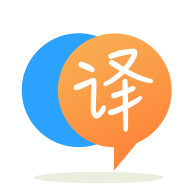
[英]Why do javascript objects output as “undefined” when looping through an array?
[英]Looping through array of objects, output created elements to html with javascript
我创建了一个构造函数和一些推入数组的对象。
从这里我需要在一个输出到基本 html 前端的数组中调用这些对象的函数。 我已经创建了构造函数,并且大部分时间都在工作,但是我在开发工具中不断收到错误,说明下面显示的 this.render() 方法不是函数。
当我尝试 console.log 数组中的对象(使用循环)时,我可以让它工作,但无法克服无法使用 render() 方法的错误。 任何帮助,将不胜感激。 代码如下。 请注意,最终目标是将渲染信息附加到 id 为“输出”的 div。 谢谢
function ArtistType(name, genre, albums) { this.name = name; this.genre = genre; this.albums = albums; this.publishAlbum = function () { this.albums++; }; this.render = function () { const artistName = document.createElement("h2"); artistName.innerText = this.name; const genre = document.createElement("div"); genre.innerText = this.genre; const albums = document.createElement("div"); albums.innerText = this.albums; document.getElementById("output").appendChild(artistName, genre, albums) }; } let artists = []; const artist1 = new ArtistType("Black Sabbath", "metal", 0) const artist2 = new ArtistType("Huey Lewis and the News", "rock", 0) const artist3 = new ArtistType("Beastie Boys", "hip hop", 0) artists.push(artist1, artist2, artist3); artist1.publishAlbum(); //below some of what I have tried... //artists.forEach(this.render()) //have also tried for...in loop and others...example: //for (var i = 0; i < artists.length; i++) { // this.render(); }
<html> <head> <title>Test</title> </head> <body> <h1>Name will go here</h1> <!-- Output the content --> <div id="output"></div> <!-- Link the script --> <script src="scripts.js"></script> </body> </html>
您必须引用特定对象才能使用其函数render()
。 像这样的东西:
function ArtistType(name, genre, albums) { this.name = name; this.genre = genre; this.albums = albums; this.publishAlbum = function () { this.albums++; }; this.render = function () { const artistName = document.createElement("h2"); artistName.innerText = this.name; const genre = document.createElement("div"); genre.innerText = this.genre; const albums = document.createElement("div"); albums.innerText = this.albums; document.getElementById("output").appendChild(artistName, genre, albums) }; } let artists = []; const artist1 = new ArtistType("Black Sabbath", "metal", 0) const artist2 = new ArtistType("Huey Lewis and the News", "rock", 0) const artist3 = new ArtistType("Beastie Boys", "hip hop", 0) artists.push(artist1, artist2, artist3); artist1.publishAlbum(); //below some of what I have tried... //artists.forEach(this.render()) //have also tried for...in loop and others...example: for (var i = 0; i < artists.length; i++) { artists[i].render(); }
<div id="output"></div>
this
存在于函数内部,你不能只是在它之外神奇地使用它试试这个:
artists.forEach(artist => artist.render())
或者
for (const artist of artists) {
artist.render()
}
在 for 循环中访问数组时,您应该使用带括号的注释和索引 - Artists artists[i].render();
. 在我的示例中,我实际上使用了forEach
来向您展示另一种循环方式。 您可以参考此文档以了解有关forEach
的更多信息。
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/forEach
除非您需要支持旧浏览器,否则您可以考虑使用类。 类也有助于简化代码,并有助于更轻松地访问对象。 更符合其他面向对象的语言。
最后,请注意我使用的是append
而不是appendChild
。 append
允许您传入多个节点并将它们全部附加。 但是它不支持 IE。 appendChild
只会追加您传入的第一个节点。
class Artist { constructor(name, genre, albums) { this.name = name; this.genre = genre; this.albums = albums; } publishAlbum() { this.albums++; } render() { const artistName = document.createElement("h2"); artistName.innerText = this.name; const genre = document.createElement("div"); genre.innerText = this.genre; const albums = document.createElement("div"); albums.innerText = this.albums; document.getElementById("output").append(artistName, genre, albums) } } let artists = [new Artist("Black Sabbath", "metal", 0), new Artist("Huey Lewis and the News", "rock", 0), new Artist("Beastie Boys", "hip hop", 0) ]; artists[0].publishAlbum(); artists.forEach(function(artist) { artist.render(); });
<div id="output"></div>
你可以做:
function ArtistType(name, genre, albums) { this.name = name this.genre = genre this.albums = albums this.publishAlbum = function (event) { // Logic to publish album console.log('event', event.target) this.albums++ } this.render = function () { const wrapper = document.createElement('div') const name = document.createElement('h2') name.innerHTML = this.name const genreP = document.createElement('p') genreP.innerHTML = `Genre: ${this.genre.toUpperCase()}` const albumsP = document.createElement('p') albumsP.innerHTML = `Albums: ${this.albums}` albumsP.addEventListener('click', this.publishAlbum) const elems = [name, genreP, albumsP] elems.forEach(el => wrapper.appendChild(el)) document .getElementById('output') .appendChild(wrapper) } } const data = [{name: 'Black Sabbath',genre: 'metal',albums: 0,},{name: 'Huey Lewis and the News',genre: 'rock',albums: 0,},{name: 'Beastie Boys',genre: 'hip hop',albums: 0,},] data.forEach(({ name, genre, albums }) => { const artist = new ArtistType(name, genre, albums) artist.render() })
<div id="output"></div>
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.