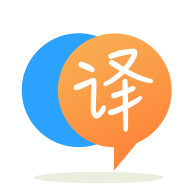
[英]How to return bool with message inside the Search Word method C#?
[英]How to return true or false with message inside the bool method for parallel array C#?
注:我正在学习使用arrays。
我的并行数组的 bool 方法的问题是我想返回 true 或 false,并显示余额为空(等于 double 数据类型中的 0.00)或不为空(返回 false)的消息。 运行这段代码后我得到了结果:
简而言之,一旦我运行这些代码,它就会循环或者不会读取用户的余额是否为空,因为它不会检查它是零还是小于零。 相反,他们只是忽略它然后返回一个错误。
这是我的代码:
// declaring three parallel arrays that store name, account number, and balance with data types
string [] customerName = new string [2];
int [] bankAccount = new int [2];
double [] balance = new double [2];
customerName = new string [] { "John Doe", "Jane Doe", "John Smith" };
bankAccount = new int [] { 123456 , 654321 , 987654 };
balance = new double [] {100.00, 200.00, 300.00};
for ( int i = 0; i < customerName.Length; i++)
{
Console.WriteLine(customerName[i] + "\t" + "Account Number: " + bankAccount[i] + "\t" + "Balance: $" + balance[i]);
}
// declared three different balances for the deposit method since context is necessary for local variables
double balance1 = balance[0];
double balance2 = balance[1];
double balance3 = balance[2];
Console.WriteLine(isEmpty(balance1));
Console.WriteLine(isEmpty(balance2));
Console.WriteLine(isEmpty(balance3));
bool isEmpty (double balance)
{
bool balance1 = balance == 0;
bool balance2 = balance == 0;
bool balance3 = balance == 0;
if (balance == 0)
{
return true;
}
else
{
Console.WriteLine("The balance is not empty");
}
return balance1 || balance2 || balance3;
}
// hardcoded values for three customers' balances to be updated
double deposit = 200.00;
double deposit1 = 300.00;
double deposit2 = 400.00;
// declared three different customers' names for return with string data type
string firstCustomer = "John Doe";
string secondCustomer = "Jane Doe";
string thirdCustomer = "John Smith";
Console.WriteLine(Deposit(firstCustomer, balance1, deposit));
Console.WriteLine(Deposit(secondCustomer, balance2, deposit1));
Console.WriteLine(Deposit(thirdCustomer, balance3, deposit2));
// string return that will return the three customers with updated balance after the deposit which is string
string Deposit (string customerName, double balance, double deposit)
{
double newBalance = balance + deposit;
return customerName + " deposited $" + deposit + " and the new balance is " + newBalance;
}
因此,我需要简单地检查并在确定余额是否为空后返回 false 或 true。 没有错误,只是显示一些重复并忽略返回 false 的任何错误。 相反,无论它是什么,它们都将始终返回 true。
在我看来,如果你用一个double
调用IsEmpty
,你只需要这个:
bool IsEmpty(double balance)
{
if (balance == 0)
{
return true;
}
else
{
Console.WriteLine("The balance is not empty");
return false;
}
}
如果您使用数组调用它:
bool IsEmpty(params double[] balances)
{
if (balances.Any(b => b == 0))
{
return true;
}
else
{
Console.WriteLine("No balance is empty");
return false;
}
}
params
关键字允许您将数组作为单独的变量传递,例如IsEmpty(balance1, balance2, balance3)
。
请注意,我将方法的名称从isEmpty
更改为IsEmpty
以遵循标准的 C# 命名约定。
您还最好创建一个数组并使用自定义类型:
Account[] accounts = new Account[]
{
new Account() { Customer = "John Doe", Number = 123456, Balance = 100m, },
new Account() { Customer = "Jane Doe", Number = 654321, Balance = 200m, },
new Account() { Customer = "John Smith", Number = 987654, Balance = 300m, },
};
public class Account
{
public string Customer { get; set; }
public int Number { get; set; }
public decimal Balance { get; set; }
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.