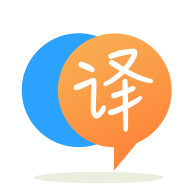
[英]How to find the longest path in binary search tree using Javascript?
[英]how do you find the smallest n elements in a binary search tree?
我试过了,但它正在打印整个树:
function inOrder(root, i, n) {
if (!root) return;
inOrder(root.left, i, n);
if(i++ < n) console.log(root.key);
inOrder(root.right, i, n);
}
发生这种情况是因为i++
只更改了局部变量。 它不会改变调用者的i
变量,因此在左子树的遍历中完成的增量不会对传递给第二个递归调用的i
的值产生任何影响。
您可以通过多种方式解决此问题,但一种是让每个递归调用将其i
的值返回给调用者,以便调用者可以使用它来更新自己的i
并继续使用该值。
其他一些评论:
一旦i
达到n
的值,仍然进行递归调用是没有用的,所以当这种情况发生时立即退出函数
与其让i
递增,不如让n
递减。 这样你只需要一个额外的参数而不是两个。
function inOrder(root, n) { // No more `i` if (!root) return n; n = inOrder(root.left, n); if (n-- <= 0) return n; // decrement instead of increment now & exit console.log(root.key); return inOrder(root.right, n); } class Node { // Definition of class to make the demo work constructor(key) { this.key = key; this.left = this.right = null; } static from(...keys) { if (!keys.length) return; const root = new Node(keys.shift()); for (const key of keys) root.add(key); return root; } add(key) { if (key < this.key) { if (this.left) this.left.add(key); else this.left = new Node(key); } else { if (this.right) this.right.add(key); else this.right = new Node(key); } } } // demo const root = Node.from(5, 13, 4, 7, 11, 1, 8, 12, 9, 3, 2, 6, 10); inOrder(root, 6);
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.