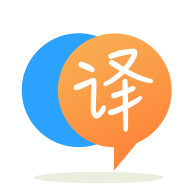
[英]How to display the div of same class from list by clicking next button?
[英]display the question to div using innerhtml from array by clicking next button
我正在制作一个有很多问题的抽认卡,我使用数组存储它们。 我做了随机的问题,但有时问题出现不止一个。 我将通过单击下一步按钮逐一显示问题。 此外,如果问题已完成,则应停止随机问题。
var btnNext = document.getElementById("next"); words = [{ question: "HTML stands for", answer: "HyperText Markup Language" }, { question: "What is a href?", answer: "Link location attribute" }, { question: "What is extensions used to save HTML pages?", answer: ".html" } ] btnNext.addEventListener('click', function() { var tempWords = []; if (tempWords.length === 0) { for (var i = 0; i < words.length; i++) { tempWords.push(words[i]); } } let index = Math.floor(Math.random() * tempWords.length); document.getElementById("question").innerHTML=tempWords[index]?.question; });
<div id="question"> <h3>Questions</h3> </div> <button id="next">Next</button>
有两种方法可以做你想做的事,我能想到
您可以使用不同的方法对数组进行洗牌,我已经按照这个堆栈溢出答案来做到这一点,这看起来是最简单的
然后,您可以每次使用 js shift
方法删除第一个问题,该方法从数组中删除第一个元素并将其返回
var btnNext = document.getElementById("next"); words = [{ question: "HTML stands for", answer: "HyperText Markup Language" }, { question: "What is a href?", answer: "Link location attribute" }, { question: "What is extensions used to save HTML pages?", answer: ".html" } ] const randomWords = words.sort(() => Math.random() - 0.5); btnNext.addEventListener('click', function() { if (randomWords.length > 0) { const question = randomWords.shift() document.getElementById("question").innerHTML = question.question; } });
<div id="question"> <h3>Questions</h3> </div> <button id="next">Next</button>
第二种方法与 js splice
function 一起使用,它从数组中删除元素并返回删除的元素(文档在这里)
要打印问题,您可以使用slice
方法访问 function:
const question = words.splice(index, 1)[0] // taking the first element because slice return an array
然后像您一样使用 InnerHTML 打印它
document.getElementById("question").innerHTML = question.question;
var btnNext = document.getElementById("next"); words = [{ question: "HTML stands for", answer: "HyperText Markup Language" }, { question: "What is a href?", answer: "Link location attribute" }, { question: "What is extensions used to save HTML pages?", answer: ".html" } ] btnNext.addEventListener('click', function() { if (words.length > 0) { var tempWords = []; if (tempWords.length === 0) { for (var i = 0; i < words.length; i++) { tempWords.push(i); } } let index = Math.floor(Math.random() * tempWords.length); const question = words.splice(index, 1)[0] document.getElementById("question").innerHTML = question.question; } });
<div id="question"> <h3>Questions</h3> </div> <button id="next">Next</button>
我的想法是:首先随机排列数组。 所以你的代码(JS部分)应该是这样的:
var btnNext = document.getElementById("next"),
tempwords = [{
question: "HTML stands for",
answer: "HyperText Markup Language"
},
{
question: "What is a href?",
answer: "Link location attribute"
},
{
question: "What is extensions used to save HTML pages?",
answer: ".html"
}
],
words = [],
num = 0;
while (tempWords.length !== 0) {
let index = Math.floor(Math.random() * tempWords.length);
words.push(tempWords[index]);
tempWords.splice(index, 1);
}
delete tempWords;
btnNext.addEventListener('click', function() {
if (num < words.length) {
let indexItems = words[num++];
}
// More code....
});
通过跟踪索引是否以前使用过它们。
const btn = document.getElementById("next");
var indexArray = [];
btn.onclick = function getNewQuestion() {
let tempWords = [
"What is the capital of India?",
'What is mean by "Lo siento"?',
'What is mean by "mesa"?',
'What is mean by "Para"?',
"What is mean by 'Bonito'?",
];
let index = getValidIndex(indexArray, tempWords.length);
console.log(tempWords[index]);
};
// function to get valid non-repeative index
function getValidIndex(indexArray, lengthOfTempWords) {
let indexs = parseInt(Math.floor(Math.random() * lengthOfTempWords));
let count = 0;
for (let i = 0; i < indexArray.length; i++) {
if (indexs == indexArray[i]) {
count++;
}
}
if (count > 0) {
if (indexArray.length === lengthOfTempWords) {
return -1;
}
return getValidIndex(indexArray, lengthOfTempWords);
} else {
indexArray.push(indexs);
return indexs;
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.