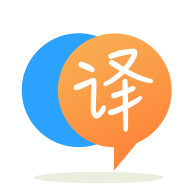
[英]Count the number of unique occurrences in an array that contain a specific string with Javascript
[英]Find unique characters in a string and the count of their occurrences
我需要计算给定字符串中字符的出现次数并打印出唯一字符以及它们出现的次数。 因此,例如,如果我收到一串“HELLO”,它应该打印出来:
H:1,E:1,L:2,O:1
这是一个问题的简化版本,但答案应该让我朝着正确的方向前进。 我该如何解决这个问题?
先感谢您。
这或多或少应该是它的样子,以便更容易以 JSON 格式打印它,如果需要,您已经可以自己将其转换为 String。
function count_occurrence(text = "") {
const array_from_text = text.split("");
const result = {};
Array.from(new Set(array_from_text)).forEach(word => {
const { length } = array_from_text.filter(w => w === word);
result[word] = length;
});
return result;
};
const occurences = count_occurence("HELLO");
console.log(occurences); // {H: 1, E: 1, L: 2, O: 1}
const countChars = (str) => {
const charCount = {} ;
for (const c of [...str]) {
charCount[c] = (charCount[c] || 0) + 1 ;
}
return charCount ;
}
console.log(countChars('HELLO')) ; // {H: 1, E: 1, L: 2, O: 1}
您可以使用Array.reduce()
来获取输入单词中每次出现的计数。
我们使用 ... 运算符将单词转换为数组,然后使用 .reduce() 创建一个对象,该对象具有单词中每个唯一字母的属性。
const input = 'HELLO'; const result = [...input].reduce((acc, chr) => { acc[chr] = (acc[chr] || 0) + 1; return acc; }, {}); console.log('Result:', result)
.as-console-wrapper { max-height: 100% !important; }
我解决这个问题的方法是:
let str = "HELLO"; // An object for the final result {character:count} let counts = {}; // Loop through the str... for (let index = 0; index < str.length; ++index) { // Get each char let ch = str.charAt(index); // Get the count for that char let count = counts[ch]; // If we have one, store that count plus one; if (count) { counts[ch] += 1; } else { // if not, store one counts[ch] = 1; } // or more simply with ternary operator // counts[ch] = count ? count + 1 : 1;. } console.log(counts);
也许最简单的答案就是拆分为 char 并将其放入地图中。
const count={}
"HELLO".split("").forEach(e=>{
count[e]??=0;
count[e]++;
})
count
是你想要的。
使用像数据结构这样的字典,它可以为您提供O(1)
访问和更新时间。 在 JS 中,您可以使用 Object literat(不推荐)或Map
。
遍历字符串的字符并通过增加当前字符的字符数来更新字典。 如果它不在您的字典中,请将其添加并将计数设置为 1。
完成迭代后,迭代字典的键,其中值是该特定字符的出现次数,并以您喜欢的任何格式输出它们。
const myStr = "Hello" const myMap = new Map() for (let c of myStr) { if (myMap.has(c)) { myMap.set(c, myMap.get(c)+1) } else { myMap.set(c, 1) } } for (let k of myMap.keys()) { console.log(`${k} occures ${myMap.get(k)} times`) }
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.