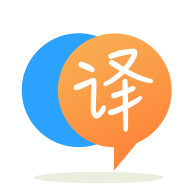
[英]C++ How to convert a big integer in std::string form to byte/char-array/vector
[英]C++ std::any function that convert std::any of C char-array to string
#include <iostream>
#include <any>
#include <string>
#include <vector>
#include <map>
using namespace std;
string AnyPrint(const std::any &value)
{
cout << size_t(&value) << ", " << value.type().name() << " ";
if (auto x = std::any_cast<int>(&value)) {
return "int(" + std::to_string(*x) + ")";
}
if (auto x = std::any_cast<float>(&value)) {
return "float(" + std::to_string(*x) + ")";
}
if (auto x = std::any_cast<double>(&value)) {
return "double(" + std::to_string(*x) + ")";
}
if (auto x = std::any_cast<string>(&value)) {
return "string(\"" + (*x) + "\")";
}
if (auto x = std::any_cast<char*>(&value)) {
return string(*x);
}
}
int main()
{
int a = 1;
float b = 2;
double c = 3;
string d = "4";
char *e = "555";
cout << AnyPrint(a) << "\n";
cout << AnyPrint(b) << "\n";
cout << AnyPrint(c) << "\n";
cout << AnyPrint(d) << "\n";
cout << AnyPrint("555") << "\n";
cout << AnyPrint(e) << "\n";
return 0;
}
我正在尝试制作一个 function 将std::any
object 转换为字符串,因为可能的类型列表是硬编码的。 但是,当用户解析AnyPrint("555")
之类的原始字符串时会出现问题。 我使用Checking std::any's type without RTTI中的方法
当我运行程序时,我得到以下 output:
140722480985696, i int(1)
140722480985696, f float(2.000000)
140722480985696, d double(3.000000)
140722480985696, NSt7__cxx1112basic_stringIcSt11char_traitsIcESaIcEEE string("4")
140722480985696, PKc string("4")
140722480985696, Pc 555
如何处理std::any
原始字符串? 我不想写AnyPrint("555"s)
除非这是唯一的方法。
"555"
的类型是const char[4]
,它可能会衰减到const char*
。 您处理char*
,但不处理const char*
。
处理const char*
可以解决您的问题:
std::string AnyPrint(const std::any &value)
{
std::cout << size_t(&value) << ", " << value.type().name() << " ";
if (auto x = std::any_cast<int>(&value)) {
return "int(" + std::to_string(*x) + ")";
}
if (auto x = std::any_cast<float>(&value)) {
return "float(" + std::to_string(*x) + ")";
}
if (auto x = std::any_cast<double>(&value)) {
return "double(" + std::to_string(*x) + ")";
}
if (auto x = std::any_cast<std::string>(&value)) {
return "string(\"" + (*x) + "\")";
}
if (auto x = std::any_cast<const char*>(&value)) {
return *x;
}
return "other";
}
如评论中所述,像"555"
这样的字符串文字是(或衰减为) const char*
指针。 您的AnyPrint
function 不处理此类参数类型。
添加以下块可解决问题:
if (auto x = std::any_cast<const char*>(&value)) {
return string(*x);
}
另外,请注意行, char *e = "555";
在 C++ 中是非法的; 你需要const char *e = "555";
或者char e[] = "555";
; 使用后者将展示std::any_cast<T>
块中的char*
(使用AnyPrint(e)
)和const char*
(使用AnyPrint("555")
)类型之间的区别。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.