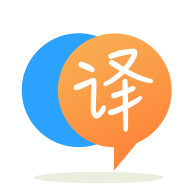
[英]Scanner NoSuchElementException when calling .next() method
[英]NoSuchElementException not working when calling method
import java.util.*;
public class Main {
static void factorFinder() {
Scanner sc = new Scanner(System.in);
boolean valid = false;
int number = 0;
while(! valid ){
System.out.println("Enter the number you want to find the factor of(Numbers only)");
try {
number = sc.nextInt();
valid = true;
} catch (InputMismatchException e) {
System.out.println("Not a number.");
sc.next();
}
}
sc.close();
System.out.print("Factors of " + number + " are: ");
for (int i = 1; i <= number; ++i) {
// if number is divided by i
// i is the factor
if (number % i == 0) {
System.out.print(i + " ");
}
}
}
public static void main(String[] args) {
Scanner choiceScanner = new Scanner(System.in);
System.out.println("Do you want to use the factor finder? (y/n)");
String answer = choiceScanner.nextLine();
choiceScanner.close();
if(answer.equals("y")){
factorFinder();
}else{
System.exit(0);
}
}
}
这是终端输出(通过replit运行)
sh -c javac -classpath .:target/dependency/* -d . $(find . -type f -name '*.java')
java -classpath .:target/dependency/* Main
Do you want to use the factor finder? (y/n)
y
Enter the number you want to find the factor of(Numbers only)
Exception in thread "main" java.util.NoSuchElementException
at java.base/java.util.Scanner.throwFor(Scanner.java:937)
at java.base/java.util.Scanner.next(Scanner.java:1594)
at java.base/java.util.Scanner.nextInt(Scanner.java:2258)
at java.base/java.util.Scanner.nextInt(Scanner.java:2212)
at Main.factorFinder(Main.java:13)
at Main.main(Main.java:38)
exit status 1
我正在制作一个计算器,可以找到给定数字的因数。 计算器部分已完成并且正在工作。 但是,它要求使用计算器的部分不起作用。 它给出了 NoSuchElementException 错误。 有人可以帮助我吗?
关闭Scanner
后,您将无法读取另一个 Scanner。
因此,您有两种可能的解决方案(如我所见):
1(推荐)。 仅使用一台Scanner
。 您可以使其成为类成员或将其作为参数传递给方法。
import java.util.*;
public class Main {
static Scanner sc = new Scanner(System.in);
static void factorFinder() {
boolean valid = false;
int number = 0;
while(! valid ){
System.out.println("Enter the number you want to find the factor of(Numbers only)");
try {
number = sc.nextInt();
valid = true;
} catch (InputMismatchException e) {
System.out.println("Not a number.");
sc.next();
}
}
System.out.print("Factors of " + number + " are: ");
for (int i = 1; i <= number; ++i) {
// if number is divided by i
// i is the factor
if (number % i == 0) {
System.out.print(i + " ");
}
}
}
public static void main(String[] args) {
System.out.println("Do you want to use the factor finder? (y/n)");
String answer = choiceScanner.nextLine();
if(answer.equals("y")){
factorFinder();
}else{
sc.close();
System.exit(0);
}
sc.close();
}
}
factorFinder()
方法后才关闭choiceScanner
。public class Main2 {
static Scanner sc = new Scanner(System.in);
static void factorFinder() {
boolean valid = false;
int number = 0;
while(! valid ){
System.out.println("Enter the number you want to find the factor of(Numbers only)");
try {
number = sc.nextInt();
valid = true;
} catch (InputMismatchException e) {
System.out.println("Not a number.");
sc.next();
}
}
System.out.print("Factors of " + number + " are: ");
for (int i = 1; i <= number; ++i) {
// if number is divided by i
// i is the factor
if (number % i == 0) {
System.out.print(i + " ");
}
}
}
public static void main(String[] args) {
Scanner choiceScanner = new Scanner(System.in);
System.out.println("Do you want to use the factor finder? (y/n)");
String answer = choiceScanner.nextLine();
if(answer.equals("y")){
factorFinder();
}else{
sc.close();
System.exit(0);
}
sc.close();
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.