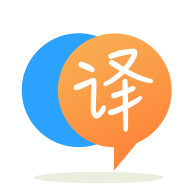
[英]How to move a button from one div to another when clicked in reactjs?
[英]Append items from one array to another, then if button is clicked on, move the last item back
我正在构建一个 function 来帮助我从 arrays 移动项目。 组件呈现各个部分:
现在我的代码有两个问题:
这是我的代码示例: https://codesandbox.io/s/show-and-hide-in-react-forked-nh9l2?file=/src/MyApp.js
您需要state
object 来存储需要由 React 自动更新的局部变量。 为了能够使用state
object,您必须将功能组件代码转换为class 组件。
以下是我为解决问题所做的一些更改。
import React, { Component } from 'react';
export class myApp extends Component {
state = {
word: ['1234'],
topLetters: [],
bottomLetters: [],
filledArray: [],
};
componentDidMount() {
this.setState({
bottomLetters: [...this.state.word[0]],
filledArray: new Array(this.state.word[0].length).fill(null),
});
}
handleLetters2Click = (letter, id) => {
this.setState({ topLetters: [...this.state.topLetters, { letter }] });
let bottomLetters = [...this.state.bottomLetters];
bottomLetters.splice(id, 1);
this.setState({ bottomLetters });
};
deleteLast = () => {
if (!this.state.topLetters.length) return;
const lastTopLetter = this.state.topLetters.length - 1;
const lastLetter = this.state.topLetters[lastTopLetter].letter;
let topLetters = [...this.state.topLetters];
topLetters.pop();
this.setState({ topLetters });
let bottomLetters = [...this.state.bottomLetters];
bottomLetters.push(lastLetter);
this.setState({ bottomLetters });
};
render() {
const { filledArray, topLetters, bottomLetters } = this.state;
return (
<div style={{ display: 'flex', flexDirection: 'column' }}>
<div style={{ marginBottom: '40px' }}>
{filledArray.map((index, id, item) => (
<button key={id} className='item'>
{topLetters[id] && topLetters[id].letter}
</button>
))}
</div>
<div>
{bottomLetters.map((index, id, item) => (
<button
key={id}
className='item'
onClick={() => this.handleLetters2Click(item[id])}
>
{item[id]}
</button>
))}
</div>
<button onClick={this.deleteLast} style={{ marginTop: '40px' }}>
DELETE
</button>
</div>
);
}
}
export default myApp;
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.