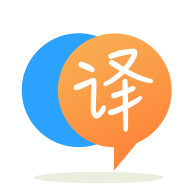
[英]How can I pass a FnMut closure to a function using a reference in Rust?
[英]How can I obtain the address of a closure in Rust?
let say_hello = || println!("hello");
如何显示闭包地址?
您可以使用原始指针转换:
fn main() {
let say_hello = || println!("hello");
let address = (&say_hello as *const _) as usize;
println!("{address}");
}
还使用std::ptr::addr_of
并再次投射:
let same_address = std::ptr::addr_of!(say_hello) as usize;
这个问题的答案实际上取决于您是指闭包 object 本身,还是闭包的调用 function。
例如,在这种情况下,闭包捕获一个变量:
let mut foo = 0;
let mut bar = || foo += 1;
因此, bar
必须在其中携带一个&mut foo
。
因此,实际运行的代码地址与闭包地址 object 不同。
要具体说明这种区别,请检查以下示例:
fn foo() {}
let bar = foo; // `bar` itself is the address of the code for foo.
println!("{:?}", bar as usize); // Prints the address of the code of foo.
println!("{:?}", &bar as *const _ as usize); // Prints the address of `bar`
第二行基本上就是@Netwave 上面的回答。
如果您正在寻找第一种情况,那么这不一定总是可能的。
如果您的闭包捕获项目,则无法将其转换为稳定 rust 中代码的地址,因为这需要您有权访问它的FnOnce
/ Fn
/ FnMut
。 但是,如果您对不稳定的 rust 没问题,那么您可以这样做:
#![feature(unboxed_closures, fn_traits)]
fn main() {
let mut x = 0;
let mut foo = || x += 1;
print_addr(foo);
}
fn print_addr<F: FnMut()>(_: F) {
println!("{:?}", <F as std::ops::FnMut<()>>::call_mut as usize);
}
如果您的闭包没有捕获任何内容,那么获取地址的正确方法是将其转换为 function 指针,然后将其转换为数字:
fn main() {
let foo = || println!("Abc");
println!("{:?}", foo as fn() as usize);
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.