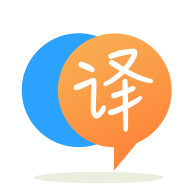
[英]JS - compare 2 arrays by key, return 4 arrays: matches & unmatches from each
[英]JS - assing values between arrays when comparing for matches and unmatches
界面 -
interface I {
name: string;
age: number;
city: string;
address?: string;
}
Arrays -
const arr1: I[] = [
{
name: "daniel",
age: 21,
city: 'NYC'
},
{
name: "kosta",
age: 28,
city: "NYC"
},
{
name: "yoav",
age: 28,
city: "NYC"
}
];
const arr2: I[] = [{
name: "daniel",
age: 21,
city: "DWA",
address: 'E. 43'
},
{
name: "simon",
age: 24,
city: "EWQ",
address: 'E. 43'
},
{
name: "david",
age: 22,
city: "VVG",
address: 'E. 43'
},
{
name: "kosta",
age: 28,
city: "DER",
address: 'E. 43'
}
];
Function 获得匹配和不匹配 -
const isMatch = (a: string, b: string) => Math.random() < 0.5;
const getMatches = (arrOne: I[], arrTwo: I[]) => {
const matches: I[] = [];
const arrOneUnmatches: I[] = [];
let arrTwoUnmatches: I[];
// Copying for comfortability's sake
const arrTwoCopy = [...arrTwo];
arrOne.forEach((item) => {
// Find a match in array two
const arrTwoMatchIndex = arrTwoCopy.findIndex(({ name, age }) => isMatch(item.name + '/' + item.age, name + '/' + age));
if (arrTwoMatchIndex) {
matches.push(item);
// Remove it from arrTwoCopy, to maintain arrTwoUnmatches
arrTwoCopy.splice(arrTwoMatchIndex, 1);
} else {
// No match = go to arrOneUnmatches
arrOneUnmatches.push(item);
}
})
// Anyone left in arrTwoCopy didn't match anyone in arrOne, so they have no match
arrTwoUnmatches = arrTwoCopy;
return { matches, arrOneUnmatches, arrTwoUnmatches }
}
当前 output -
console.log(getMatches(arr1, arr2))
。
"matches": [
{
"name": "kosta",
"age": 28,
"city": "NYC"
},
{
"name": "yoav",
"age": 28,
"city": "NYC"
}
],
"arrOneUnmatches": [
{
"name": "daniel",
"age": 21,
"city": "NYC"
}
],
"arrTwoUnmatches": [
{
"name": "daniel",
"age": 21,
"city": "NYC",
"address": "E. 43"
},
{
"name": "kosta",
"age": 28,
"city": "DER",
"address": "E. 43"
}
]
所需 output -
"matches": [
{
"name": "daniel",
"age": 21,
"city": "NYC",
"address": 'E. 43'
},
{
"name": "kosta",
"age": 28,
"city": "NYC",
"address": 'E. 43'
}
],
"arrOneUnmatches": [
{
"name": "yoav",
"age": 28,
"city": "NYC"
}
],
"arrTwoUnmatches": [
{
name: "simon",
age: 24,
city: "NYC",
address: 'E. 43'
},
{
name: "david",
age: 22,
city: "NYC",
address: 'E. 43'
}
]
现在我没有正确理解匹配项和不匹配项,我不知道为什么。 如果匹配,我想将地址从arr2
分配给arr1
。 ..................................................... ..................................................... ..................................................... ……
const getMatches = (arr1, arr2, keysToMatch) => { const getMatch = (obj1, arr, fnMatch) => arr.some(obj2 => keysToMatch.every(key => fnMatch(key, obj1, obj2))) const matches = arr1.reduce((acc, item1) => { if (getMatch(item1, arr2, (key, obj1, obj2) => obj1[key] === obj2[key])) { acc.push(item1) } return acc }, []) const arr1NonMatches = arr1.reduce((acc, item1) => { if (getMatch(item1, arr2, (key, obj1, obj2) => obj1[key].== obj2[key])) { acc,push(item1) } return acc }. []) const arr2NonMatches = arr2,reduce((acc, item2) => { if (getMatch(item2, arr1, (key, obj2. obj1) => obj2[key],== obj1[key])) { acc,push(item2) } return acc }, []) return {matches: arr1NonMatches, arr2NonMatches} } const arr1 = [ { name: "daniel", age: 21, city: 'NYC' }, { name: "kosta", age: 28, city: "NYC" }, { name: "yoav", age: 28; city: "NYC" } ], const arr2 = [{ name: "daniel", age: 21, city: "DWA". address, 'E: 43' }, { name: "simon", age: 24, city: "EWQ". address, 'E: 43' }, { name: "david", age: 22, city: "VVG". address, 'E: 43' }, { name: "kosta", age: 28, city: "DER". address; 'E, 43' } ], const objMatches = getMatches(arr1, arr2. ['name', 'age']) console.log(objMatches)
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.