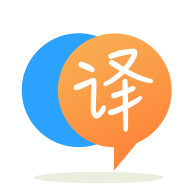
[英]Can't perform a React state update on an unmounted component. (Class component)
[英]How to update state of one component in another in react class component. Save Functionality
如何在反应 class 组件中更新另一个组件的 state。
我有两个 class 反应。
我的组件和我的容器。
export default class MyContainer extends BaseComponent{
constructor(props: any) {
super(props, {
status : false,
nameValue :"",
contentValue : ""
});
}
componentDidMount = () => {
console.log(this.state.status);
};
save = () => {
console.log("Hello I am Save");
let obj: object = {
nameValue: this.state.nameValue, // here I am getting empty string
templateValue: this.state.contentValue
};
// API Call
};
render() {
return (
<div>
<MyComponent
nameValue = {this.state.nameValue}
contentValue = {this.state.contentValue}
></MyComponent>
<div >
<button type="button" onClick={this.save} >Save</button>
</div>
</div>
);
}
}
我的组件
export default class MyComponent extends BaseComponent{
constructor(props: any) {
super(props, {});
this.state = {
nameValue : props.nameValue ? props.nameValue : "",
contentValue : props.contentValue ? props.contentValue : "",
status : false
}
}
componentDidMount = () => {
console.log("MOUNTING");
};
fieldChange = (id:String, value : String) =>{
if(id === "content"){
this.setState({nameValue:value});
}else{
this.setState({contentValue:value});
}
}
render() {
return (
<div>
<div className="form-group">
<input id="name" onChange={(e) => {this.fieldChange(e)}}></input>
<input id = "content" onChange={(e) => {this.fieldChange(e)}} ></input>
</div>
</div>
);
}
}
在 MyComponent 中,我在更改 state 的位置放置了两个输入字段。
我在 MyContainer 中的保存按钮。 在保存按钮中,我无法读取 MyComponent 的值。 实现这一目标的最佳方法是什么。
您应该在MyContainer
中更新您的 state 以便save
以查看 state 更改。 每个组件都有自己的 state,这使得MyComponent
的 state 与MyContainer
的唯一。 你应该做的是将 state 保留在你的父/容器组件中,然后将它作为道具传递下去(而不是在你的孩子中复制它)。 为此,将fieldChange
向上移动到MyContainer
function,并删除MyComponent
中重复的nameValue
和contentValue
state。 有关更多详细信息,请参见代码注释:
export default class MyContainer extends BaseComponent{
...
fieldChange = (id:String, value : String) =>{
if(id === "content"){
this.setState({nameValue: value});
} else {
this.setState({contentValue: value});
}
}
render() {
return (
<div>
<MyComponent
nameValue={this.state.nameValue}
contentValue={this.state.contentValue}
onFieldChange={this.fieldChange} /* <---- Pass the function down to `MyComponent` */
/>
...
</div>
);
}
}
然后在MyComponent
中调用this.props.onFieldChange
:
export default class MyComponent extends BaseComponent{
// !! this constructor can be removed as no state is being initialized anymore !!
constructor(props: any) {
super(props);
// removed state as we're using the state from `MyContainer`
}
componentDidMount = () => {
console.log("MOUNTING");
};
render() {
return (
<div>
<div className="form-group">
<input id="name" onChange={(e) => {this.props.fieldChange(e)}} /> /* <--- Change to `this.props.fieldChange()`. `<input />` is a self-closing tag.
<input id = "content" onChange={(e) => {this.props.fieldChange(e)}} />
</div>
</div>
);
}
}
一些附加说明:
this.props.children
,那么您应该将其称为<MyComponent... props... />
而不是<MyComponent... props...></MyComponent>
fieldChange
中的 if 语句看起来颠倒了,应该检查if(id === "name")
。 我假设这是你问题中的一个错误。fieldChange
。 我再次假设这是你问题中的一个错误。
声明:本站的技术帖子网页,遵循CC BY-SA 4.0协议,如果您需要转载,请注明本站网址或者原文地址。任何问题请咨询:yoyou2525@163.com.