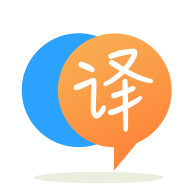
[英]How to reconcile Javascript with currying and function composition
[英]How to implement function composition in JavaScript?
以下是需要组合的三个函数,并为我们提供 output 30
:
const add = (a) => a + 10;
const mul = (a) => a * 10;
const divide = (a) => a / 5;
// How to implement `compositionFunction`?
compositionFunction(add, mul, divide)(5);
//=> 30
预期 output 是30
,因为:
5 + 10 = 15
15 * 10 = 150
150 / 5 = 30
像这样的东西
const add = (a) => a + 10; const mul = (a) => a * 10; const divide = (a) => a / 5; // How to use this function ----- const customComposeFn = (...f) => v => f.reduce((res, f) => f(res), v) console.log(customComposeFn(add, mul, divide)(5));
function组成有两种味道:
pipe
compose
这是一个递归实现,只是为了好玩:
这假设至少有两个函数要组成
const compose = (...fn) => {
const [[f, g], x] = [fn.slice(-2), fn.slice(0, -2)];
const h = a => f(g(a));
return x.length ? compose(...x, h) : h;
}
const pipe = (...fn) => {
const [f, g, ...x] = fn;
const h = a => g(f(a));
return x.length ? pipe(h, ...x) : h;
}
咱们试试吧:
const foo = x => x + 'foo';
const bar = x => x + 'bar';
const baz = x => x + 'baz';
pipe(foo, bar, baz)('');
//=> 'foobarbaz'
compose(foo, bar, baz)('');
//=> 'bazbarfoo'
const add = (a) => a + 10; const mul = (a) => a * 10; const divide = (a) => a / 5; const customComposeFn = (...fn) => { return function (arg) { if (fn.length > 0) { const output = fn[0](arg); return customComposeFn(...fn.splice(1))(output); } else { return arg; } }; }; const res = customComposeFn(add, mul, divide)(5); console.log(`res`, res);
这是我会做的:
function customComposeFn(...funcs) { return function(arg) { let f, res = arg; while (f = funcs.shift()) { res = f(res) } return res; } } const add = a => a + 10; const mul = a => a * 10; const divide = a => a / 5; // How to use this function ----- console.log(customComposeFn(add, mul, divide)(5));
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.