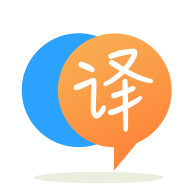
[英]Convert array of objects with string property to an array of strings in c#
[英]Convert String into Array of Strings in C
我正在尝试将一串按字母顺序排序的单词char *str = "a/apple/arm/basket/bread/car/camp/element/..."
分成按字母顺序排列的字符串数组,如下所示:
arr[0] = "a/apple/arm"
arr[1] = "basket/bread"
arr[2] = "car/camp"
arr[3] = ""
arr[4] = "element"
...
我对 C 不是很熟练,所以我的方法是声明:
char arr[26][100];
char curr_letter = "a";
然后遍历字符串中的每个字符以查找“/”,然后是 char,= curr_letter。 然后将 substring strcpy 到正确的位置。
我不确定我的方法是否很好,更不用说如何正确实施了。 任何帮助将不胜感激!
所以我们基本上循环遍历字符串,检查是否找到了“拆分字符”,还检查了没有找到下一个字符“curr_letter”。
我们跟踪消耗的长度,当前长度(用于 memcpy 稍后将当前字符串复制到数组中)。
当我们找到一个 position 可以将当前字符串添加到数组中时,我们分配空间并将字符串复制到它作为数组中的下一个元素。 我们还将 current_length 添加到消费中,并且 current_length 被重置。
我们使用due_to_end
来确定当前字符串中是否有/
,并相应地删除它。
尝试:
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
int main() {
char *str = "a/apple/arm/basket/bread/car/camp/element/...";
char split_char = '/';
char nosplit_char = 'a';
char **array = NULL;
int num_elts = 0;
// read all the characters one by one, and add to array if
// your condition is met, or if the string ends
int current_length = 0; // holds the current length of the element to be added
int consumed = 0; // holds how much we already added to the array
for (int i = 0; i < strlen(str); i++) { // loop through string
current_length++; // increment first
int due_to_end = 0;
if ( ( str[i] == split_char // check if split character found
&& ( i != (strlen(str) - 1) // check if its not the end of the string, so when we check for the next character, we don't overflow
&& str[i + 1] != nosplit_char ) ) // check if the next char is not the curr_letter(nosplit_char)
|| (i == strlen(str) - 1 && (due_to_end = 1))) { // **OR**, check if end of string
array = realloc(array, (num_elts + 1) * sizeof(char *)); // allocate space in the array
array[num_elts] = calloc(current_length + 1, sizeof(char)); // allocate space for the string
memcpy(array[num_elts++], str + consumed, (due_to_end == 0 ? current_length - 1 : current_length)); // copy the string to the current array offset's allocated memory, and remove 1 character (slash) if this is not the end of the string
consumed += current_length; // add what we consumed right now
current_length = 0; // reset current_length
}
}
for (int i = 0; i < num_elts; i++) { // loop through all the elements for overview
printf("%s\n", array[i]);
}
}
是的,原则上,您在问题中指定的方法似乎不错。 但是,我看到以下问题:
使用strcpy
将需要一个以 null 结尾的源字符串。 这意味着如果你想使用strcpy
,你必须用 null 字符覆盖/
。 如果您不想通过在其中写入 null 字符来修改源字符串,那么另一种方法是使用 function memcpy
而不是strcpy
。 这样,您可以指定要复制的确切字符数,并且不需要源字符串具有 null 终止字符。 但是,这也意味着您必须以某种方式计算要复制的字符数。
另一方面,您可以不使用strcpy
或memcpy
,而是一次将一个字符从str
复制到arr[0]
,直到遇到下一个字母,然后一次将一个字符从str
复制到arr[1]
等。 该解决方案可能更简单。
根据家庭作业问题的社区指南,我目前不会为您的问题提供完整的解决方案。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.