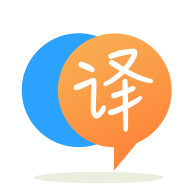
[英]How to load paths into ListBox and afterwards start the file per selected Index
[英]How to load image from disk according to selected index in listBox?
绘制完矩形后,我将图像以 bitmap 的形式保存到硬盘,将图像编号以及矩形位置和大小添加到列表框,最后我将创建一个包含该信息的文本文件。
private void pictureBox2_MouseUp(object sender, MouseEventArgs e)
{
if (e.Button != MouseButtons.Left) return;
if (DrawingRects.Count > 0)
{
// The last drawn shape
var dr = DrawingRects.Last();
if (dr.Rect.Width > 0 && dr.Rect.Height > 0)
{
rectImage = cropAtRect((Bitmap)pictureBox2.Image, dr.Rect);
if (saveRectangles)
{
rectangleName = @"d:\Rectangles\rectangle" + saveRectanglesCounter + ".bmp";
rectImage.Save(rectangleName);
saveRectanglesCounter++;
}
pixelsCounter = rect.Width * rect.Height;
pictureBox1.Invalidate();
// ListBox used to present the shape coordinates
listBox1.Items.Add($"{dr.Location}, {dr.Size}");
StreamWriter w = new StreamWriter(@"d:\Rectangles\rectangles.txt", true);
w.WriteLine(rectangleName.Substring(23) + " ===> " + $"{dr.Location}, {dr.Size}");
w.Close();
}
}
}
结果是:
左边是列表框,右边的项目是文本文件的内容和硬盘上的图像。
我想要做的是在绘制矩形并且应用程序正在运行时能够在列表框中的项目之间移动并在 pictureBox1 中显示保存的图像以及再次运行应用程序以读取文本文件内容并添加回来列表框中格式的项目回到列表框,然后再次能够在项目之间移动并显示图像。
不知道如何知道什么图像属于列表框中的什么项目,然后如何在选定的索引事件中加载它。
private void listBox1_SelectedIndexChanged(object sender, EventArgs e)
{
foreach (int x in listBox1.SelectedIndices)
{
}
}
完整代码
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Drawing.Drawing2D;
using System.Drawing.Imaging;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using static System.Net.Mime.MediaTypeNames;
using static System.Windows.Forms.VisualStyles.VisualStyleElement.ProgressBar;
namespace Image_Crop
{
public partial class Form1 : Form
{
System.Drawing.Image img = null;
Rectangle rect;
int pixelsCounter = 0;
Color SelectedColor = Color.LightGreen;
List<DrawingRectangle> DrawingRects = new List<DrawingRectangle>();
Bitmap rectImage;
int saveRectanglesCounter = 1;
bool drawBorder = true;
bool clearRectangles = true;
bool saveRectangles = true;
string rectangleName;
public Form1()
{
InitializeComponent();
pictureBox2.Image = System.Drawing.Image.FromFile(@"d:\Comparison\ConvertedBmp.bmp");
img = System.Drawing.Image.FromFile(@"d:\Comparison\radar_without_clouds.jpg");
checkBoxDrawBorder.Checked = true;
checkBoxClearRectangles.Checked = true;
checkBoxSaveRectangles.Checked = true;
}
public class DrawingRectangle
{
public Rectangle Rect => new Rectangle(Location, Size);
public Size Size { get; set; }
public Point Location { get; set; }
public Control Owner { get; set; }
public Point StartPosition { get; set; }
public Color DrawingcColor { get; set; } = Color.LightGreen;
public float PenSize { get; set; } = 3f;
}
private void Form1_Load(object sender, EventArgs e)
{
}
private void button1_Click(object sender, EventArgs e)
{
if (img == null)
{
// Create image.
img = System.Drawing.Image.FromFile(@"d:\Comparison\ConvertedBmp.bmp");
}
}
private void Form1_Paint(object sender, PaintEventArgs e)
{
}
private void pictureBox2_MouseDown(object sender, MouseEventArgs e)
{
if (e.Button != MouseButtons.Left) return;
DrawingRects.Add(new DrawingRectangle()
{
Location = e.Location,
Size = Size.Empty,
StartPosition = e.Location,
Owner = (Control)sender,
DrawingcColor = SelectedColor // <= Shape's Border Color
});
}
private void pictureBox2_MouseMove(object sender, MouseEventArgs e)
{
if (e.Button != MouseButtons.Left) return;
var dr = DrawingRects[DrawingRects.Count - 1];
if (e.Y < dr.StartPosition.Y) { dr.Location = new Point(dr.Rect.Location.X, e.Y); }
if (e.X < dr.StartPosition.X) { dr.Location = new Point(e.X, dr.Rect.Location.Y); }
dr.Size = new Size(Math.Abs(dr.StartPosition.X - e.X), Math.Abs(dr.StartPosition.Y - e.Y));
pictureBox2.Invalidate();
}
private void pictureBox2_MouseUp(object sender, MouseEventArgs e)
{
if (e.Button != MouseButtons.Left) return;
if (DrawingRects.Count > 0)
{
// The last drawn shape
var dr = DrawingRects.Last();
if (dr.Rect.Width > 0 && dr.Rect.Height > 0)
{
rectImage = cropAtRect((Bitmap)pictureBox2.Image, dr.Rect);
if (saveRectangles)
{
rectangleName = @"d:\Rectangles\rectangle" + saveRectanglesCounter + ".bmp";
rectImage.Save(rectangleName);
saveRectanglesCounter++;
}
pixelsCounter = rect.Width * rect.Height;
pictureBox1.Invalidate();
// ListBox used to present the shape coordinates
listBox1.Items.Add($"{dr.Location}, {dr.Size}");
StreamWriter w = new StreamWriter(@"d:\Rectangles\rectangles.txt", true);
w.WriteLine(rectangleName.Substring(23) + " ===> " + $"{dr.Location}, {dr.Size}");
w.Close();
}
}
}
private void pictureBox2_Paint(object sender, PaintEventArgs e)
{
DrawShapes(e.Graphics);
}
private void pictureBox1_Paint(object sender, PaintEventArgs e)
{
if (drawBorder)
{
ControlPaint.DrawBorder(e.Graphics, pictureBox1.ClientRectangle, Color.Red, ButtonBorderStyle.Solid);
}
if (rectImage != null && DrawingRects.Count > 0)
{
var dr = DrawingRects.Last();
e.Graphics.DrawImage(rectImage, dr.Rect);
if (clearRectangles)
{
DrawingRects.Clear();
pictureBox2.Invalidate();
}
}
}
private void DrawShapes(Graphics g)
{
if (DrawingRects.Count == 0) return;
g.SmoothingMode = SmoothingMode.AntiAlias;
foreach (var dr in DrawingRects)
{
using (Pen pen = new Pen(dr.DrawingcColor, dr.PenSize))
{
g.DrawRectangle(pen, dr.Rect);
};
}
}
public Bitmap cropAtRect(Bitmap b, Rectangle r)
{
Bitmap nb = new Bitmap(r.Width, r.Height);
using (Graphics g = Graphics.FromImage(nb))
{
g.DrawImage(b, -r.X, -r.Y);
return nb;
}
}
private void checkBoxDrawBorder_CheckedChanged(object sender, EventArgs e)
{
if(checkBoxDrawBorder.Checked)
{
drawBorder = true;
}
else
{
drawBorder = false;
}
pictureBox1.Invalidate();
}
private void checkBoxClearRectangles_CheckedChanged(object sender, EventArgs e)
{
if(checkBoxClearRectangles.Checked)
{
clearRectangles = true;
}
else
{
clearRectangles = false;
}
pictureBox2.Invalidate();
}
private void checkBoxSaveRectangles_CheckedChanged(object sender, EventArgs e)
{
}
private void listBox1_SelectedIndexChanged(object sender, EventArgs e)
{
foreach (int x in listBox1.SelectedIndices)
{
}
}
}
}
抱歉,为了美观,我隐藏了部分代码,希望您能理解。 您可以使用Dictionary
来匹配名称和路径:
Dictionary<string, string> FileList = new Dictionary<string, string>();
private void pictureBox2_MouseUp(object sender, MouseEventArgs e)
{
// ...
if (saveRectangles)
{
// ...
FileList.Add($"{dr.Location}, {dr.Size}", rectangleName);
}
// ...
}
private void listBox1_SelectedIndexChanged(object sender, EventArgs e)
{
yourPictureBox.Image = Image.FromFile(FileList
.Where(x => x.Key == ((ListBox)sender).SelectedItem?.ToString())
.Select(x => x.Value).First()
);
}
如果以后需要保存这些密钥对,我觉得保存到JSON格式的配置文件中使用会比较方便。 为此,您需要连接Newtonsoft.Json package。对于此 package,文档位于此处。
下面是一个保存和加载路径的小例子:
// Path of demo config file
string Path = $@"{Environment.CurrentDirectory}\data.json";
Dictionary<string, string> PathList = new Dictionary<string, string>();
// Checking config file data.json in current directory
if(!File.Exists(Path))
{
// Loading demos data if config file not found
PathList.Add("path1", "value1");
PathList.Add("path2", "value2");
}
else
{
// If demo config file found - upload him
// by specifying the type Dictionary<string, string>
using (StreamReader sr = new StreamReader(Path))
PathList = JsonConvert.DeserializeObject<Dictionary<string, string>>(sr.ReadToEnd());
}
// Showing the config keys
ListBoxKeys.Items.AddRange(PathList.Select(x => x.Key).ToArray());
// Showing the config value by selected index
ListBoxKeys.SelectedIndexChanged += (s, e) =>
{
LabelValue.Text = PathList
.Where(x => x.Key == ((ListBox)s).SelectedItem?.ToString())
.Select(x => x.Value).First();
};
// Saving data list to demo config file
ButtonSave.Click += (s, e) =>
{
using (StreamWriter sw = new StreamWriter(Path))
sw.WriteLine(JsonConvert.SerializeObject(PathList, Formatting.Indented));
};
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.