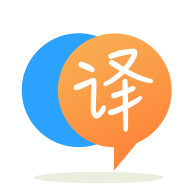
[英]how to give different text color for each item of listview in xamarin forms
[英]How to color each item in listView control in wpf in a different color?
首先我尝试了这个,但不确定在哪里以及如何实现它。
<ListView.Resources>
<ControlTemplate x:Key="SelectedTemplate" TargetType="ListViewItem">
<Border CornerRadius="5" BorderThickness="1" BorderBrush="DarkGray" Background="#FF92C6F9" Padding="2" HorizontalAlignment="Left" Margin="5" Tag="{Binding Value}" Cursor="Hand" MouseUp="Border_MouseUp_1">
<TextBlock Text="{Binding Name}" Margin="5" />
</Border>
</ControlTemplate>
<Style TargetType="ListViewItem">
<Setter Property="Template">
<Setter.Value>
<ControlTemplate TargetType="ListViewItem">
<Border CornerRadius="5" BorderThickness="1" BorderBrush="DarkGray" Background="WhiteSmoke" Padding="2" HorizontalAlignment="Left" Margin="5" Tag="{Binding Value}" Cursor="Hand" MouseUp="Border_MouseUp_1" >
<TextBlock Text="{Binding Name}" Margin="5" />
</Border>
</ControlTemplate>
</Setter.Value>
</Setter>
<Style.Triggers>
<MultiTrigger>
<MultiTrigger.Conditions>
<Condition Property="IsSelected" Value="true" />
<Condition Property="Selector.IsSelectionActive" Value="true" />
</MultiTrigger.Conditions>
<Setter Property="Template" Value="{StaticResource SelectedTemplate}" />
</MultiTrigger>
</Style.Triggers>
</Style>
</ListView.Resources>
我在列表视图名称之后或在网格部分内部尝试过,但出现了太多错误。
这是我的 xaml 代码
<Window x:Class="Lab_ListView.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:Lab_ListView"
mc:Ignorable="d"
Title="Video Game List" Height="450" Width="800">
<Grid Margin="0,0,10,10">
<Label Content="Game Name" HorizontalAlignment="Left" Margin="40,59,0,0" VerticalAlignment="Top"/>
<TextBox x:Name="GameNameTextBox" HorizontalAlignment="Left" Height="23" Margin="121,62,0,0" TextWrapping="Wrap" VerticalAlignment="Top" Width="366"/>
<Button x:Name="AddBtn" Content="Add" HorizontalAlignment="Left" Margin="121,151,0,0" VerticalAlignment="Top" Width="75" Click="AddBtn_Click"/>
<ListView x:Name="VideoGameListView" HorizontalAlignment="Left" Height="166" Margin="40,203,0,0" VerticalAlignment="Top" Width="447">
<ListView.View>
<GridView>
<GridViewColumn Header="Game Name" DisplayMemberBinding="{Binding GameName}"/>
<GridViewColumn Header="Rating" DisplayMemberBinding="{Binding Rating}"/>
<GridViewColumn Header="Price" DisplayMemberBinding="{Binding Price}"/>
</GridView>
</ListView.View>
</ListView>
<Label Content="Rating" HorizontalAlignment="Left" Margin="40,90,0,0" VerticalAlignment="Top"/>
<Label Content="Price" HorizontalAlignment="Left" Margin="40,121,0,0" VerticalAlignment="Top"/>
<TextBox x:Name="PriceTextBox" HorizontalAlignment="Left" Height="23" Margin="121,123,0,0" TextWrapping="Wrap" VerticalAlignment="Top" Width="366"/>
<TextBox x:Name="RatingTextBox" HorizontalAlignment="Left" Height="23" Margin="121,92,0,0" TextWrapping="Wrap" VerticalAlignment="Top" Width="366"/>
</Grid>
</Window>
这是 class Cropped_Info 的代码
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Lab_ListView
{
class Cropped_Info
{
private string m_GameName;
private string m_Rating;
private double m_Price;
public string GameName
{
get
{
return m_GameName;
}
set
{
m_GameName = value;
}
}
public string Rating
{
get
{
return m_Rating;
}
set
{
m_Rating = value;
}
}
public double Price
{
get
{
return m_Price;
}
set
{
m_Price = value;
}
}
public Cropped_Info()
{
GameName = " ";
Rating = " ";
Price = 0.0;
}
}
}
以及我将项目添加到 listView 的主要 xaml.cs 代码
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
namespace Lab_ListView
{
/// <summary>
/// Interaction logic for MainWindow.xaml
/// </summary>
public partial class MainWindow : Window
{
Cropped_Info vg = new Cropped_Info();
int counter = 0;
Random random;
List<Cropped_Info> list = new List<Cropped_Info>();
System.Windows.Threading.DispatcherTimer dispatcherTimer;
public MainWindow()
{
InitializeComponent();
random= new Random();
this.WindowStartupLocation = WindowStartupLocation.CenterScreen;
dispatcherTimer = new System.Windows.Threading.DispatcherTimer();
dispatcherTimer.Tick += DispatcherTimer_Tick;
dispatcherTimer.Interval = new TimeSpan(0, 0, 0);
dispatcherTimer.Start();
}
private void DispatcherTimer_Tick(object sender, EventArgs e)
{
vg = new Cropped_Info();
vg.GameName = randStr(5) + " " + counter.ToString();
VideoGameListView.Items.Add(vg);
if(counter == 1000)
{
dispatcherTimer.Stop();
for(int i = 0; i < VideoGameListView.Items.Count; i++)
{
var tt = VideoGameListView.Items[i];
}
}
counter++;
}
public string randStr(int len)
{
const string chars = "ABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789!@#$%^&*()";
return new string(Enumerable.Repeat(chars, len).Select(s => s[random.Next(s.Length)]).ToArray());
}
}
}
我希望添加到 listView 的每个字符串项目都可以是随机颜色,但主要目标是为每个项目着色。
您可以使用转换器来实现这一点。 例如,颜色的红色通道可能取决于价格。 价格越大,颜色越红,就像这样:
这是一个如何做到这一点的例子:
主窗口.xaml
<Window
x:Class="WpfListViewColorsDemo.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:local="clr-namespace:WpfListViewColorsDemo"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
Title="MainWindow"
Width="800"
Height="450"
mc:Ignorable="d">
<Window.Resources>
<local:PriceToBackgroundColorConverter x:Key="PriceToBackgroundColorConverter" />
</Window.Resources>
<Grid>
<ListView x:Name="ListViewItems">
<ListView.ItemTemplate>
<DataTemplate>
<!-- The Background="{Binding Price, Converter={StaticResource PriceToBackgroundColorConverter}}" is where Price gets converted into a Brush that can be set as the Background -->
<Border
Padding="5"
Background="{Binding Price, Converter={StaticResource PriceToBackgroundColorConverter}}"
BorderThickness="1"
CornerRadius="5">
<TextBlock Foreground="White">
<Run Text="{Binding Name}" />
<Run Text="{Binding Price, StringFormat=c}" />
</TextBlock>
</Border>
</DataTemplate>
</ListView.ItemTemplate>
</ListView>
</Grid>
</Window>
MainWindow.xaml.cs、Item.cs 和 PriceToBackgroundColorConverter.cs
using System;
using System.Collections.Generic;
using System.Globalization;
using System.Windows;
using System.Windows.Data;
using System.Windows.Media;
namespace WpfListViewColorsDemo;
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
ListViewItems.ItemsSource = new List<Item>
{
new Item()
{
Name = "Apple",
Price = 15.99
},
new Item()
{
Name = "Peach",
Price = 24.50
},
new Item()
{
Name = "Watermelon",
Price = 39.66
},
new Item()
{
Name = "Diamond",
Price = 70.42
},
new Item()
{
Name = "Unicorn",
Price = 99.00
}
};
}
}
public class Item
{
public string? Name { get; set; }
public double Price { get; set; }
}
public class PriceToBackgroundColorConverter : IValueConverter
{
public object Convert(object value, Type targetType, object parameter, CultureInfo culture)
{
if(value is double price)
{
return ConvertPriceToColor(price);
}
return value;
}
private Brush ConvertPriceToColor(double price)
{
// Add your custom coloring logic here.
// I'm simply making the color more red as the price goes up (capped at 100).
var red = Math.Clamp(price, 0, 100) * 255 / 100;
var color = Color.FromArgb(255, (byte)red, 0, b: 0);
return new SolidColorBrush(color);
}
public object ConvertBack(object value, Type targetType, object parameter, CultureInfo culture)
{
//do nothing here
return value;
}
}
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.