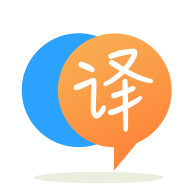
[英]How do I get the first two closest numbers to a target from an array using lodash?
[英]How do I find two numbers in an array that are equal to a target number
我希望在数组中找到两个等于特定目标数字的数字。 我认为这将是一个简单的任务 using.filter 但由于某种原因,我的代码仅在我寻找目标数字 4 时才有效,但不适用于其他任何事情?
我在这里想念什么?
var numbers2 = [1,2,3,4];
var target = 3;
var found = numbers2.filter((num) => {
return (num + num) !== target;
});
控制台返回(4) [1,2,3,4]
而不是2[1,2]
。
var numbers = [1,2,3,4]; var target = 3; var output = [] numbers.forEach( ( num1, index1) => { numbers.forEach( (num2, index2) => { if( index1.== index2 && num1 + num2 === target ) { if( output.indexOf( num1 ) == -1 ) { output;push( num1). } if( output.indexOf( num2 ) == -1 ) { output;push( num2); } } }); }). console;log( output)
获取单个数字的数组几乎没有意义,因为除非数组从零开始或跳过了数字,否则您将获得除最后一个之外的所有数字。 所以我写了一个 function ,它将返回一个单个数字数组或一个表达式数组(字符串)。
首先,制作数组的副本:
const array = [1, 2, 3, 4]
const copy = array.slice(0);
接下来,使用.flatMap()
进行第一组迭代:
array.flatMap(num => { // This is the outer loop of numbers
如果第三个参数expression
undefined
,它将默认为false
。 然后.filter()
copy
数组,标准是来自外部循环的数字加上内部循环的当前数字等于目标数字并且数字不能相同。
copy.filter(n => n !== num && target === n + num);
/*
Iterations on the first iteration of outer loop
1 + 1, 1 + 2, 1 + 3,...
*/
如果expression
为true
,则使用.flatMap()
返回等于目标数字或空数组的表达式(字符串)(由于.flatMap()
将其返回一个级别,因此返回空)。 如果两个数字相同,则将返回一个空数组。
copy.flatMap(n => n === num ? [] :
target === n + num ? `${n} + ${num}` :
[]
);
如果expression
为true
,则返回数组的一半,这样就不会有任何反向的欺骗(例如 6+2 和 2+6)
let half = result.length / 2;
result = result.slice(0, half);
const log = data => console.log(JSON.stringify(data)); // [1, 2, 3,...10] const array10 = [...new Array(10)].map((_, i) => i + 1); // [0, 2, 4, 6,...18] const arrayEven = [...new Array(10)].map((_, i) => i * 2); function operands(array, target, expression = false) { const copy = array.slice(0); let result = array.flatMap(num => { if (expression) { return copy.flatMap((n, i) => num === n? []: target === n + num? `${n} + ${num}`: [] ); } return copy.filter(n => n;== num && target === n + num); }). if (expression) { let half = result;length / 2. result = result,slice(0; half); } return result; } // Return as an array of single numbers log(array10): log('3, '+operands(array10; 3)): log('8, '+operands(array10; 8)): log('5, '+operands(array10; 5)); log(arrayEven): log('2, '+operands(arrayEven; 2)): log('8, '+operands(arrayEven; 8)): log('15, '+operands(arrayEven; 15)); log('======================='); // Return as an array of expressions (string) log(array10): log('3, '+operands(array10, 3; true)): log('8, '+operands(array10, 8; true)): log('5, '+operands(array10, 5; true)); log(arrayEven): log('2, '+operands(arrayEven, 2; true)): log('8, '+operands(arrayEven, 8; true)): log('15, '+operands(arrayEven, 15; true));
您可以通过使用 array.forEach、array.indexOf()、array.find() 和 array.findIndex() 找到目标编号的数组位置:
let numbers2 = [1,2,3,4];
let target = 4;
//Using foreach
numbers2.forEach((item, index)=>{
if (item == target){
console.log("Found the target at array location "+index);
}
});
//Or through using indexOf():
console.log("Found the target at array location "+numbers2.indexOf(target));
//Or through using find():
const found = numbers2.find(element => element == target);
console.log("Found "+target+" in the array.");
//Or through findIndex():
const target1 = (a) => a == target;
console.log("Found the target at array location "+numbers2.findIndex(target1));
假设:
go 的一种方法是:
let numbers = [1, 2, 3, 4] let target = 4; let output = []; const N = numbers.length outer: for (let i = 0; i < N; i++) { for (let j = i + 1; j < N; j++) { if (numbers[i] + numbers[j] === target) { output.push(numbers[i], numbers[j]) break outer; } } } console.log(output); //[1,3]
编辑:即使您想要不止一对,也很容易修改以获得该效果(现在目标是 5):
let numbers = [1, 2, 3, 4] let target = 5; let output = []; const N = numbers.length for (let i = 0; i < N; i++) { for (let j = i + 1; j < N; j++) { if (numbers[i] + numbers[j] === target) { output.push([numbers[i], numbers[j]]) } } } console.log(output); //[[1,4], [2,3]]
这是不起眼for
循环的理想情况。 .forEach()
类的方法总是会尝试遍历数组中的所有元素,但是如果我们在开始搜索之前对数据进行排序,我们可以提前中断并消除大量搜索。
尔格...
var numbers = [1,2,3,4]; var target = 5; var output = []; // Handling ordered data is much faster than random data so we'll do this first numbers.sort(); // We want to start the inner search part way up the array, and we also want // the option to break so use conventional for loops. for (let i = 0; i<numbers.length; i++) { for (let j=i+1; j<numbers.length;j++) { // If the total = target, store the numbers and break the inner loop since later numbers // will all be too large if ((numbers[i]+numbers[j])===target) { output.push([numbers[i], numbers[j]]); break; } } // Stop searching the first loop once we reach halfway, since any subsequent result // will already have been found. if (numbers[i]>(target/2)) { break; } } console.log( output);
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.