorldController(IHomeWorldService homeWorldService)
{
_homeWorldService = homeWorldService;
}
[HttpPost]
public async Task<IActionResult> Create(string json)
{
var jsondata = JsonSerializer.Deserialize<dynamic>(json);
//Get your variables here from AJAX call
var name = jsondata["name"];
var hwBonus = jsondata["hwBonus"];
return View(await _homeWorldService.UpdateCharacterList()); //this will have implementation later
}
}
}
将 ajax 的数据类型更改为“json”
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.