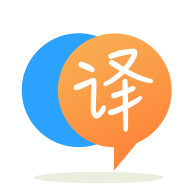
[英]How do I print every object in an arraylist and correctly count their quantity?
[英]How do I count every unique item in an Arraylist?
我需要计算 Arraylist 中的每个唯一字符。我已经将每个字符分开。
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
import java.util.ArrayList;
public class Aschenputtel {
public static void main(String args[]) {
ArrayList <String> txtLowCase = new ArrayList <String> ();
ArrayList <Character> car = new ArrayList <Character> ();
File datei = new File ("C:/Users/Thomas/Downloads/Aschenputtel.txt");
Scanner scan = null;
try {
scan = new Scanner (datei);
} catch (FileNotFoundException e) {
System.out.println("File not found.");
}
while (scan.hasNext()) {
String temp = scan.next().replace("„", "„").replace("“", "“").toLowerCase();
txtLowCase.add(temp);
for(int i = 0; i < temp.length(); i++) {
car.add(temp.charAt(i));
}
}
System.out.println(car);
}
}
那是我当前的代码。 car 目前给出了每个字符,但结果应该是这样的:a = 16, b = 7, c = 24,....有没有好的方法来做到这一点?
一旦你有了你的character
,你就可以在你的 for 循环中做一些类似的事情:
...
Map<Character, Integer> map2=new HashMap<Character, Integer>();
for (int i = 0; i < temp.length(); i++) {
map2.put(temp.charAt(i), map2.getOrDefault(temp.charAt(i), 0)+1);
}
System.out.println(map2);
...
您已经了解了算法的第一部分,即数据处理:
car
。您想要将每个字符关联到一个计数。 一个 HashMap 就可以了。
这是第二部分的方法,有一些解释:
/*
This will return something that looks like:
{ a: 16, b: 7, c: 24, ... }
*/
HashMap<Character, Int> getCharacterCount(ArrayList<Character> charList) {
// for each character we associate an int, its count.
HashMap<Character, Int> counter = new Hashmap<>();
for (Character car: charList) {
// if the map doesn't contain our character, we've never seen it before.
int currentCount = counter.containsKey(car) ? counter.get(car) : 0;
// increment this character's current count
counter.put(car, currentCount + 1);
}
return counter;
}
如果您需要计算汽车列表中的字符数,您可以使用集合 stream 和聚合操作groupingby
结合下游counting
。
此操作将产生一个Map
,其键是列表的字符,它们的对应值是它们在列表中的出现。
List<Character> list = new ArrayList<>(List.of('a', 'a', 'a', 'b', 'c', 'b', 'b', 'b', 'a', 'c'));
Map<Character, Long> mapRes = list.stream().collect(Collectors.groupingBy(c -> c, Collectors.counting()));
System.out.println(mapRes);
public class Aschenputtel {
public static void main(String args[]) {
ArrayList<String> txtLowCase = new ArrayList<String>();
ArrayList<Character> car = new ArrayList<Character>();
File datei = new File("C:/Users/Thomas/Downloads/Aschenputtel.txt");
Scanner scan = null;
try {
scan = new Scanner(datei);
} catch (FileNotFoundException e) {
System.out.println("File not found.");
}
while (scan.hasNext()) {
String temp = scan.next().replace("„", "„").replace("“", "“").toLowerCase();
txtLowCase.add(temp);
for (int i = 0; i < temp.length(); i++) {
car.add(temp.charAt(i));
}
}
Map<Character, Long> mapRes = car.stream().collect(Collectors.groupingBy(c -> c, Collectors.counting()));
System.out.println(mapRes);
}
}
这是最简单的方法,在初始化时将数组列表传递给 HashSet。
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
import java.util.ArrayList;
public class Aschenputtel {
public static void main(String args[]) {
ArrayList <String> txtLowCase = new ArrayList <String> ();
ArrayList <Character> car = new ArrayList <Character> ();
File datei = new File ("C:/Users/Thomas/Downloads/Aschenputtel.txt");
Scanner scan = null;
try {
scan = new Scanner (datei);
} catch (FileNotFoundException e) {
System.out.println("File not found.");
}
while (scan.hasNext()) {
String temp = scan.next().replace("„", "„").replace("“", "“").toLowerCase();
txtLowCase.add(temp);
for(int i = 0; i < temp.length(); i++) {
car.add(temp.charAt(i));
}
}
HashSet<String> hset = new HashSet<String>(ArrList); // Only adds an element if it doesn't exists prior.
System.out.println("ArrayList Unique Values is : " + hset); // All the unique values.
System.out.println("ArrayList Total Coutn Of Unique Values is : " + hset.size()); // Count of all unique items.
}
}
如果你想有更多的控制/可定制性,你也可以这样做:
private static void calcCount(List<Character> chars) {
chars.sort(Comparator.naturalOrder());
Character prevChar = null;
int currentCount = 0;
for (Character aChar : chars) {
if (aChar != prevChar) {
if (prevChar != null) {
System.out.print(currentCount + " ");
currentCount = 0;
}
System.out.print(aChar + ":");
prevChar = aChar;
}
currentCount++;
}
System.out.print(currentCount);
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.