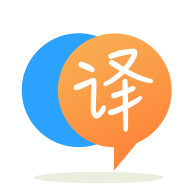
[英]General error: 1005 Can't create table (errno: 150 "Foreign key constraint is incorrectly formed")
[英]General error : 1005 Can't create table ... (errno:150 "Foreign key constraint is incorrectly formed"
我必须在我的在线项目上运行迁移,但它不适用于外键。 我有两个表:media 和 video_categorie(现有) 这是我创建 video_categorie 文件的迁移文件:
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreateVideoCategorieTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('video_categorie', function (Blueprint $table) {
$table->increments('id');
$table->string('nom_fr', 50);
$table->string('nom_en', 50)->nullable();
$table->unsignedSmallInteger('ordre')->nullable();
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('video_categorie');
}
}
另一个在我的媒体表上创建外键:
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class AddForeignKeyToMediaTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::table('media', function (Blueprint $table) {
$table->unsignedInteger('video_categorie_id')->nullable();
$table->unsignedSmallInteger('ordre_video')->nullable();
$table->foreign('video_categorie_id')->references('id')->on('video_categorie');
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::table('media', function (Blueprint $table) {
$table->dropForeign('media_video_categorie_id_foreign');
$table->dropColumn('video_categorie_id');
$table->dropColumn('ordre_video');
});
}
}
当我在本地服务器上尝试时,它就像一个魅力,我的数据库按我想要的方式更新。 但是在我的 web 主机上,当我尝试运行时出现此错误
php artisan migrate
Migrating: 2022_09_15_092133_create_video_categorie_table
Migrated: 2022_09_15_092133_create_video_categorie_table (7.91ms)
Migrating: 2022_09_15_115815_add_foreign_key_to_media_table
Illuminate\Database\QueryException
SQLSTATE[HY000]: General error: 1005 Can't create table `stag_db`.`media` (errno: 150 "Foreign key constraint is incorrectly formed") (SQL: alter table `media` add constraint `media_video_categorie_id_foreign` foreign key (`video_categorie_id`) references `video_categorie` (`id`))
at vendor/laravel/framework/src/Illuminate/Database/Connection.php:712
708▕ // If an exception occurs when attempting to run a query, we'll format the error
709▕ // message to include the bindings with SQL, which will make this exception a
710▕ // lot more helpful to the developer instead of just the database's errors.
711▕ catch (Exception $e) {
➜ 712▕ throw new QueryException(
713▕ $query, $this->prepareBindings($bindings), $e
714▕ );
715▕ }
716▕ }
+9 vendor frames
10 database/migrations/2022_09_15_115815_add_foreign_key_to_media_table.php:20
Illuminate\Support\Facades\Facade::__callStatic("table")
+22 vendor frames
33 artisan:37
Illuminate\Foundation\Console\Kernel::handle(Object(Symfony\Component\Console\Input\ArgvInput), Object(Symfony\Component\Console\Output\ConsoleOutput))
有谁知道这是从哪里来的?
我检查了我的本地和我的主机 web 版本的 php 和 mysql,它们并不相同。
本地 php:PHP 7.4.3
托管 php:PHP 7.3.33
本地 mysql:mysql 版本 8.0.30
托管 mysql:mysql Ver 15.1 Distrib 10.3.36-MariaDB
这些差异有问题吗?
谢谢!
编辑
上次迁移(php artisan migrate:status):
| Yes | 2022_08_18_135729_add_fichier_column_to_contact_table | 32 |
| Yes | 2022_08_29_120103_add_contact_motif_name_column_to_contact_table | 33 |
| Yes | 2022_09_15_092133_create_video_categorie_table | 33 |
| No | 2022_09_15_115815_add_foreign_key_to_media_table | |
| No | 2022_09_15_120150_add_orph_video_column_to_media_table | |
+------+------------------------------------------------------------------------------------------------------+-------+
编辑
几个小时后,我终于找到了问题所在; 如果它可以帮助其他人;)
在我的虚拟主机上,使用的默认存储引擎是 MyISAM,所以我的新表 video_categorie 是用这个引擎创建的。
但是这个引擎不允许在表之间建立关系。
我创建表 video_categorie 的第一次迁移有效,但是当我尝试进行第二次迁移以建立外键时,由于 video_categorie 引擎,它不起作用。
我联系我的虚拟主机以将我的服务器 mysql 配置更改为默认引擎 InnoDB。
在等待他们回答我时,我刚刚运行了我的第一次迁移,然后手动更改表引擎(ALTER TABLE video_categorie ENGINE = InnoDB)。
最后我运行了第二次迁移,它工作了!!!
该错误通知您media
上的主键和video_categorie
上的外键的列类型不匹配。
我的建议是更改您的迁移并使用列定义助手。
创建视频类别表
Schema::create('video_categorie', function (Blueprint $table) {
$table->id('id'); // using the id column type
$table->string('nom_fr', 50);
$table->string('nom_en', 50)->nullable();
$table->unsignedSmallInteger('ordre')->nullable();
$table->timestamps();
});
AddForeignKeyToMediaTable
Schema::table('media', function (Blueprint $table) {
$table->foreignId('video_categorie_id')->nullable();
$table->unsignedSmallInteger('ordre_video')->nullable();
$table->foreign('video_categorie_id')->references('id')->on('video_categorie');
});
更新
为清楚起见,问题在于列类型integer
和unsignedInteger
不兼容,因此您需要更改外键列类型以与主键列类型兼容。 上面显示了如何进行映射。
此修改需要在您的AddForeignKeyToMediaTable迁移中完成,替换$table->unsignedInteger('video_categorie_id')->nullable();
完全声明。 如果它仍然存在于任何地方的迁移中,您将继续看到错误。 您不能为此语句添加新的rollback
迁移,因为迁移失败,它从未执行过。
几个小时后,我终于找到了问题所在; 如果它可以帮助其他人;)
在我的虚拟主机上,使用的默认存储引擎是 MyISAM,所以我的新表 video_categorie 是用这个引擎创建的。
但是这个引擎不允许在表之间建立关系。
我创建表 video_categorie 的第一次迁移有效,但是当我尝试进行第二次迁移以建立外键时,由于 video_categorie 引擎,它不起作用。
我联系我的虚拟主机以将我的服务器 mysql 配置更改为默认引擎 InnoDB。
在等待他们回答我时,我刚刚运行了我的第一次迁移,然后手动更改表引擎(ALTER TABLE video_categorie ENGINE = InnoDB)。
最后我运行了第二次迁移,它工作了!!!
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.