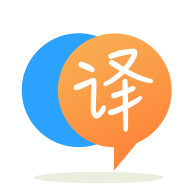
[英]How to have different color for dropdown label and dropdown list text in Flutter?
[英]I have an issue saving a different list on multiple dropdown button in Flutter
我是 Flutter 的菜鸟,毕竟是编程。 我正在完成我作为土木工程师毕业的最后一个项目,但我决定编写一个适用于 Android 的应用程序,用于计算用于微桩设计的各种方程。
我已经完成了模拟披萨订单的代码(这对解释变得更加友好),但是我在保存不同列表中的值时遇到问题,这些列表会根据披萨素食或肉类而变化,我仍在努力。
我可以达到这样做的方法或表格,请有人解释或帮助我。
到目前为止的代码:
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:provider/provider.dart';
//Provider
class Datos with ChangeNotifier {
List _datos = [' '];
List get datos => _datos;
set datos(List value){
_datos = value;
notifyListeners();
}
void goVegan () {
_datos = [' ','Vegan 1', 'Vegan 2', 'Vegan 3'];
notifyListeners();
}
void goMeat () {
_datos = [' ', 'Meat 1', 'Meat 2', 'Meat 3'];
notifyListeners();
}
}
class lechada extends StatelessWidget {
// const lechada({Key? key}) : super(key: key);
int nes = 0;
@override
Widget build(BuildContext context) {
return ChangeNotifierProvider<Datos>(
create: (context) => Datos(),
child: Scaffold(
appBar: AppBar(
title: const Text('definitivo'),
),
body:Column(
children: [
entrada('cantidad de Pizzas'),
cuerpo(),//nes),
],
),
),
);
}
//inputs de formatos
entrada(String hint){
return TextFormField(
validator: (String? value) { //Esta valida que no este vacio
if (value == null || value.isEmpty) {
return 'Campo requerido'; //campo en caso de que este vacio
}
return null;
},
onSaved: (value) => nes = int.parse(value!),//eje.add(double.parse(value!)), //Aca guarda el valor
decoration: InputDecoration(
hintText: hint,
// icon: Icon(Icons.calendar_view_day_sharp, color: Color(0xFFB14246))
),
keyboardType: TextInputType.number,
);
}
}
class cuerpo extends StatefulWidget {
// const cuerpo({Key? key}) : super(key: key);
int nes = 3;
// cuerpo (int nes);
@override
State<cuerpo> createState() => _cuerpoState();
}
class _cuerpoState extends State<cuerpo> {
// List selec = [' ','Pizza Vegan','Pizza Meat'];
String initial = ' ';
String initial2 = ' ';
@override
Widget build(BuildContext context) {
final datos = Provider.of<Datos>(context,listen: false);
return Column(
children: [
ListView.builder(
shrinkWrap: true,
itemCount: widget.nes ,
itemBuilder: (BuildContext context, int index) => datosPizza(),
// {
// return datosPizza();
// },
),
SizedBox(height: 20),
Container(
padding: EdgeInsets.all(8),
child: Text('selec.toString()'),
),
SizedBox(height: 20),
Row(
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: [
ElevatedButton(onPressed: (){
setState(() {
initial = ' ';
datos.goVegan();
});
}, child: Text('Go Vegan')),
ElevatedButton(onPressed: (){
setState(() {
initial = ' ';
datos.goMeat();
});
}, child: Text('Go Meat')),
],
)
],
);
}
}
class datosPizza extends StatefulWidget {
// const datosPizza({Key? key}) : super(key: key);
@override
_datosPizzaState createState() => _datosPizzaState();
}
class _datosPizzaState extends State<datosPizza> {
List selec = [' ','Pizza Vegan','Pizza Meat'];
String initial = ' ';
String initial2 = ' ';
@override
Widget build(BuildContext context) {
final datos = Provider.of<Datos>(context,listen: false);
return Row(
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: [
Container(
padding: EdgeInsets.all(8),
child: DropdownButton(
value: initial,
//Aca recibe los valores y los pasa al map
items: selec.map((value){ //datos se mete al list
return DropdownMenuItem(
child: new Text(value),
value:value,
);
}).toList(),
onChanged: (value) {
value.toString() == 'Pizza Vegan' ?datos.goVegan() :null;
value.toString() == 'Pizza Meat' ?datos.goMeat() :null;
initial2 = ' ';
setState(() {
initial = value.toString();
});
})
),
Container(
padding: EdgeInsets.all(8),
child: DropdownButton(
value: initial2,
//Aca recibe los valores y los pasa al map
items: datos.datos.map((value){ //datos se mete al list
return DropdownMenuItem(
child: new Text(value),
value:value,
);
}).toList(),
onChanged: (value) {
setState(() {
initial2 = value.toString();
});
})
),
],
);
}
}
请不要责怪我的英语,我是西班牙裔母语人士
更新:我找到了问题的解决方案,简化是为主要树小部件创建更多的孩子,并继承这些值,所以小部件将保存我们需要的这些值,我将分享代码示例:
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:provider/provider.dart';
//Provider
class Datos with ChangeNotifier {
List _datos = [' '];
List guardado = [];
List gg = [];
List<String> esgua = [];
List<double> algua = [];
List<double> zgua = [];
// List guardado = [' '];
int p = 0;
List get datos => _datos;
set datos(List value){
_datos = value;
notifyListeners();
}
void goVegan () {
_datos = [' ','Vegan 1', 'Vegan 2', 'Vegan 3'];
// p = 0;
notifyListeners();
}
void goMeat () {
_datos = [' ', 'Meat 1', 'Meat 2', 'Meat 3'];
// p = 1;
notifyListeners();
}
void plus () {
p++;
notifyListeners();
}
}
class lechada extends StatelessWidget {
// const lechada({Key? key}) : super(key: key);
// int nes = 0;
@override
Widget build(BuildContext context) {
return ChangeNotifierProvider<Datos>(
create: (context) => Datos(),
child: Scaffold(
appBar: AppBar(
title: const Text('definitivo'),
),
body:Column(
children: [
Text('Toma pedido'),
cuerpo(),
],
),
),
);
}
}
class cuerpo extends StatefulWidget {
// const cuerpo({Key? key}) : super(key: key);
@override
State<cuerpo> createState() => _cuerpoState();
}
class _cuerpoState extends State<cuerpo> {
// List selec = [' ','Pizza Vegan','Pizza Meat'];
String initial = ' ';
String initial2 = ' ';
// GlobalKey<FormState> dkey = GlobalKey<FormState>();
@override
Widget build(BuildContext context) {
final datos = Provider.of<Datos>(context);
return Column(
children: [
ListView.builder(
shrinkWrap: true,
itemCount: datos.p,
itemBuilder: (BuildContext context, int index) => datosPizza(),
// {
// return datosPizza();
// },
),
SizedBox(height: 20),
Container(
padding: EdgeInsets.all(8),
child: Text('selec.toString()'),
),
SizedBox(height: 20),
Row(
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: [
ElevatedButton(
onPressed: (){
setState(() {
print (datos.gg);
datos.p!=datos.gg.length
?print ('error')
:null;
});
}, child: Text('Print')
),
ElevatedButton(
onPressed: (){
datos.p = 0;
datos.gg.clear();
},
child: Text('Reinicia')),
ElevatedButton(
onPressed: (){
datos.plus();
},
child: Text('Add estrato')
),
],
)
],
);
}
}
class datosPizza extends StatefulWidget {
const datosPizza({Key? key}) : super(key: key);
@override
_datosPizzaState createState() => _datosPizzaState();
}
class _datosPizzaState extends State<datosPizza> {
List selec = [' ','Pizza Vegan','Pizza Meat'];
String initial = ' ';
String initial2 = ' ';
List temp = [];
List<double> eje = [];
@override
Widget build(BuildContext context) {
final datos = Provider.of<Datos>(context,listen: false);
return Row(
mainAxisAlignment: MainAxisAlignment.spaceAround,
children: [
Container(
width: 150,
padding: EdgeInsets.all(8),
child: DropdownButton(
value: initial,
//Aca recibe los valores y los pasa al map
items: selec.map((value){ //datos se mete al list
return DropdownMenuItem(
child: new Text(value),
value:value,
);
}).toList(),
onChanged: (value) {
value.toString() == 'Pizza Vegan' ?datos.goVegan() :null;
value.toString() == 'Pizza Meat' ?datos.goMeat() :null;
initial2 = ' ';
temp.addAll(datos._datos); //Añade los datos de entrada y los pasa al widget para que los conserve
setState(() {
initial = value.toString();
});
})
),
Container(
width: 100,
padding: EdgeInsets.all(8),
child: dropdowBut(temp),
),
Container(
width: 70,
padding: EdgeInsets.all(8),
child: entrada('mts'),
)
],
);
}
//inputs de formatos
entrada(String hint){
return TextFormField(
validator: (String? value) { //Esta valida que no este vacio
if (value == null || value.isEmpty) {
return 'Campo requerido'; //campo en caso de que este vacio
}
return null;
},
onSaved: (value) => eje.add(double.parse(value!)),//eje.add(double.parse(value!)), //Aca guarda el valor
onFieldSubmitted: (value) => print(value),
// onChanged: (value)=> print(value),
decoration: InputDecoration(
hintText: hint,
// icon: Icon(Icons.calendar_view_day_sharp, color: Color(0xFFB14246))
),
keyboardType: TextInputType.number,
);
}
}
class dropdowBut extends StatefulWidget {
// const dropdowBut({Key? key}) : super(key: key);
String initial2 =' ';
List temp ;
dropdowBut(this.temp);
@override
_dropdowButState createState() => _dropdowButState();
}
class _dropdowButState extends State<dropdowBut> {
String _value = ' ';
@override
Widget build(BuildContext context) {
final datos = Provider.of<Datos>(context,listen: false);
return DropdownButton(
// value: widget.initial2,
value: _value,
// hint: Text('hi'),
//Aca recibe los valores y los pasa al map
items:
widget.temp.map((value){ //datos se mete al list
return DropdownMenuItem(
child: new Text(value),
value:value,
);
}).toList(),
onChanged: (value) {
datos.gg.add(value);
setState(() {
_value = value.toString();
});
});
}
}
对不起,如果我纠结了代码,但我还在学习 Flutter。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.