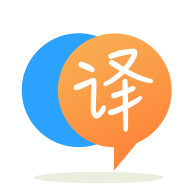
[英]How to move cursor-to-next from one input to another input and button by pressing keyboard enter in angular 6
[英]The cursor is not moving from one input to the next by pressing an Enter button in JavaScript
我希望光标仅在按下回车按钮时从一个输入移动到下一个输入。 当它到达最后一个输入时,我希望它再次将其移动到第一个输入(如循环)。 问题在于这段 JavaScript 代码。 我使用 OpenAI Playground 生成了它。
在这种情况下是否需要向按钮添加事件侦听器? 如果是这样,如何在输入字段中调用此代码?
var inputs = document.querySelectorAll("input");
inputs.forEach(input => {
input.addEventListener('keyup', function(e) {
if (e.keyCode === 13) {
var nextInput = inputs[inputs.indexOf(e.target) + 1];
if (nextInput) {
nextInput.focus();
}
}
})
});
完整的html在这里:
<!DOCTYPE html>
<html>
<head>
<title>
Inserting meaning
</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css">
<meta name="viewport" content="width=device-width, initial-scale=1">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.12.4/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
<style>
#one
{
margin-left:auto;
margin-right:auto;
width:90%;
}
</style>
</head>
<body>
<script>
var inputs = document.querySelectorAll("input");
inputs.forEach(input => {
input.addEventListener('keyup', function(e) {
if (e.keyCode === 13) {
var nextInput = inputs[inputs.indexOf(e.target) + 1];
if (nextInput) {
nextInput.focus();
}
}
})
});
</script>
<div align ="center">
<h1>
Meaning In Sentence
</h1>
<div>
<h3>
Sentence:
</h3>
<input type="text" id="sentence" class="form-control" style="width:80%" placeholder="Enter the sentence">
</div>
<br>
<h3>
Difficult Word:
</h3>
<input type="text" id="word" class="form-control" style="width:80%" placeholder="Enter the difficult word">
<br>
<h3>
Meaning:
</h3>
<input type="text" id="meaning" class="form-control" style="width:80%" onchange="func()" placeholder="Enter the meaning">
<br>
</div>
<br>
<div class="h5" align = "center" id="modifiedsentence" onchange="func()">
<div>
<script>
function func()
{
var s=document.getElementById("sentence").value.replace(/['"]+/g, '');
var w=document.getElementById("word").value;
var m=document.getElementById("meaning").value;
s=s[0].toUpperCase()+s.slice(1);
var f=s.replace(w,w+" ("+m+") ")+"<br>"+
document.getElementById("modifiedsentence").innerHTML;
document.getElementById("modifiedsentence").innerHTML = f.toString();
document.getElementById("sentence").value = " ";
document.getElementById("word").value = " ";
document.getElementById("meaning").value = " ";
mvCursor();
// console.log(f);
}
</script>
</div>
</body>
</html>
您可以使用 forEach 中的索引参数来掌握索引。
inputs.forEach((input, index) => { // HERE grab the index
input.addEventListener('keyup', function(e) {
if (e.keyCode === 13) {
var nextInput = inputs[index + 1]; // // HERE use the index
if (nextInput) {
nextInput.focus();
}
}
})
});
完整的代码段进行测试:
<html> <head> <title> Inserting meaning </title> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css"> <meta name="viewport" content="width=device-width, initial-scale=1"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.12.4/jquery.min.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script> <style> #one { margin-left:auto; margin-right:auto; width:90%; } </style> </head> <body> <div align ="center"> <h1> Meaning In Sentence </h1> <div> <h3> Sentence: </h3> <input type="text" id="sentence" class="form-control" style="width:80%" placeholder="Enter the sentence"> </div> <br> <h3> Difficult Word: </h3> <input type="text" id="word" class="form-control" style="width:80%" placeholder="Enter the difficult word"> <br> <h3> Meaning: </h3> <input type="text" id="meaning" class="form-control" style="width:80%" onchange="func()" placeholder="Enter the meaning"> <br> </div> <br> <div class="h5" align = "center" id="modifiedsentence" onchange="func()"> <div> </div> <script> function func() { var s=document.getElementById("sentence").value.replace(/['"]+/g, ''); var w=document.getElementById("word").value; var m=document.getElementById("meaning").value; s=s[0].toUpperCase()+s.slice(1); var f=s.replace(w,w+" ("+m+") ")+"<br>"+ document.getElementById("modifiedsentence").innerHTML; document.getElementById("modifiedsentence").innerHTML = f.toString(); document.getElementById("sentence").value = " "; document.getElementById("word").value = " "; document.getElementById("meaning").value = " "; // mvCursor(); REMOVING AS THIS IS NOT DEFINED // console.log(f); } var inputs = document.querySelectorAll("input"); inputs.forEach((input, index) => { input.addEventListener('keyup', function(e) { if (e.keyCode === 13) { var nextInput = inputs[index + 1]; if (nextInput) { nextInput.focus(); } } }) }); </script> </body> </html>
添加到上面蜜蜂零的答案:
您可以检查最后一个输入元素,当按下返回键到达最后一个输入元素时,您可以聚焦到第零个索引。
const nextinputIndex = index < (inputs.length - 1) ? (index + 1) : 0;
测试片段:
<html> <head> <title> Inserting meaning </title> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css"> <meta name="viewport" content="width=device-width, initial-scale=1"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/1.12.4/jquery.min.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script> <style> #one { margin-left:auto; margin-right:auto; width:90%; } </style> </head> <body> <div align ="center"> <h1> Meaning In Sentence </h1> <div> <h3> Sentence: </h3> <input type="text" id="sentence" class="form-control" style="width:80%" placeholder="Enter the sentence"> </div> <br> <h3> Difficult Word: </h3> <input type="text" id="word" class="form-control" style="width:80%" placeholder="Enter the difficult word"> <br> <h3> Meaning: </h3> <input type="text" id="meaning" class="form-control" style="width:80%" onchange="func()" placeholder="Enter the meaning"> <br> </div> <br> <div class="h5" align = "center" id="modifiedsentence" onchange="func()"> <div> </div> <script> function func() { var s=document.getElementById("sentence").value.replace(/['"]+/g, ''); var w=document.getElementById("word").value; var m=document.getElementById("meaning").value; s=s[0].toUpperCase()+s.slice(1); var f=s.replace(w,w+" ("+m+") ")+"<br>"+ document.getElementById("modifiedsentence").innerHTML; document.getElementById("modifiedsentence").innerHTML = f.toString(); document.getElementById("sentence").value = " "; document.getElementById("word").value = " "; document.getElementById("meaning").value = " "; // mvCursor(); REMOVING AS THIS IS NOT DEFINED // console.log(f); } var inputs = document.querySelectorAll("input"); inputs.forEach((input, index) => { input.addEventListener('keyup', function(e) { if (e.keyCode === 13) { const nextinputIndex = index < (inputs.length - 1) ? (index + 1) : 0; var nextInput = inputs[nextinputIndex]; if (nextInput) { nextInput.focus(); } } }) }); </script> </body> </html>
可能需要做一个小循环才能找到下一个输入。
var nextInput = e.target.nextElementSibling;
var found = false;
while (nextInput) {
if (nextInput.classList.contains("form-control")) {
found = true;
break;
}
nextInput = nextInput.nextElementSibling
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.