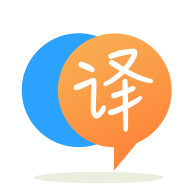
[英]C++: Elegant way to split string and stuff contents into std::vector
[英]c++ String from file to vector - more elegant way
我写了一个代码,我想在其中将几个字符串从文本文件传递到字符串向量。 目前我这样做:
using namespace std;
int main()
{
string list_name="LIST";
ifstream REF;
REF.open(list_name.c_str());
vector<string> titles;
for(auto i=0;;i++)
{
REF>>list_name;
if(list_name=="-1"){break;}
titles.push_back(list_name);
}
REF.close();
cout<<titles.size();
for(unsigned int i=0; i<titles.size(); i++)
{
cout<<endl<<titles[i];
}
它工作正常,我按预期得到了 output。 我担心的是,当将字符串从文件流传递到字符串 object 并将其分配给带有 push_back 的向量作为单独的步骤时,是否有更优雅的方法将字符串从文本文件直接传递到向量,从而避免此片段:
REF>>list_name;
if(list_name=="-1"){break;}
titles.push_back(list_name);
更优雅的算法方式
std::copy_if(std::istream_iterator<std::string>(REF),
std::istream_iterator<std::string>(),
std::back_inserter(titles),
[](const std::string& t) { return t != "-1"; });
如果您事先知道要读取的字符串数,则可以
using StringVector = std::vector<std::string>;
int main(int argc, const char* argv) {
constexpr size_t N = 4; // or however many strings you want...
StringVector data(N);
std::ifstream stream("foo.txt");
for (size_t i =0; (i < N) && stream; i++) {
stream >> data[i];
}
}
但这会不太灵活,并且实施"-1"
“终止符”约定会更加棘手。
如果那个"-1"
是一个真正的要求(与任意选择相反),并且如果你多次使用它,它可能会得到“抽象”的回报,你如何阅读这些字符串。 抽象通常以 function 的形式完成。
// compile with:
// clang++-13 -std=c++20 -g -O3 -o words words.cpp
#include <iostream>
#include <string>
#include <vector>
#include <sstream>
using StringVector = std::vector<std::string>;
std::istream& operator>> (std::istream& stream, StringVector& sv)
{
std::string word;
while (stream) {
stream >> word;
if (word == "-1")
return stream;
sv.push_back(word);
}
return stream;
}
std::ostream& operator<< (std::ostream& stream,
const StringVector& sv) {
for (const auto& s : sv) {
stream << s << std::endl;
}
return stream;
}
int main(int argc, const char* argv[]) {
std::string file_data{R"(word1 word2
word3
word4 -1)"};
std::istringstream stream(file_data);
StringVector data;
data.reserve(10);
stream >> data;
std::cout
<< "Number of strings loaded: "
<< data.size() << std::endl;
std::cout << data;
return 0;
}
上面的operator>>()
通常适用于流,因此它也适用于文件流。
顺便说一句:人们不喜欢"-1"
终结符方法的一个原因是性能。 如果你不断地向一个 vector 推送任意次数,那么随着 vector 的增长,需要重新分配 vector 的存储,这是可以避免的开销。 因此,通常人们会使用另一种文件格式,例如首先给出字符串的数量,然后给出字符串,这将允许:
size_t n;
stream >> n;
StringVector data;
data.reserve(n); // avoids "spurious reallocs as we load the strings"
for (size_t i = 0; i < n; i++) { ... }
其他答案可能太复杂或太复杂。
让我先对您的代码做一个小小的审查。 请在代码中查看我的评论:
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
using namespace std; // You should not open the full std namespace. Better to use full qualifiacation
int main()
{
string list_name = "LIST";
ifstream REF; // Here you coud directly use the construct ofr the istream, which will open the file for you
REF.open(list_name.c_str()); // No need to use c_str
vector<string> titles; // All variables should be initialized. Use {}
for (auto i = 0;; i++) // Endless loop. You could also write for(;;), but bad design
{
REF >> list_name;
if (list_name == "-1") { break; } // Break out of the endless loop. Bad design. Curly braces not needed
titles.push_back(list_name);
}
REF.close(); // No nbeed to close the file. With RAII, the destructor of the istream will close the file for you
cout << titles.size();
for (unsigned int i = 0; i < titles.size(); i++) // Better to use a range based for loop
{
cout << endl << titles[i]; // end not recommended. For cout`'\n' is beter, because it does not call flush unneccesarily.
}
}
您会看到许多需要改进的地方。
让我向您解释一些更重要的主题。
std::ifstreams
构造函数直接打开文件。bool
和!
std::ifstream
的运算符被覆盖。 所以可以做一个简单的测试std::ifstream
的析构函数将为您完成该操作。 如果您想读取文件直到 EOF(文件结尾)或任何其他条件,您可以简单地使用 while 循环并调用提取运算符>>
例如:
while (REF >> list_name) {
titles.push_back(list_name);
}
为什么这行得通? 提取运算符将始终返回对 stream 的引用及其调用。 因此,您可以想象在读取字符串后,while 将包含while (REF)
,因为 REF 是由(REF >> list_name
返回的。并且,如前所述,stream 的bool
运算符被覆盖并返回 state 的stream。如果有任何错误或 EOF,则if (REF)
将为假。
所以现在附加条件:与“-1”的比较可以很容易地添加到 while 语句中。
while ((REF >> list_name) and (list_name != "-1")) {
titles.push_back(list_name);
}
这是一个安全的操作,因为 boolean 快捷方式评估。 如果第一个条件已经为假,则不会评估第二个。
有了上面的所有知识,代码可以重构为:
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
int main() {
// Here our source data is stored
const std::string fileName{ "list.txt" };
// Open the file and check, if it could be opened
std::ifstream fileStream{ fileName };
if (fileStream) {
// Here we will store all titles that we read from the file
std::vector<std::string> titles{};
// Now read all data and store vit in our resulting vector
std::string tempTitle{};
while ((fileStream >> tempTitle) and (tempTitle != "-1"))
titles.push_back(tempTitle);
// For debug purposes. Show all titles on screen:
for (const std::string title : titles)
std::cout << '\n' << title;
}
else std::cerr << "\n*** Error: Could not open file '" << fileName << "'\n";
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.