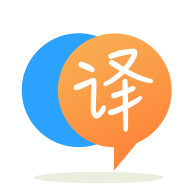
[英]How do i use regex in javascript to find three periods inside of a string?
[英]How do I use regex to replace periods in a string?
我正在使用 javascript。
我有一个字符串:
let x = ".175\" x 2.5\" x 144\""; // .175" x 2.5" x 144"
我想操纵字符串返回 3 个单独的变量
var thickness = 0.175
var width = 2.5
var length = 144
我需要将字符串中的粗细从 .175 更改为 0.175,这是我的尝试:
let x = ".175\" x 2.5\" x 144\"";
let regex = / /g;
let regex2 = /\./i;
let regex3 = /\"/g;
//Do the regex function
const regexFun = () => {
try {
console.log(x);
if (x.charAt(0) == "."){
const fun1 = x.replace(regex2, '0.');
console.log(fun1);
}
else{
const fun1 = x.replace(regex3, '');
console.log(fun1);
}
} catch (err) {
console.log(err.message)
}
}
在我给出的例子中,我可以使用 " 删除引号,但它似乎不起作用。我怎么能 go 关于这个?谢谢!
您可以将字符串的格式与命名的捕获组相匹配。
^(?<thickness>\d*\.?\d+)"\s+x\s+(?<width>\d*\.?\d+)"\s+x\s+(?<length>\d*\.?\d+)"$
模式匹配:
^
字符串开始(?<thickness>\d*\.?\d+)
组粗细匹配可选数字、可选点和 1+ 数字"\s+x\s+
Match "
和可选空白字符之间的x
字符(?<width>\d*\.?\d+)
具有相同数字模式的组宽度"\s+x\s+
匹配"
和x
字符(?<length>\d*\.?\d+)
具有相同数字模式的组长度"
字面匹配$
字符串结尾请参阅regex101 演示中的组值。
let x = ".175\" x 2.5\" x 144\""; const regex = /^(?<thickness>\d*\.?\d+)"\s+x\s+(?<width>\d*\.?\d+)"\s+x\s+(?<length>\d*\.?\d+)"$/; const m = x.match(regex); if (m) { const thickness = parseFloat(m.groups.thickness); const width = parseFloat(m.groups.width); const length = parseFloat(m.groups.length); console.log(thickness, width, length); }
使用这个正则表达式
(^|\D)(\.\d+)
( // Begin capture
^ // Beginning of line
| // Or
\D // Any non-digit
) // End capture
( // Begin capture
\. // Decimal point
\d+ // Number
) // End capture
替换为$10$2
$1 // Contents of the 1st capture
0 // Literal 0
$2 // Contents of the 2nd capture
let x = ".175\" x 2.5\" x 144\""; console.log(x.replace(/(^|\D)(\.\d+)/g, "$10$2"));
正如 Felix 评论的那样,最好的方法可能是先使用正则表达式提取数字,然后将它们解析为浮点数:
var x = ".175\" x 2.5\" x 144\""; //.175" x 2.5" x 144" let numbers = x.match(/(\d*\.)?\d+/g).map(n => parseFloat(n)) var thickness = numbers[0] var width = numbers[1] var length = numbers[2] console.log('thickness: ' + thickness) console.log('width: ' + width) console.log('length: ' + length)
尝试使用split()
和parseFloat
let x = ".175\" x 2.5\" x 144\""; const regexFun = () => { numbers = x.split('x').map(n=>parseFloat(n)) console.log(numbers) } regexFun()
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.