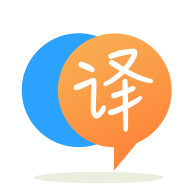
[英]How to add specific key values from one object to another array of objects, for each object
[英]How does one, from a list of objects, group and collect just specific values which are explicitly referred to, each by its key?
我有一个像
const dd = [{
keyone: "test",
two: "you",
three: 'op',
}, {
keyone: "youuuu",
two: "ttt",
three: 'op',
}, {
keyone: "operation",
two: "test",
three: 'op',
}];
我希望能够将 keyone 和两个拉出到 object 中,如下所示
const obj = { keyone: ['test', 'youuuu', 'operation'], two: ['you', 'ttt', 'test']}
我已经使用两张地图并将它们组合起来实现了这一点,但如果可能的话,我只想使用一个循环。
编辑:
我目前正在使用解构来提取值:
const mapOne = dd.map(({ keyone }) => keyone);
const mapTwo = dd.map(({ two }) => two);
const test = {
keyone: mapOne,
two: mapTwo,
};
你可以这样做:
const dd = [ { keyone: "test", two: "you", three: 'op' }, { keyone: "youuuu", two: "ttt", three: 'op' }, { keyone: "operation", two: "test", three: 'op' }]; const result = dd.reduce((prev, acc, arr ) => { if (acc['keyone']) { prev['keyone'].push(acc['keyone']) } if (acc['two']) { prev['two'].push(acc['two']) } return prev; }, {keyone: [], two: []}); console.log(result);
您可以使用reduce对数组进行一次迭代,并在每次迭代中通过Object.keys和forEach对 object 进行迭代:
const data = [ { keyone: "test", two: "you", three: 'op' }, { keyone: "youuuu", two: "ttt", three: 'op' }, { keyone: "operation", two: "test", three: 'op' }]; const targetKeys = ['keyone', 'two'] const result = data.reduce((acc, obj)=> { Object.keys(obj).forEach(k => { if(targetKeys.includes(k)) (acc[k] = acc[k] || []).push(obj[k]) }); return acc; }, {}); console.log(result);
您可以获取一组键并将值组合在一起。
const data = [{ keyone: "test", two: "you", three: 'op' }, { keyone: "youuuu", two: "ttt", three: 'op' }, { keyone: "operation", two: "test", three: 'op' }], keys = ['keyone', 'two'], grouped = data.reduce((r, o) => { keys.forEach(k => (r[k]??= []).push(o[k])); return r; }, {}); console.log(grouped);
一个通用实现,因此可重用和可配置的 function,它完全符合 OP 的要求,将接近下一个提供的示例代码......
function groupAndCollectSpecifcEntriesOnly(collector, item) { const { keyList, result } = collector; keyList.forEach(key => { if (item.hasOwnProperty(key)) { (result[key]??= []).push(item[key]) } }); return collector; } const sampleData = [{ keyone: "test", two: "you", three: 'op', }, { keyone: "youuuu", two: "ttt", three: 'op', }, { keyone: "operation", two: "test", three: 'op', }]; console.log( sampleData.reduce(groupAndCollectSpecifcEntriesOnly, { keyList: ['keyone', 'two'], result: {}, }).result ); console.log( sampleData.reduce(groupAndCollectSpecifcEntriesOnly, { keyList: ['three', 'keyone'], result: {}, }).result );
.as-console-wrapper { min-height: 100%;important: top; 0; }
const dd = [{ keyone: "test", two: "you", three: 'op' }, { keyone: "youuuu", two: "ttt", three: 'op' }, { keyone: "operation", two: "test", three: 'op' } ]; const pullOut = (...keys) => { return keys.map(key => { const val = dd.reduce((pV, cV, cI) => { pV.push(cV[key]); return pV; }, []); return { [key]: val }; }); }; console.log(pullOut('keyone', 'two'));
你要求一个循环:
const test = { keyone:[], two:[] }
dd.map( obj => {
if ( obj.hasOwnProperty( "keyone" )){
test[ "keyone" ].push( obj[ "keyone" ])
}
if ( obj.hasOwnProperty( "two" )){
test[ "two" ].push( obj[ "two" ])
}
})
我对 JavaScript 相当陌生,也许我的解决方案不是最好的。 我创建了一个 function,您可以在其中添加要从中获取信息的键的数量作为可选参数。 我假设您不知道键的名称。
const dd = [
{
keyone: "test",
two: "you",
three: "op",
},
{
keyone: "youuuu",
two: "ttt",
three: "op",
},
{
keyone: "operation",
two: "test",
three: "op",
},
];
getterInformation(dd);
function getterInformation(object, keyCount = Object.keys(object).length) {
let objectWithDesiredKey = {};
for (let index = 0; index < keyCount; index++) {
objectWithDesiredKey[`${Object.keys(object[0])[index]}`] = [];
}
object.forEach((items) => {
for (const key in items) {
if (key in objectWithDesiredKey) {
objectWithDesiredKey[key].push(items[key]);
}
}
});
console.log(objectWithDesiredKey);
}
你要求一个循环,但我免费给你三个;-)
function objReducer( arrayOfObjects, ...keys ) { const result = {} keys.forEach( key => { const arrayOfKeyValues = [] result[key] = arrayOfKeyValues }) arrayOfObjects.forEach( obj => { keys.forEach( key => { if ( obj.hasOwnProperty( key )) { result[key].push( obj[key] ) } }) }) return result } const dd = [{ keyone: "test", two: "you", three: 'op' }, { keyone: "youuuu", two: "ttt", three: 'op' }, { keyone: "operation", two: "test", three: 'op' } ] console.log( objReducer( dd, 'keyone', 'two' ))
我认为这是您正在寻找的答案:
(使用object 解构从每个 object 中提取“keyone”和“two”并将它们push
送到同一个map
循环中)
const data = [ { keyone: "test", two: "you", three: 'op' }, { keyone: "youuuu", two: "ttt", three: 'op' }, { keyone: "operation", two: "test", three: 'op' }]; const test = { keyone:[], two:[] } data.map(({ keyone, two }) => { test.keyone.push( keyone ); test.two.push( two ) }) console.log( test )
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.