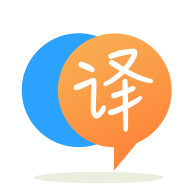
[英]how do i import and use promise function on my react component?
[英]how do I use "this" on an component function in react?
我需要从Animal
组件调用this.getFact
,但是使用this
会引发TypeError: this is undefined
import './App.css';
import React from 'react';
class App extends React.Component {
constructor(props) {
super(props);
this.state = {
fact: '',
}
}
getFact(endpoint) {
fetch(endpoint)
.then(response => response.json())
.then(data => {
this.setState({
fact: data.text,
});
})
}
Animal(props) {
return (
<div>
<h1>{props.name}</h1>
<button onClick={() => this.getFact(props.endpoint)}>get a {props.name.toLowerCase()} fact</button>
</div>
)
}
render() {
return (
<div className="App">
<div>
<p>{this.state.fact}</p>
</div>
<div className="animals">
<this.Animal name="Dog" endpoint="https://some-random-api.ml/facts/dog" />
<this.Animal name="Cat" endpoint="https://some-random-api.ml/facts/dog" />
<this.Animal name="Bird" endpoint="https://some-random-api.ml/facts/dog" />
<this.Animal name="Otter" endpoint="https://some-random-api.ml/facts/dog" />
</div>
</div>
);
}
}
export default App;
您需要绑定两个函数(getFact 和 Animal)才能在 Animal function 中使用它。 在您的构造函数中执行以下操作:
constructor(props) {
super(props);
this.state = {
fact: ""
};
// Here you will bind the functions so they can be callable with this
this.getFact = this.getFact.bind(this);
this.Animal = this.Animal.bind(this);
}
这将解决问题,但我建议将 Animal 组件移到 class 之外,并将 getFact 作为道具传递。 您仍然需要绑定 getFact function,但老实说,它会更加反应,并且从长远来看更易于维护。 像这样的东西
function Animal(props) {
return (
<div>
<h1>{props.name}</h1>
<button onClick={() => props.getFact(props.endpoint)}>
get a {props.name.toLowerCase()} fact
</button>
</div>
);
}
class AppReactWay extends React.Component {
constructor(props) {
super(props);
this.state = {
fact: ""
};
this.getFact = this.getFact.bind(this);
}
// Same code as you have
render() {
return (
<div className="App">
<div>
<p>{this.state.fact}</p>
</div>
<div className="animals">
<Animal
name="Dog"
getFact={this.getFact}
endpoint="https://some-random-api.ml/facts/dog"
/>
</div>
</div>
);
}
}
export default App;
同样在检查 API 响应后,您需要修改 getFact function,不是 data.text,而是 data.fact。
getFact(endpoint) {
fetch(endpoint)
.then((response) => response.json())
.then(({ fact }) => {
this.setState({
fact
});
});
}
这是一个包含两个示例的工作沙箱。
更好的方法是直接为每只动物调用{this.Animal({ name: 'Dog', endpoint: 'https://some-random-api.ml/facts/dog'})}
等等。
最好的方法是让Animal
拥有它自己的组件并将getFact
function 作为参数传递,不要忘记在这种情况下你需要绑定它,否则它会失去这个上下文。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.