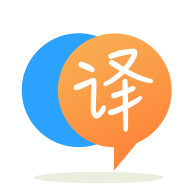
[英]Rest API response as XML format, calling from Spring Boot with Resttemplate
[英]How to extract data from JSON response coming from external REST API using RestTemplate in Spring Boot?
我正在使用 RestTemplate 开发 spring 引导应用程序以使用外部 API。
下面是我为从外部 API 获得响应而编写的服务 class:
public CustomOlapReports getReportListByClientId(String clientId) {
ResponseEntity<CustomOlapReports> responseEntity = null;
CustomOlapReports jsonResponse = null;
try {
final String uri = "https://chapi.cloudhealthtech.com/olap_reports/custom?client_api_id="+clientId;
RestTemplate restTemplate = new RestTemplate();
HttpHeaders header = new HttpHeaders();
header.set(HttpHeaders.AUTHORIZATION, "Bearer "+apiKey);
header.set(HttpHeaders.ACCEPT, "application/json");
HttpEntity<String> requestEntity = new HttpEntity<String>("body",header);
responseEntity = restTemplate.exchange(uri, HttpMethod.GET, requestEntity, CustomOlapReports.class);
jsonResponse = responseEntity.getBody();
} catch (Exception e) {
e.printStackTrace();
}
return jsonResponse;
}
以下是我使用 exchange() 方法得到的响应:
{
"responseMessage": "SUCCESS",
"status": "OK",
"statusCode": 1,
"response": {
"links": {
"RCDSO - Production Environment Cost": {
"href": "href 1"
},
"RCDSO - Development & UAT Environment Cost": {
"href": "href 2"
},
"RCDSO - Total Cost for Prod - Compugen Managed": {
"href": "href 3"
},
"RCDSO - Virtual Machine Cost": {
"href": "href 4"
},
"RCDSO - Azure File Storage Cost": {
"href": "href 5"
},
"RCDSO - Azure Backup and Site Recovery": {
"href": "href 6"
},
"RCDSO - Azure App Services Cost": {
"href": "href 7"
}
}
}
}
/********************** CustomOlapReports.java *************************/
@Getter
@Setter
public class CustomOlapReports {
@JsonProperty("links")
private Links links;
}
/********************** Links.java *************************/
@Setter
@Getter
public class Links {
@JsonProperty("RCDSO - Production Environment Cost")
private RCDSOProductionEnvironmentCost rCDSOProductionEnvironmentCost;
@JsonProperty("RCDSO - Development & UAT Environment Cost")
private RCDSODevelopmentUATEnvironmentCost rCDSODevelopmentUATEnvironmentCost;
@JsonProperty("RCDSO - Total Cost for Prod - Compugen Managed")
private RCDSOTotalCostForProdCompugenManaged rCDSOTotalCostForProdCompugenManaged;
@JsonProperty("RCDSO - Virtual Machine Cost")
private RCDSOVirtualMachineCost rCDSOVirtualMachineCost;
@JsonProperty("RCDSO - Azure File Storage Cost")
private RCDSOAzureFileStorageCost rCDSOAzureFileStorageCost;
@JsonProperty("RCDSO - Azure Backup and Site Recovery")
private RCDSOAzureBackupAndSiteRecovery rCDSOAzureBackupAndSiteRecovery;
@JsonProperty("RCDSO - Azure App Services Cost")
private RCDSOAzureAppServicesCost rCDSOAzureAppServicesCost;
}
/********************** RCDSOProductionEnvironmentCost.java *************************/
@Getter
@Setter
public class RCDSOProductionEnvironmentCost {
@JsonProperty("href")
private String href;
}
/********************** RCDSODevelopmentUATEnvironmentCost.java *************************/
@Getter
@Setter
public class RCDSODevelopmentUATEnvironmentCost {
@JsonProperty("href")
private String href;
}
/********************** RCDSOTotalCostForProdCompugenManaged.java *************************/
@Getter
@Setter
public class RCDSOTotalCostForProdCompugenManaged {
@JsonProperty("href")
private String href;
}
/********************** RCDSOVirtualMachineCost.java *************************/
@Getter
@Setter
public class RCDSOVirtualMachineCost {
@JsonProperty("href")
private String href;
}
/********************** RCDSOAzureFileStorageCost.java *************************/
@Getter
@Setter
public class RCDSOAzureFileStorageCost {
@JsonProperty("href")
private String href;
}
/********************** RCDSOAzureBackupAndSiteRecovery.java *************************/
@Getter
@Setter
public class RCDSOAzureBackupAndSiteRecovery {
@JsonProperty("href")
private String href;
}
/********************** RCDSOAzureAppServicesCost.java *************************/
@Getter
@Setter
public class RCDSOAzureAppServicesCost {
@JsonProperty("href")
private String href;
}
所以现在,在我的应用程序中向客户端发送响应之前。 我想从此响应中获取数据并想进行一些操作并将其转换为自定义 POJO class。我尝试使用 JSONObject 但出现了一些异常。
我想使用 AJAX 在 HTML 表中显示这个 JSON 数据。
那么我应该如何从上面的 JSON 中提取或获取个人数据呢?
基于 JSON 结构,假设您有以下 POJO:
public class Report implements Serializable {
private Map<String, Link> links;
// getters, setters
}
public class Link {
private String href;
// getters, setters
}
现在不是将响应主体提取为 JSON String
,而是直接将其转换为 POJO。
public Report getReportListByClientId(String clientId) {
ResponseEntity<Report> responseEntity = null;
try {
..
responseEntity = restTemplate.exchange(uri, HttpMethod.GET, requestEntity, Report.class);
return responseEntity.getBody();
} catch (Exception e) {
..
}
}
假设:该项目在类路径中有Jackson
,因此默认情况下将启用MappingJacksonHttpMessageConverter
和RestTemplate
以使上述方法起作用。
我会尽力帮助你。 您可以使用 JsonPath 来操作您的字符串 json。
<dependency>
<groupId>com.jayway.jsonpath</groupId>
<artifactId>json-path</artifactId>
<version>2.7.0</version>
</dependency>
将此库添加到您的代码中,然后尝试使用它
String json = """
{
"links": {
"RCDSO - Production Environment Cost": {
"href": "href 1"
},
"RCDSO - Development & UAT Environment Cost": {
"href": "href 2"
},
"RCDSO - Total Cost for Prod - Compugen Managed": {
"href": "href 3"
},
"RCDSO - Virtual Machine Cost": {
"href": "href 4"
},
"RCDSO - Azure File Storage Cost": {
"href": "href 5"
},
"RCDSO - Azure Backup and Site Recovery": {
"href": "href 6"
},
"RCDSO - Azure App Services Cost": {
"href": "href 7"
}
}
}
""";
Map<String, Map<String, String>> links = JsonPath.read(json, "$.links");
links.forEach((key, value) -> {
System.out.println("k = " + key);
System.out.print("v = ");
value.forEach((key2, value2) -> {
System.out.println("key = " + key2 + " : value = " + value2);
});
});
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.