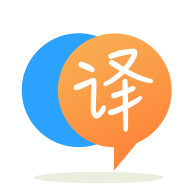
[英]How to sort a HashMap in descending order (based on the value) and keep the lexigraphy order (based on key) at the same time?
[英]Sort HashMap descending by value and ascending by key at the same time
考虑以下 hashmap:
HashMap<String, Double> cityScoreMap = new HashMap<>();
具有诸如
CityB, 5.0
CityC, 10.0
CityA, 5.0
我需要按值对 hashmap 进行降序排序,但如果值相等,则按键升序排序:
CityC, 10.0 (highest value)
CityA, 5.0 (comes before CityB, because A < B)
CityB, 5.0 (comes after CityA)
到目前为止,尝试按键分别排序,然后按值排序,但我不相信这种方法。 除了创建更多的哈希图之外,还有什么好的方法可以做到这一点?
实现这种 Map 的最简单方法是使用 stream。 你必须实现一个比较器。
Map<String, Double> cityScoreMap = new HashMap<>();
cityScoreMap.put("CityB", 5.0);
cityScoreMap.put("CityC", 10.0);
cityScoreMap.put("CityA", 5.0);
Comparator<Map.Entry<String, Double>> descendingValueAscendingKeyComparator = (entry1, entry2) -> {
//natural ordering of numbers is ascending, so comparing entry2 value to entry1 value makes for descending order
int cmp = entry2.getValue().compareTo(entry1.getValue());
if (cmp == 0) {
//if comparison result is zero, that means values are equal, so we are comparing keys
//we are comparing entry1 to entry2 keys for ascending order, which is natural for string
return entry1.getKey().compareTo(entry2.getKey());
}
return cmp;
};
cityScoreMap.entrySet()//get the set of entries in the map
.stream()//stream the entries
.sorted(descendingValueAscendingKeyComparator)//sort the entries with the supplied comparator
.forEach(entry -> System.out.println(entry.getKey() + ", " + entry.getValue()));//print to verify ordering
比较器定义元素的顺序,这可能与它们的自然顺序不同。 此比较器定义 map 中条目(键值对)的顺序 - 首先按值降序,然后按键升序。 这里的条目只是打印出来的,所以你可以看到排序,但你也可以将它们存储在另一个结构中,这样可以保持它们的顺序——例如 LinkedHashMap,无论你的实际需要是什么。
您应该从 javadoc 开始阅读Comparator和Comparable接口。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.