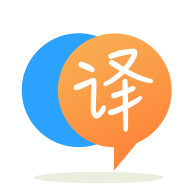
[英]How to parse, read, and store only one column of .CSV file into an array in C++
[英]How to read only one column from a csv file in c++?
这里我有一个 excel 文件,其第一列有 ID,即:ID 12 32 45 12.. 还有其他列,但我只想读取第一列中存在的数据,即 ID。
这是我抛出异常的代码。 我不知道为什么?
#include <iostream>
#include <string>
#include <fstream>
#include <sstream>
#include <vector>
#include <algorithm>
#include<string>
#include<cstdlib>
//std::find
#include<cstring>
using namespace std;
int main()
{
ifstream fin("1.csv");
string line;
int rowCount = 0;
int rowIdx = 0; //keep track of inserted rows
//count the total nb of lines in your file
while (getline(fin, line)) {
rowCount++;
}
//this will be your table. A row is represented by data[row_number].
//If you want to access the name of the column #47, you would
//cout << data[0][46]. 0 being the first row(assuming headers)
//and 46 is the 47 column.
//But first you have to input the data. See below.
std::vector<std::vector<std::string>> data;
fin.clear(); //remove failbit (ie: continue using fin.)
fin.seekg(fin.beg); //rewind stream to start
while (getline(fin, line)) //for every line in input file
{
stringstream ss(line); //copy line to stringstream
string value;
while (getline(ss, value, ',')) { //for every value in that stream (ie: every cell on that row)
data[rowIdx].push_back(value);//add that value at the end of the current row in our table
}
rowIdx++; //increment row number before reading in next line
}
fin.close();
//Now you can choose to access the data however you like.
//If you want to printout only column 47...
int colNum;
string colName = "ID";
//1.Find the index of column name "computer science" on the first row, using iterator
//note: if "it == data[0].end()", it means that that column name was not found
vector<string>::iterator it = find(data[0].begin(), data[0].end(), colName);
//calulate its index (ie: column number integer)
colNum = std::distance(data[0].begin(), it);
//2. Print the column with the header "computer science"
for (int row = 0; row < rowCount; row++)
{
cout << data[row][colNum] << "\t"; //print every value in column 47 only
}
cout << endl;
return 0;
}
请帮我解决这个问题。 我只想显示包含 ID 的第一列。
这是上述代码的简化版本。 它摆脱了 2D 向量,只读取第一列。
std::vector<std::string> data;
ifstream fin("1.csv");
string line;
while (getline(fin, line)) //for every line in input file
{
stringstream ss(line); //copy line to stringstream
string value;
if (getline(ss, value, ',')) {
data.push_back(value);
}
}
编辑
如何显示数据
for (size_t i = 0; i < data.size(); ++i)
cout << data[i] << '\n';
问题:
std::vector<std::vector<std::string>> data;
分配vector
s 的vector
。 这两个维度当前都设置为 0。
data[rowIdx].push_back(value);
推入并可能调整内部vector
的大小,但外部向量的大小保持为rowIdx
的值无效。 天真的解决方案是使用rowCount
来确定外部vector
的大小,但事实证明这是一种浪费。 您可以组装整行,然后将行push_back
到data
中,但即使这样也是一种浪费,因为只需要一列。
vector 的优点之一是您不必知道行数。 您制作一个行向量,将列推入其中,然后将行推入数据。 当您到达文件末尾时,您就完成了阅读。 无需两次读取文件,但您可能会在数据上次自我调整大小时浪费一些存储空间。
#include <iostream>
#include <string>
#include <fstream>
#include <sstream>
#include <vector>
// reduced includes to minimum needed for this example
// removed using namespace std; to reduce odds of a naming collision
int main() {
std::ifstream fin("1.csv"); // Relative path, so watch out for problems
// with working directory
std::string line;
std::vector<std::string> data; // only need one column? Only need one
// dimension
if (fin.is_open())
{
while (std::getline(fin, line)) //for every line in input file
{
std::stringstream ss(line);
std::string value;
if (std::getline(ss, value, ','))
{
// only take the first column If you need, say, the third
// column read and discard the first two columns
// and store the third
data.push_back(value); // vector sizes itself with push_back,
// so there is no need to count the rows
}
}
}
else
{
std::cerr << "Cannot open file\n";
return -1;
}
// use the column of data here
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.