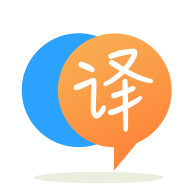
[英]How to map over an object which has a unique id for each object values and return the values to a React component
[英]Identify unique values in the array and for each one will return an object - how to do with a loop or a hash map
我有个问题。 我的任务是写一个 function :
下面的 function 工作得很好,但我想知道我怎样才能做得更好。 或者可能使用循环或 hash map? 我想改进我的游戏!
const data = [
"Test1",
"Test1",
"Test2",
"Test2",
"Test2",
"Test3",
"Test3",
"Test3",
"Test3",
"Test4",
"Test4",
"Test4",
]
function calcComponent(data: string[]) {
const indices = data.map((el: string) => data.indexOf(el)*10);
const outputPosition = new Set(indices);
const outputNames = [...new Set(data)];
const output_array: number[] = [...outputPosition];
let result = outputNames.map((_, index) => {
if(index == outputNames.length-1){
return {
name: outputNames[index],
start: output_array[index],
end: "120%"
}
}
else{
return {
name: outputNames[index],
start: output_array[index].toString() + "%",
end: output_array[index+1].toString() + "%"
}
}
})
console.log(result)
}
calcComponent(data)
嘿,我对这个解决方案做了一些假设,希望它们对你没问题:
Test2
,您仍然可以拥有Test1
end: 100%
,第一项start: 0%
const data = [
"Test1",
"Test1",
"Test2",
"Test2",
"Test2",
"Test3",
"Test3",
"Test3",
"Test3",
"Test1",
"Test4",
"Test4",
"Test2",
"Test4",
"Test3"
]
function calcComponent(data: string[]) {
return data.filter((val, index, array) => array.indexOf(val) == index).map((val) => {
return {
name: val,
start: ((data.indexOf(val) / (data.length - 1)) * 100) + "%", // or just (data.indexOf(val) * 10) + "%"
end: ((data.lastIndexOf(val) / (data.length - 1)) * 100) + "%" // or just (data.lastIndexOf(val) * 10) + "%"
}
});
}
const result = calcComponent(data);
console.log({ result });
output 就像:
{
"result": [
{
"name": "Test1",
"start": "0%",
"end": "64.28571428571429%"
},
{
"name": "Test2",
"start": "14.285714285714285%",
"end": "85.71428571428571%"
},
{
"name": "Test3",
"start": "35.714285714285715%",
"end": "100%"
},
{
"name": "Test4",
"start": "71.42857142857143%",
"end": "92.85714285714286%"
}
]
}
根据您的评论,这里有一个不同的版本,可以满足您的要求!
const data = [
"Test1",
"Test1",
"Test2",
"Test2",
"Test2",
"Test3",
"Test3",
"Test3",
"Test3",
"Test1",
"Test4",
"Test4",
"Test2",
"Test4",
"Test3"
]
type Rage = { name: string, start: string, end: string };
function calcComponent(data: string[]) {
return data.reduce((output, value, index, array) => {
const lastItem = output[output.length - 1];
if(lastItem && lastItem.name === value) {
lastItem.end = (index / (data.length - 1) * 100) + "%";
} else {
output.push({
name: value,
start: (index / (data.length - 1) * 100) + "%",
end: (index / (data.length - 1) * 100) + "%"
})
}
return output;
}, [] as Rage[])
}
const result = calcComponent(data);
console.log({ result });
Output 将是:
{
"result": [
{
"name": "Test1",
"start": "0%",
"end": "7.142857142857142%"
},
{
"name": "Test2",
"start": "14.285714285714285%",
"end": "28.57142857142857%"
},
{
"name": "Test3",
"start": "35.714285714285715%",
"end": "57.14285714285714%"
},
{
"name": "Test1",
"start": "64.28571428571429%",
"end": "64.28571428571429%"
},
{
"name": "Test4",
"start": "71.42857142857143%",
"end": "78.57142857142857%"
},
{
"name": "Test2",
"start": "85.71428571428571%",
"end": "85.71428571428571%"
},
{
"name": "Test4",
"start": "92.85714285714286%",
"end": "92.85714285714286%"
},
{
"name": "Test3",
"start": "100%",
"end": "100%"
}
]
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.