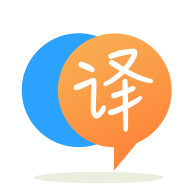
[英]How to check if a condition in foreach function matched in Javascript?
[英]How to reset forEach() function if statement is matched [NodeJS]
const downloadurl = ['url1', 'url2']
const filename = 'run.jar'
downloadurl.forEach((urls) => {
https.get(urls, (httpsres) => {
const path = `${installationdirectory}/${filename}`
const filePath = fs.createWriteStream(path);
httpsres.pipe(filePath);
filePath.on('finish',() => {
filePath.close();
const filesize = fs.statSync(path).size
// Verify if the size of the file is smaller than 1Kb
if((filesize / 1024) < 1) return;
// Want to skip this console.log if the file size is smaller than 1Kb and try with the other downloadurl (Hence why the downloadurl.forEach)
console.log('File downloaded!');
})
})
})
基本上,我使用的是来自 NodeJS 的 https 模块,如果下载 url 不存在,它不会给出错误,因为它只是创建一个 1 字节文件,而不是名称为run.jar
。 所以,如果文件的大小小于1Kb,我想让它停止执行代码并尝试与其他URL一起下载,有什么想法吗?
// Prints "1, 2, 3"
[1, 2, 3, 4, 5].every(v => {
if (v > 3) {
return false;
}
console.log(v);
// Make sure you return true. If you don't return a value, `every()` will stop.
return true;
});
const myNums = [1, 2, 3, 4, 5];
myNums.forEach((v, index, arr) => {
console.log(v);
if (val > 3) {
arr.length = index + 1; // Behaves like `break`
}
}
如果您现在使用回调结构,您可以尝试递归 function 以尝试进一步下载。
function tryDownload(downloadUrls):
// try download the first
https.get(downloadUrls[0], (res) => {
// read it, etc
// ...
// if too small, try the next urls
if ((filesize / 1024) < 1)
tryDownload(downloadUrls.slice(1));
else
// success!
// ...
})
不过,您可能会发现将其重组为异步 function 很清楚。 在伪代码中:
for each url in download urls
res = await download url
if res indicates success
handle the res
return
使用@Matt 的答案,因为它是最简单的实现(而且我不知道 HTTPS 模块确实有检查状态码的响应)。 谢谢所有回答的人^^
const downloadurl = ['url1', 'url2']
const filename = 'run.jar'
downloadurl.forEach((urls) => {
https.get(urls, (httpsres) => {
if(httpsres.statusCode !== 200) {
return console.log(`Attempt of downloading failed with ${httpsres.statusCode} error! Retrying with another download URL`);
} else {
const path = `${installationdirectory}/${filename}`
const filePath = fs.createWriteStream(path);
httpsres.pipe(filePath);
filePath.on('finish',() => {
filePath.close();
const filesize = fs.statSync(path).size
// Verify if the size of the file is smaller than 1Kb
if((filesize / 1024) < 1) return;
// Want to skip this console.log if the file size is smaller than 1Kb and try with the other downloadurl (Hence why the downloadurl.forEach)
console.log('File downloaded!');
})
}
})
})
```
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.