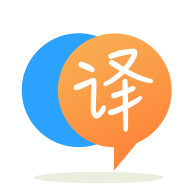
[英]Is there a way to use MUI Autocomplete when the search string is not in the option label?
[英]MUI <Autocomplete/> input label different from search option
在这个演示中: https://codesandbox.io/s/oxvsfy?file=/demo.js我可以用它的名字搜索一个国家(西班牙、法国等)。 但是当我单击时,我希望保留在 TextField 上的值是国家/地区的电话号码。
有可能实现吗? 谢谢你。
为此,您需要将 AutoComplete 转换为受控组件,并利用inputValue
属性
import React, { useState } from 'react';
import Autocomplete from '@mui/material/Autocomplete';
export default function CountrySelect() {
const [inputValue, setInputValue] = React.useState('');
const handleChange = e => {
const text = e.currentTarget.innerText
setInputValue(text.substring(text.indexOf('+'), text.length))
}
return (
<Autocomplete
options={countries}
onChange={handleChange}
inputValue={inputValue}
renderInput={(params) => (
<TextField
{...params}
/>
)}
/>
);
}
我有这样的东西,可能需要为 styles 安装额外的依赖项
import { StyledButton, StyledListbox, StyledOption } from '../../styles/Autocomplete.styles';
const [value, setValue] = useState("US")
return (
<CustomSelect value={value} onChange={(newValue) => setValue(newValue)}>
{countries.map((c, i) => (
<StyledOption key={i} value={c.code}>
<img loading="lazy"
width="20"
src={`https://flagcdn.com/w20/${c.code}.png`}
srcSet={`https://flagcdn.com/w40/${c.code}.png 2x`}
alt={`Flag of ${c.label}`} />
{c.label} {c.phone}
</StyledOption>
))}
</CustomSelect>
)
const StyledPopper = styled(PopperUnstyled)`
z-index: 1;
`;
const CustomSelect = forwardRef(function CustomSelect(
props: SelectUnstyledProps,
ref: React.ForwardedRef,
) {
const components: SelectUnstyledProps['components'] = {
Root: StyledButton,
Listbox: StyledListbox,
Popper: StyledPopper,
...props.components,
};
return <SelectUnstyled {...props} ref={ref} components={components} />;
});
Autocomplete.styles.js 中的 styles
import styled from "styled-components"
import OptionUnstyled, { optionUnstyledClasses } from '@mui/base/OptionUnstyled';
import {selectUnstyledClasses} from '@mui/base/SelectUnstyled';
export const StyledButton = styled('button')(() => `
font-family: IBM Plex Sans, sans-serif;
font-size: 0.875rem;
box-sizing: border-box;
min-height: calc(1.5em + 22px);
width: 200px;
background: '#fff';
border: 1px solid grey;
border-radius: 4px;
margin: 0.5em;
padding: 10px;
text-align: left;
line-height: 1.5;
color: grey;
&.MuiSelectUnstyled-popper {
background: white;
}
&:hover {
background: #e4e4e4;
border-color: grey;
}
&.${selectUnstyledClasses.focusVisible} {
outline: 3px solid blue;
}
&.${selectUnstyledClasses.expanded} {
&::after {
content: '▴';
}
}
&::after {
content: '▾';
float: right;
}
& img {
margin-right: 10px;
}
`);
export const StyledListbox = styled('ul')(() => `
font-family: IBM Plex Sans, sans-serif;
font-size: 0.875rem;
box-sizing: border-box;
border-radius: 4px;
margin: 0;
width: 200px;
max-height: 400px;
background: '#fff';
color: grey;
overflow: auto;
outline: 0px;
border: 1px solid lightgray;
`);
export const StyledOption = styled(OptionUnstyled)(() => `
list-style: none;
padding: 8px;
cursor: default;
background: #fff;
&:last-of-type {
border-bottom: none;
}
&.${optionUnstyledClasses.selected} {
background-color: #e7e7ff;
color: blue;
}
&.${optionUnstyledClasses.highlighted} {
background-color: #e4e4e4;
color: grey;
}
&.${optionUnstyledClasses.highlighted}.${optionUnstyledClasses.selected} {
background-color: #e7e7ff;
color: blue;
}
&.${optionUnstyledClasses.disabled} {
color: grey;
}
&:hover:not(.${optionUnstyledClasses.disabled}) {
background-color: #e4e4e4;
color: grey;
}
& img {
margin-right: 10px;
}
`);
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.