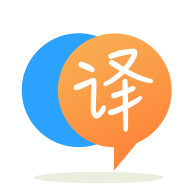
[英]Flutter Firestore : Add a List of Data to Firestore Document
[英]How to Read And view Firestore Document data using Flutter Forms
我需要使用 flutter 表格读取 Cloud firestore 文档数据
我尝试使用以下代码,但出现此错误 Expected a value of type 'DocumentSnapshot<Object?>', but got one of type 'String'
我正在使用以下代码将 dta 发送到 firestore 并发送到 accountnumber(docid) 阅读页面
import 'package:datetime_picker_formfield/datetime_picker_formfield.dart';
import 'package:kavinie/main.dart';
import 'package:kavinie/model/user.dart';
import 'package:flutter/material.dart';
import 'package:intl/intl.dart';
import 'package:kavinie/other/createbill.dart';
import 'package:kavinie/other/testnew.dart';
//import 'package:kavinie/other/home.dart';
import 'createdbill.dart';
class ReadAdd extends StatefulWidget {
@override
State<ReadAdd> createState() => _ReadAddState();
}
class _ReadAddState extends State<ReadAdd> {
final formKey = GlobalKey<FormState>();
late TextEditingController _controllerAccountnumber;
late TextEditingController _controlleryear;
late TextEditingController _controllerlastmonth;
late TextEditingController _controllermonth ;
var AccountNumber;
var Year;
var Month;
@override
void initState() {
super.initState();
_controllerAccountnumber = TextEditingController();
_controlleryear = TextEditingController();
_controllerlastmonth = TextEditingController();
_controllermonth = TextEditingController();
AccountNumber = _controllerAccountnumber.text;
Year = _controlleryear.text;
Month = _controllermonth.text;
}
@override
void dispose() {
_controllerAccountnumber.dispose();
_controlleryear.dispose();
_controllerlastmonth.dispose();
_controllermonth.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) => Scaffold(
appBar: AppBar(
title: Text('Create New Bill'),
backgroundColor: Colors.pink,
),
body: Form(
key: formKey,
child: ListView(
padding: EdgeInsets.all(16),
children: <Widget>[
TextFormField(
controller: _controllerAccountnumber,
decoration: decoration('Account Number'),
validator: (text) =>
text != null && text.isEmpty ? 'Not valid input' : null,
),
const SizedBox(height: 24),
TextFormField(
controller: _controlleryear,
decoration: decoration('Year'),
validator: (text) =>
text != null && text.isEmpty ? 'Not valid input' : null,
),
const SizedBox(height: 24),
TextFormField(
controller: _controllerlastmonth,
decoration: decoration('Last Month'),
validator: (text) =>
text != null && text.isEmpty ? 'Not valid input' : null,
),
const SizedBox(height: 24),
const SizedBox(height: 24),
TextFormField(
controller: _controllermonth,
decoration: decoration(' Month'),
validator: (text) =>
text != null && text.isEmpty ? 'Not valid input' : null,
),
const SizedBox(height: 24),
const SizedBox(height: 24),
const SizedBox(height: 32),
ElevatedButton(
child: Text('Create'),
onPressed: () {
final isValid = formKey.currentState!.validate();
if (isValid) {
final read = Read(
AccountNumber: _controllerAccountnumber.text,
Month: _controllermonth.text,
);
createUser(read);
final snackBar = SnackBar(
backgroundColor: Colors.green,
content: Text(
'Create New Bill ${_controllerAccountnumber.text} Sucessfully!',
style: TextStyle(fontSize: 24),
),
);
ScaffoldMessenger.of(context).showSnackBar(snackBar);
Navigator.pop(context);
Navigator.push(
context,
MaterialPageRoute(
builder: (_) =>editnote( docid: AccountNumber, year:Year, month:Month)
),
);
}
},
),
],
),
),
);
InputDecoration decoration(String label) => InputDecoration(
labelText: label,
border: OutlineInputBorder(),
);
Future createUser(Read read) async {
final docUser = FirebaseFirestore.instance.collection('customer').doc(_controllerAccountnumber.text).collection(_controlleryear.text).doc(_controllermonth.text);
//Read.id = docUser.id;
final json = read.toJson();
await docUser.set(json);
}
}
import 'dart:async';
import 'package:cloud_firestore/cloud_firestore.dart';
import 'package:firebase_auth/firebase_auth.dart';
import 'package:firebase_database/firebase_database.dart';
import 'package:flutter/material.dart';
import 'package:kavinie/home_screen.dart';
//import 'home.dart';
import 'bill.dart';
class editnote extends StatefulWidget {
DocumentSnapshot docid;
var year;
var month;
editnote({required this.docid,
required this.year, required month
});
@override
_editnoteState createState() => _editnoteState(docid: docid, year:year, month:month);
}
class _editnoteState extends State<editnote> {
DocumentSnapshot docid;
CollectionReference users = FirebaseFirestore.instance.collection('customers');
String displayUnit= 'no signal';
String displayPrevious = 'no signal';
String displayName = 'no signal';
late StreamSubscription _esp32;
final databse = FirebaseDatabase.instance.ref();
final FirebaseAuth auth = FirebaseAuth.instance;
_editnoteState({required this.docid, required year, required month});
TextEditingController AccountNumber = TextEditingController();
TextEditingController CustomerName = TextEditingController();
TextEditingController LastRead = TextEditingController();
TextEditingController NewRead = TextEditingController();
TextEditingController Unit = TextEditingController();
void _activateListeners() async {
_esp32 = databse.child("Py1/User").onValue.listen((event) {
final data = Map<String, dynamic>.from(event.snapshot.value as dynamic);
final Object? Units = data['Units'];
final Object? Total = data['Total'];
final Object? Usage = data['Usage'];
final Object? Previous = data['Previous'];
final Object? Name = data['Name'];
setState(() {
displayUnit = ' $Units';
displayPrevious = '$Previous';
displayName='$Name';
});
});
}
@override
void initState() {
_activateListeners();
AccountNumber = TextEditingController(text: widget.docid.get('AccountNumber'));
//CustomerName = TextEditingController(text: widget.docid.get('CustomerName'));
//LastRead = TextEditingController(text: widget.docid.get('LastRead'));
//NewRead = TextEditingController(text: widget.docid.get('NewRead'));
super.initState();
}
@override
Widget build(BuildContext context) {
final Stream<QuerySnapshot> _usersStream =
FirebaseFirestore.instance.collection('users').snapshots();
final dataRef = databse.child('Py/Users');
return Scaffold(
appBar: AppBar(title:Text("EBMS"),
backgroundColor:Colors.pink,
actions: <Widget>[
//IconButton
IconButton(
icon: const Icon(Icons.undo),
tooltip: 'Back',
onPressed: () {
Navigator.pushReplacement(
context, MaterialPageRoute(builder: (_) => HomeExtend()));
},
),
IconButton(
icon: const Icon(Icons.delete),
tooltip: 'delete',
onPressed: () {
widget.docid.reference.delete().whenComplete(() {
Navigator.pushReplacement(
context, MaterialPageRoute(builder: (_) => HomeExtend()));
});
},
), //Ico
//IconButton
], //<Widget>[]
elevation: 50.0,
leading: IconButton(
icon: const Icon(Icons.menu),
tooltip: 'Menu Icon',
onPressed: () async {
// Navigator.push(context,
// MaterialPageRoute(builder: (context) => TestHome()));
},
),
),
body: SingleChildScrollView(
child: Container(
child: Column(
children: [
Column(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Container(
width: 199,
height: 185,
// child: Image(image: AssetImage('assets/images/img.png')),
),
],
),
SizedBox(
height: 25,
),
SizedBox(
height: 20,
),
Container(
padding: EdgeInsets.symmetric(horizontal: 30, vertical: 16),
color: Color(0xffd9d9d9),
//padding: const EdgeInsets.only(left: 131, right: 152, top: 21, bottom: 15, ),
child: Row(
//mainAxisSize: MainAxisSize.min,
mainAxisAlignment: MainAxisAlignment.center,
crossAxisAlignment: CrossAxisAlignment.center,
children: [
Text(
"New Read: " + displayUnit,
style: TextStyle(
letterSpacing: 1.0,
// default is 0.0
color: Colors.white,
fontSize: 16,
fontFamily: "Inter",
fontWeight: FontWeight.w700,
),
),
],
),
),
SizedBox(
height: 20,
),
Container(
decoration: BoxDecoration(border: Border.all()),
child: TextField(
controller: AccountNumber,
decoration: InputDecoration(
hintText: 'AccountNumber',
),
),
),
SizedBox(
height: 10,
),
Container(
decoration: BoxDecoration(border: Border.all()),
child: TextField(
controller: CustomerName,
maxLines: null,
keyboardType: TextInputType.number,
decoration: InputDecoration(
hintText: 'CustomerName',
),
),
),
SizedBox(
height: 10,
),
Container(
decoration: BoxDecoration(border: Border.all()),
child: TextField(
controller: LastRead,
maxLines: null,
keyboardType: TextInputType.number,
decoration: InputDecoration(
hintText: 'LastRead',
),
),
),
SizedBox(
height: 10,
),
SizedBox(
height: 20,
),
MaterialButton(
color: Color.fromARGB(255, 238, 37, 119),
onPressed: () {
widget.docid.reference.update({
'CustomerName': CustomerName.text,
'LastRead': LastRead.text,
'NewRead': displayUnit,
'Total': displayUnit,
});
Navigator.push(
context,
MaterialPageRoute(
builder: (_) => GenaratedBill(
docid: docid,
),
),
);
},
child: Text(
"Create Bill",
style: TextStyle(
fontSize: 20,
color: Color.fromARGB(255, 251, 251, 251),
),
),
),
],
),
),
),
);
}
}
我尝试使用上面的代码读取数据
您已将 docId 构造函数指定为 DocumentSnapShot,但在路由到下一页时传递的字符串值为 controller。
改进:
class editnote extends StatefulWidget {
//DocumentSnapshot docid;
String docid;
var year;
var month;
editnote({required this.docid,
required this.year, required month
});
@override
_editnoteState createState() => _editnoteState(docid: docid, year:year, month:month);
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.