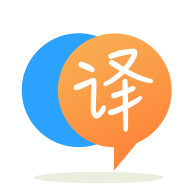
[英]nextInt() method of Scanner class does not ask me for input again in while loop?
[英]Scanner inside method dont ask for input
我要求用户插入一个item name with extension ".txt"
的项目名称,如果该item name
与existing itemName.txt
对应,则用户需要在他想要“传输”它的地方插入location name with extension ".txt"
。 如果一切正常,那么itemName.txt
中的内容将被写入locationName.txt
我的主要是这样的:
package victor;
import java.io.*;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Scanner;
public class Main extends Item {
public Main(String location, Integer length, Integer height, Integer depth) {
super(location, length, height, depth);
}
public static void main(String[] args) throws IOException {
List<Item> itemList = new ArrayList<>();
List<Location> locationList = new ArrayList<>();
System.out.println("Welcome to create new:");
System.out.println("Please follow the steps:");
HashMap<Integer, String> menu = new HashMap<>();
menu.put(1, "Create Location");
menu.put(2, "Create Item");
menu.put(3, "Transfer to location");
menu.forEach((key, value) -> System.out.println(key + "- " + value));
int user_choice;
Scanner userInputMenu = new Scanner(System.in);
user_choice = userInputMenu.nextInt();
switch (user_choice) {
case 1:
createLocationPlusFile(locationList);
case 2:
createItemPlusFile(itemList);
case 3:
transferItemToLocation(itemList, locationList);
}
}
}
当用户从 menu- 3
输入时,程序正在关闭Process finished with exit code 0
。
这是我的项目 Class 和我写的方法:
package victor;
import java.io.*;
import java.util.List;
import java.util.Scanner;
public class Item extends Location {
private String itemName;
private Integer kg;
private Integer length;
private Integer height;
private Integer depth;
public Item(String itemName, Integer kg, Integer length, Integer height, Integer depth) {
super();
this.itemName = itemName;
this.kg = kg;
this.length = length;
this.height = height;
this.depth = depth;
}
public Item() {
}
public Item(String location, Integer length, Integer height, Integer depth) {
super();
}
public String getItemName() {
return itemName;
}
public void setItemName(String itemName) {
this.itemName = itemName;
}
public Integer getKg() {
return kg;
}
public void setKg(Integer kg) {
this.kg = kg;
}
public Integer getLength() {
return length;
}
public void setLength(Integer length) {
this.length = length;
}
public Integer getHeight() {
return height;
}
public void setHeight(Integer height) {
this.height = height;
}
public Integer getDepth() {
return depth;
}
public void setDepth(Integer depth) {
this.depth = depth;
}
@Override
public String toString() {
return "Item{" +
"itemName='" + itemName + '\'' +
", kg=" + kg +
", length=" + length +
", height=" + height +
", depth=" + depth +
'}';
}
public static void createItemPlusFile(List<Item> itemList) throws NullPointerException, IOException {
int defaultLength = 80;
int defaultHeight = 205;
int defaultDepth = 120;
int defaultKg = 3500;
Scanner in1 = new Scanner(System.in);
System.out.println("Enter Item Name: ");
String itemName = in1.nextLine();
System.out.println("New Item created successfully!" + "\n" + itemName);
int inLength;
Scanner in2 = new Scanner(System.in);
System.out.println("Enter Item height: ");
int itemLength = in2.nextInt();
if (itemLength > defaultLength) {
System.out.println("Height that you added is to big, please use a height of max 205");
inLength = in2.nextInt();
} else {
System.out.println("Height inserted correctly! ");
}
int inHeight;
Scanner in3 = new Scanner(System.in);
System.out.println("Enter Item height: ");
int itemHeight = in3.nextInt();
if (itemHeight > defaultHeight) {
System.out.println("Height that you added is to big, please use a height of max 205");
inHeight = in3.nextInt();
} else {
System.out.println("Height inserted correctly! ");
}
int inDepth;
Scanner in4 = new Scanner(System.in);
System.out.println("Enter Item depth: ");
int itemDepth = in4.nextInt();
if (itemDepth > defaultDepth) {
System.out.println("Depth that you added is to big, please use a depth of max 120");
inDepth = in4.nextInt();
} else {
System.out.println("Depth inserted correctly! ");
}
int inKg;
Scanner in5 = new Scanner(System.in);
System.out.println("Enter Item kg: ");
int itemKg = in4.nextInt();
if (itemKg > defaultKg) {
System.out.println("kg that you added is to big, please use a depth of max 3500");
inDepth = in4.nextInt();
} else {
System.out.println("Kg inserted correctly! ");
}
Item item = new Item(itemName, itemKg, itemLength, itemHeight, itemDepth);
File file = new File(item.getItemName() + "CUSCAS001GRY-uk.txt");
if (!file.exists()) {
file.createNewFile();
}
FileWriter fileWriter = new FileWriter(item.getItemName() + "CUSCAS001GRY-uk.txt", true);
fileWriter.write(item + "\n");
fileWriter.close();
}
//this method I wrote, I expect to ask user to input the item and if item with this name exist then he insert the location where he want to "transfer" it .
public static void transferItemToLocation(List<Item> itemList, List<Location> locationList) throws IOException {
for (Item item : itemList) {
for (Location location : locationList) {
Scanner inputItemTransfer = new Scanner(System.in);
System.out.println("Insert the item you want to transfer + .txt extension!");
inputItemTransfer.nextLine();
if (inputItemTransfer.nextLine()
.equalsIgnoreCase(item + ".txt")) {
Scanner inputTransferToLocation = new Scanner(System.in);
System.out.println("Insert the location where you want to transfer the item");
inputTransferToLocation.nextLine();
if (inputTransferToLocation.nextLine()
.equalsIgnoreCase(String.valueOf(location))) {
BufferedReader bufferedReader = new BufferedReader(new FileReader(String.valueOf(inputItemTransfer)));
FileWriter fileWriter = new FileWriter(inputTransferToLocation + ".txt", true);
BufferedWriter outputStream = new BufferedWriter(fileWriter);
String str;
while ((str = bufferedReader.readLine()) != null) {
outputStream.write(str + "\n");
}
outputStream.close();
}
}
}
}
}
}
我假设我对扫描仪做错了什么。 非常感谢任何帮助/批评
您不需要为用户需要输入数据的每个提示创建扫描仪 object。 整个应用程序的一个扫描仪 object 就可以了。 只需在Main class 中将 object 声明为public static即可,例如:
public class Main extends Item {
// Declare a Scanner object that can potentially be used anywhere.
public static Scanner userInput = new Scanner(System.in).useDelimiter("\\R");
public Main(String location, Integer length, Integer height, Integer depth) {
super(location, length, height, depth);
}
public static void main(String[] args) throws IOException {
// ... main() method code here ...
}
}
然后在任何地方提示用户并需要使用 Scanner 方法获取输入,请使用Main.userInput。 相反,例如:
user_choice = Main.userInput.nextInt();
或者:
String itemName = Main.userInput.nextLine();
当使用Scanner#nextInt() (或任何 Scanner#nextXXX 方法)并且 Scanner# nextLine () 提示将直接跟随时,您很可能需要使用从 Scanner#nextInt( ) 利用。 nextInt()
方法不像nextline()
方法那样使用换行符,因此似乎跳过了使用nextLine()
方法的提示。 在这种情况下,你会想要这样做:
user_choice = Main.userInput.nextInt();
Main.userInput.nextLine();
一个好的经验法则是:
如果您使用Scanner#nextLine()方法,则将其用于其他任何事情,根本不要使用它。 请改用next()
和nextXXX()
方法。
如果您按上面所示创建 Scanner(使用useDelimiter("\\R")
方法),则Scanner#next()
方法将返回类似于Scanner#nextLine()
方法返回的内容。
目前,由于您使用 Scanner 方法的方式,您的transferItemToLocation()
方法对方法中的每个提示进行双重提示。
public static void transferItemToLocation(List<Item> itemList, List<Location> locationList) throws IOException {
for (Item item : itemList) {
for (Location location : locationList) {
Scanner inputItemTransfer = new Scanner(System.in);
System.out.println("Insert the item you want to transfer + .txt extension!");
inputItemTransfer.nextLine();
if (inputItemTransfer.nextLine()
.equalsIgnoreCase(item + ".txt")) {
Scanner inputTransferToLocation = new Scanner(System.in);
System.out.println("Insert the location where you want to transfer the item");
inputTransferToLocation.nextLine();
if (inputTransferToLocation.nextLine()
.equalsIgnoreCase(String.valueOf(location))) {
BufferedReader bufferedReader = new BufferedReader(new FileReader(String.valueOf(inputItemTransfer)));
FileWriter fileWriter = new FileWriter(inputTransferToLocation + ".txt", true);
BufferedWriter outputStream = new BufferedWriter(fileWriter);
String str;
while ((str = bufferedReader.readLine()) != null) {
outputStream.write(str + "\n");
}
outputStream.close();
}
}
}
}
}
我只能假设您为什么要这样做,因为您没有提供所有代码,这部分是为什么我在上面发布了很多关于 Scanner 的废话。 你在这里得到的东西不会像你想象的那样工作。 如前所述,摆脱所有这些 Scanner 对象并使用类似于Main class 中声明的内容(类似于本文开头显示的内容)。 声明名为inputItemTransfer和inputTransferToLocation的字符串变量。 将用户输入分别放入每个提示的这些变量中,并使用这些变量中包含的数据而不是扫描仪对象,例如:
public static void transferItemToLocation(List<Item> itemList, List<Location> locationList) throws IOException {
String inputItemTransfer;
String inputTransferToLocation;
for (Item item : itemList) {
for (Location location : locationList) {
System.out.println("Insert the item you want to transfer + .txt extension!");
inputItemTransfer = Main.userInput.nextLine();
if (inputItemTransfer.equalsIgnoreCase(item + ".txt")) {
System.out.println("Insert the location where you want to transfer the item");
inputTransferToLocation = Main.userInput.nextLine();
if (inputTransferToLocation.equalsIgnoreCase(String.valueOf(location))) {
BufferedReader bufferedReader = new BufferedReader(new FileReader(String.valueOf(inputItemTransfer)));
FileWriter fileWriter = new FileWriter(inputTransferToLocation + ".txt", true);
BufferedWriter outputStream = new BufferedWriter(fileWriter);
String str;
while ((str = bufferedReader.readLine()) != null) {
outputStream.write(str + "\n");
}
outputStream.close();
}
}
}
}
}
这个方法现在是否正确地转到 function 真的超出了我的范围。 没有所有代码或至少没有最小的可重现示例,很难说。 我想一步一个脚印。
在一边:
正如评论中提到的那样,用户确实需要输入大量数据才能使用您的应用程序,这对某些人来说有点令人沮丧,而且实际上很难。 或许可以考虑使用更多基于菜单的系统,以减少进入要求并使使用体验更舒适。
祝你好运。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.